When it comes to Node.js development, ensuring your application runs smoothly and efficiently is a top priority. One of the best ways to keep an eye on your app’s health, troubleshoot issues, and improve performance is through logging.
And if you're diving into logging in Node.js, Winston is one of the most popular and powerful libraries you can use.
In this guide, we’ll walk through everything you need to know about Winston logging, from its basic features to some advanced configurations.
What is Winston?
Winston is a flexible, multi-transport logging library built for Node.js. It’s designed to be simple yet powerful, allowing you to log messages in various formats and send them to different destinations, like the console, files, or even remote servers.
Whether you’re working on a small project or a large-scale application, Winston’s customizability makes it a perfect fit for any use case.
Why Use Winston for Logging in Node.js?
Logging is key for debugging, monitoring, and keeping your application running smoothly. So, why should you pick Winston for your logging needs?
Here are a few reasons why it stands out:
Multiple types of transport
Winston gives you the flexibility to log data to multiple places (called transports) — whether that’s to the console, a file, or even cloud storage. You can even create your own custom transports to send logs to remote servers if that’s what you need.
Log Levels
Winston supports different log levels like info
, debug
, warn
, and error
. This helps you organize and prioritize logs based on their importance and severity.
Custom Formats
Want your logs to look a certain way? Winston’s got you covered. It supports various log formats, such as JSON, plain text, or even custom formats that you can define. This means your logs can be tailored to exactly how you want them.
Easy Configuration
Configuring Winston is a breeze. You can set up log levels, formats, and transports with minimal fuss. It’s a simple process that saves you time and effort, especially when you need to adjust logging settings quickly.
Performance
Let’s face it—logging can sometimes slow down your app. But with Winston, that’s not a concern. It’s optimized for performance, so logging won’t create unnecessary overhead and will keep your app running smoothly.
How to Get Started with Winston in Your Node.js Project
Before talking about the configuration details, let's start with installing Winston in your Node.js project.
Step 1: Install Winston
To install Winston, you can use either npm or yarn:
npm install winston # or yarn add winston
Step 2: Basic Setup
Once you’ve installed Winston, you can start using it by requiring it in your application and configuring it for basic logging functionality.
const winston = require('winston');
const logger = winston.createLogger({
level: 'info', // Set the minimum logging level
transports: [
new winston.transports.Console(), // Log to the console
],
});
logger.info('This is an info log');
logger.error('This is an error log');
This will output logs to the console, with the log level set to info
. You can adjust the logging level to capture different types of logs based on your needs.
Advanced Configuration Options
Winston excels in customization. Here are some advanced options to tailor logging behavior for your project.
1. Adding Multiple Transports
You can log to multiple destinations at the same time, such as both the console and a log file:
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'logs/app.log' }),
],
});
With this setup, your logs will appear both in the console and the app.log
file, giving you more options for log analysis.
2. Customizing Log Formats
You can create structured logs by defining a custom format function. Winston provides a built-in format module to create logs in various formats, such as JSON or plain text.
const { printf, combine, timestamp, errors } = winston.format;
const customFormat = printf(({ timestamp, level, message, stack }) => {
return `${timestamp} [${level}]: ${stack || message}`;
});
const logger = winston.createLogger({
level: 'debug',
format: combine(
timestamp(),
errors({ stack: true }), // Capture stack traces for errors
customFormat
),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'logs/app.log' }),
],
});
logger.error(new Error('Something went wrong!'));
This setup logs timestamps and error stack traces in both the console and the log file.
3. Setting Log Levels
Log levels help categorize log importance. Winston has default log levels like info
, warn
, error
, debug
. You can customize the levels if needed. Log levels work hierarchically—setting the log level to info
captures info
, warn
, and error
logs, but debug
logs are ignored.
const logger = winston.createLogger({
level: 'warn', // Only log 'warn' and 'error'
transports: [
new winston.transports.Console(),
],
});
logger.info('This will not appear');
logger.warn('This is a warning');
logger.error('This is an error');
In this example, only warn
and error
logs will be displayed because the log level is set to warn
.
How to Centralize Winston Logs with Popular Services and Tools
As your application scales and your log data grows, managing logs across multiple servers and instances can become a challenge.
Centralizing log management helps you aggregate, search, analyze, and monitor your logs from a single location, making troubleshooting and performance monitoring much more efficient.
Here are some popular options for centralizing your Winston logs, including sending logs to cloud services or log management tools.
1. Cloud Log Management Services
Cloud-based logging services offer scalability, reliability, and powerful analytics features. These services can handle large volumes of logs, allow for fast searching, and provide powerful dashboards for monitoring.
AWS CloudWatch Logs
If you’re already using AWS for your infrastructure, CloudWatch Logs is a great choice for centralizing your logs. You can configure Winston to send logs to CloudWatch, where they can be stored, analyzed, and visualized.
Setup Example:
const winston = require('winston');
const CloudWatchTransport = require('winston-cloudwatch');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new CloudWatchTransport({
logGroupName: 'my-log-group',
logStreamName: 'my-log-stream',
awsRegion: 'us-east-1',
}),
],
});
logger.info('This log will be sent to AWS CloudWatch');
Google Cloud Logging
Google Cloud offers a similar service called Stackdriver Logging (now part of Google Cloud Operations). It integrates well with other Google Cloud services and can help with analyzing logs in real-time.
Setup Example:
const { Logging } = require('@google-cloud/logging');
const winston = require('winston');
const logging = new Logging();
const log = logging.log('my-log');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new winston.transports.Http({
host: 'logging.googleapis.com',
path: `/v2/${log.name}/entries:write`,
}),
],
});
logger.info('This log will be sent to Google Cloud Logging');
Azure Monitor
If your infrastructure runs on Microsoft Azure, you can use Azure Monitor to collect and analyze logs. Azure Monitor integrates with Application Insights to provide performance metrics and diagnostics from your applications.
Setup Example:
const winston = require('winston');
const AzureLog = require('winston-azure-logger');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new AzureLog({
instrumentationKey: 'your-instrumentation-key',
}),
],
});
logger.info('This log will be sent to Azure Monitor');
2. Log Management Tools
Log management tools provide a dedicated platform to ingest, analyze, and visualize logs. These tools usually come with advanced querying capabilities, alerting features, and integration options for other parts of your infrastructure.
Last9
Last9to is a high-cardinality observability platform designed to simplify observability by bringing together metrics, logs, and traces in one unified view.
It helps teams troubleshoot and monitor performance across distributed systems and microservices architectures.
If you're already using Winston for logging in to your Node.js application, you can easily integrate Last9 to centralize and monitor your logs from a single place.
Setup Example: Below is an example of how you can send logs to Last9 from your Node.js application using Winston:
const winston = require('winston');
const Last9Transport = require('winston-last9'); // Assuming a Last9 transport exists for Winston
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new Last9Transport({
apiKey: 'your-last9-api-key', // Replace with your Last9 API key
projectId: 'your-project-id', // Replace with your Last9 project ID
environment: 'production', // Optional: specify the environment (e.g., production, staging)
}),
],
});
logger.info('This log will be sent to Last9');
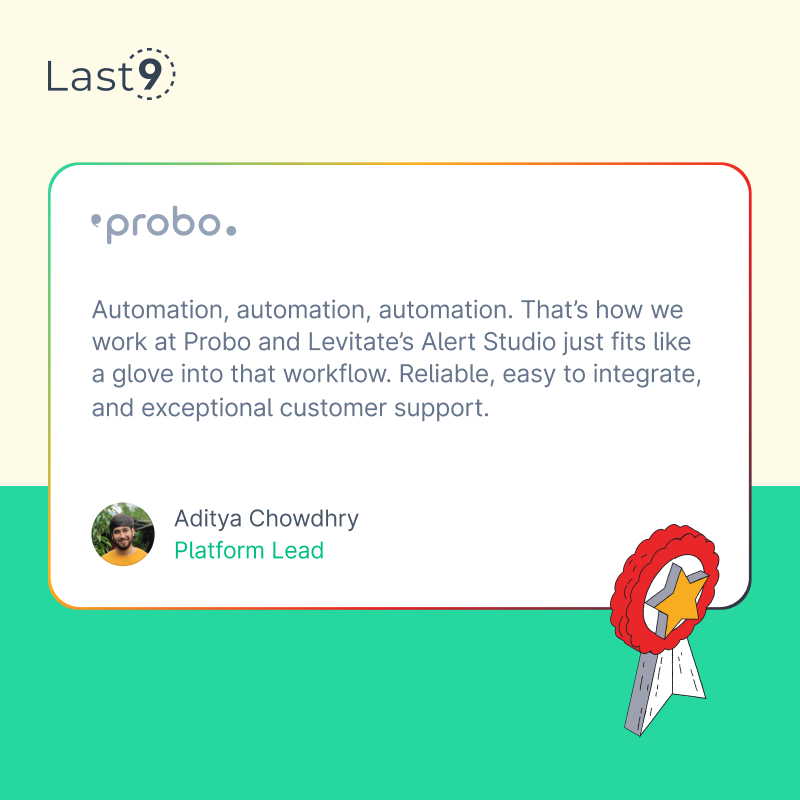
Papertrail
Papertrail is another cloud-based log management service that offers easy log aggregation and real-time log monitoring. It provides advanced filtering and search capabilities, making it easy to track down issues across distributed systems.
Setup Example:
const winston = require('winston');
const Papertrail = require('winston-papertrail').Papertrail;
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new Papertrail({
host: 'logs.papertrailapp.com',
port: 12345,
program: 'my-app',
}),
],
});
logger.info('This log will be sent to Papertrail');
This example assumes there's a custom transport winston-last9
for integrating Winston with Last9, similar to the Loggly transport in your original example. If such a transport doesn't exist, you could build one or use Last9's REST API to send logs via HTTP.
Make sure to replace the placeholders with your actual API key, project ID, and environment details for proper integration!
ELK Stack (Elasticsearch, Logstash, Kibana)
The ELK Stack is a powerful open-source solution for aggregating, indexing, and visualizing logs. You can send your Winston logs to Elasticsearch via Logstash, and then use Kibana for real-time analysis and dashboards.
Setup Example:
const winston = require('winston');
const Elasticsearch = require('winston-elasticsearch');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new Elasticsearch({
level: 'info',
clientOpts: {
node: 'http://localhost:9200',
},
}),
],
});
logger.info('This log will be sent to Elasticsearch');
3. Self-Hosted Solutions
If you prefer to manage your own log aggregation system, you can set up your own logging infrastructure using open-source tools like Fluentd or Logstash.
Fluentd
Fluentd is an open-source data collector for unified logging. It can be used to collect logs from multiple sources, transform the data, and send it to various destinations like Elasticsearch, Kafka, or databases.
Setup Example:
const winston = require('winston');
const Fluentd = require('fluent-logger').createFluentSender;
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new Fluentd('my-fluentd-host', 24224),
],
});
logger.info('This log will be sent to Fluentd');
Logstash
Logstash is a powerful tool for log aggregation, filtering, and forwarding. You can configure Logstash to receive logs from your application, process them, and then forward them to Elasticsearch or other log storage systems.
Setup Example: Sending logs to Logstash can be done by using TCP or UDP transports in combination with the winston-logstash package.
const winston = require('winston');
const Logstash = require('winston-logstash');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new Logstash({
host: 'logstash-server', // Replace with your Logstash server
port: 5044, // Default port for Logstash
}),
],
});
logger.info('This log will be sent to Logstash');
Make sure you have Logstash running and configured to listen on the specified port. This code will send Winston logs to Logstash, which can then forward them to other destinations like Elasticsearch for further processing.
Configuring Transports in Winston
Winston is a versatile logging library for Node.js, enabling you to configure multiple transports. These transports determine where your log messages are sent, such as the console, a file, or a remote log management system.
Configuring the right transports allows you to control how and where your logs are output, making monitoring and troubleshooting more efficient.
Let’s understand the different types of transports that you can configure in Winston.
1. Console Transport
This is commonly used for development and debugging. It allows log messages to be printed directly to the terminal. This transport also supports customizable formats and color coding, which makes it easy to distinguish between different log levels (e.g., errors in red).
Example Configuration:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info', // Default log level
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.colorize(), // Adds color to the logs
winston.format.simple() // Logs in a simple text format
)
})
],
});
logger.info('This is an info message.');
logger.error('This is an error message.');
In this example, log messages are printed with color depending on their level.
2. File Transport
For storing logs over time, the File transport is a great option. It helps in keeping persistent logs, especially for production environments. Winston supports rotating log files, which prevents logs from growing too large.
Example Configuration:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.File({ filename: 'logs/app.log' })
],
});
logger.info('This log will be written to a file.');
In this case, all logs are written to the app.log
file in the logs
directory.
3. File Transport with Log Rotation
In production environments, managing log file size is crucial. The winston-daily-rotate-file transport helps with log rotation. It rotates logs daily, weekly, or at any configurable interval.
Example Configuration:
const winston = require('winston');
require('winston-daily-rotate-file');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.DailyRotateFile({
filename: 'logs/application-%DATE%.log',
datePattern: 'YYYY-MM-DD',
maxSize: '20m', // Max file size before rotation
maxFiles: '14d', // Keep logs for 14 days
})
],
});
logger.info('This log will be written to a rotated file.');
In this example, log files are rotated daily, and logs older than 14 days are removed.
4. HTTP Transport
The HTTP transport sends logs to remote destinations, such as HTTP servers or centralized log management systems (e.g., Loggly, Papertrail). This transport is ideal for centralized logging and remote analysis.
Example Configuration:
const winston = require('winston');
const HttpTransport = require('winston-transport-http'); // Assuming you have this transport installed
const logger = winston.createLogger({
level: 'info',
transports: [
new HttpTransport({
host: 'your-log-server.com',
path: '/logs',
method: 'POST',
})
],
});
logger.info('This log will be sent to an HTTP server.');
This transport sends logs to a specified HTTP endpoint, making it easy to centralize and analyze logs remotely.
5. Custom Transports
Winston also allows you to create custom transports. You can extend the base TransportStreamOptions
class to send logs wherever you need them—whether to a database, a custom log server, or another destination.
Example Configuration:
const winston = require('winston');
class MyCustomTransport extends winston.transportStreamOptions {
log(info, callback) {
setImmediate(() => this.emit('logged', info));
console.log('Custom log output:', info.message);
callback();
}
}
const logger = winston.createLogger({
level: 'info',
transports: [
new MyCustomTransport(),
],
});
logger.info('This log uses a custom transport.');
In this example, the custom transport simply outputs logs to the console, but you can modify it to send logs to other destinations.
6. Log Levels in Transports
Winston allows you to configure specific log levels for each transport. For example, you may want to log everything to a file but only log warnings and errors to the console. This feature allows fine-grained control over your logging setup.
Example Configuration:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
level: 'warn', // Only log warnings and errors to the console
}),
new winston.transports.File({
filename: 'logs/app.log',
level: 'info', // Log all messages to the file
}),
],
});
logger.info('This log will be written to the file.');
logger.warn('This log will be written to both the file and console.');
logger.error('This log will be written to both the file and console.');
In this case, logs of level warn
and higher are shown in both the file and console, while info
logs appear only in the file.
Formatting Log Messages in Winston for Improved Readability and Structure
Winston gives you the flexibility to customize your log messages, whether you want to add timestamps, log levels, custom formats, colors, or even extra contextual details.
In this section, we'll take a look at how to format your log messages in Winston using various options to make them more structured and easy to read.
1. Basic Log Format
The simplest way to format log messages is by using the basic format, which outputs only the log message without any additional context. This can be helpful for quick debugging when you don’t need much information.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
format: winston.format.simple(), // Simple format: just log message
}),
],
});
logger.info('This is a simple log message');
Output:
This is a simple log message
2. Adding Timestamps
Adding timestamps to your logs is pretty common, as it helps you track when each event happened. Winston has a built-in timestamp
format that automatically adds a timestamp to each log message.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.timestamp(),
winston.format.simple() // Combine timestamp with simple log message
),
}),
],
});
logger.info('This log includes a timestamp');
Output:
2025-01-02T10:00:00.000Z This log includes a timestamp
3. Colorizing Logs
Colorizing logs is especially useful in a console environment to make different log levels (like info, warn, and error) stand out. Winston has a colorize
format that colorizes the log level, helping you quickly identify the severity of each log.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.colorize(), // Adds color to log levels
winston.format.simple()
),
}),
],
});
logger.info('This log is colorized');
logger.warn('This is a warning message');
logger.error('An error has occurred');
Output:
info: This log is colorized
warn: This is a warning message
error: An error has occurred
4. Custom Log Formats
Winston allows you to define your own custom formats by combining different formatting methods. The combine
method lets you stack multiple formats, such as adding timestamps, colors, and custom message structures.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.colorize(),
winston.format.timestamp(), // Add timestamp to each log
winston.format.printf(({ timestamp, level, message }) => {
return `${timestamp} [${level}]: ${message}`; // Custom log format
})
),
}),
],
});
logger.info('This is a custom formatted log');
Output:
2025-01-02T10:00:00.000Z [info]: This is a custom formatted log
5. JSON Log Format
JSON formatting is great for structured logging, especially when you need to parse and analyze logs later (e.g., for log management or cloud services). Winston supports the json
format, which outputs logs in a machine-readable format.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.json() // Format log messages as JSON
),
}),
],
});
logger.info('This log is in JSON format');
Output:
{
"message": "This log is in JSON format",
"level": "info",
"timestamp": "2025-01-02T10:00:00.000Z"
}
6. Combining Formats for Better Structure
You can stack multiple formats to create more detailed, structured logs that include timestamps, log levels, and even additional user-related fields. For example, you might want to track which user-generated the log.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.colorize(),
winston.format.timestamp(),
winston.format.printf(({ timestamp, level, message, user }) => {
return `${timestamp} [${level}] ${user ? `[User: ${user}]` : ''}: ${message}`;
})
),
}),
],
});
logger.info('This is a log with additional user information', { user: 'john_doe' });
Output:
2025-01-02T10:00:00.000Z [info] [User: john_doe]: This is a log with additional user information
7. Log Rotation and File Formatting
For logs written to files, it’s important to format them in a way that makes them easier to read and analyze later. You can combine timestamp, JSON, or custom formats to ensure that logs are well-structured in the file system.
Example:
const winston = require('winston');
require('winston-daily-rotate-file');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.DailyRotateFile({
filename: 'logs/application-%DATE%.log',
datePattern: 'YYYY-MM-DD',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json() // Log as JSON for structured logging
),
}),
],
});
logger.info('This log is written to a rotating log file');
This will create daily log files in JSON format, making it easier to process them later.
Logging Errors in Winston: Handling Uncaught Exceptions
In Winston, there are several methods for logging errors, including handling uncaught exceptions and unhandled promise rejections.
These ensure that your application can log even the most unexpected issues that occur during runtime.
Let’s explore how you can configure Winston to handle and log these error scenarios effectively.
1. Logging Errors with the Default Transport
Winston has built-in support for logging errors through its transport system. By default, you can use any of the transports (such as the console or file transport) to log error messages that you manually capture or throw.
const logger = winston.createLogger({
level: 'info', // Default log level
transports: [
new winston.transports.Console({ format: winston.format.simple() }),
],
});
logger.error('This is an error message.');
In this example, an error message is logged to the console. You can configure Winston to log errors to a file or remote service as needed.
2. Handling Uncaught Exceptions
Uncaught exceptions are errors that occur in the application but aren’t caught by any try-catch block. These errors typically crash the application if not handled properly.
Winston allows you to log these exceptions and prevent the application from crashing immediately and provides a built-in option for catching uncaught exceptions and logging them.
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console({ format: winston.format.simple() }),
new winston.transports.File({ filename: 'logs/uncaught_exceptions.log' }),
],
exceptionHandlers: [
new winston.transports.Console({ format: winston.format.combine(winston.format.colorize(), winston.format.simple()) }),
new winston.transports.File({ filename: 'logs/uncaught_exceptions.log' }),
],
});
throw new Error('This is an uncaught exception'); // This will be logged
- The
exceptionHandlers
property ensures that Winston catches and logs uncaught exceptions. - Errors are logged both to the console and to a file (
uncaught_exceptions.log
). - The application won’t crash immediately after an uncaught exception, allowing for better control and monitoring.
3. Handling Unhandled Promise Rejections
Promise rejections occur when a promise is rejected but no handler is attached (i.e., no .catch()
or .then()
to handle the rejection).
By default, unhandled promise rejections in Node.js can cause the application to crash, but it’s better to log them before crashing or terminating the process. Winston also has a built-in way to handle unhandled promise rejections.
process.on('unhandledRejection', (reason, promise) => {
logger.error('Unhandled Rejection at:', promise, 'reason:', reason);
});
Promise.reject(new Error('This is an unhandled promise rejection')); // This will be logged
- The
process.on('unhandledRejection')
event handler is used to catch unhandled promise rejections. - The rejection is logged using Winston, and the message is written to both the console and a log file.
This way, you ensure that no rejection goes unnoticed, even if it wasn’t properly caught or handled in the code.
4. Catching and Logging Errors in Async Functions
In asynchronous code, it’s common to encounter errors that may not be immediately apparent because they are part of a promise chain or async function.
If you want to ensure that errors in async functions are logged, you can use try-catch
blocks combined with Winston’s error logging.
async function someAsyncFunction() {
try {
throw new Error('This is an error in an async function');
} catch (error) {
logger.error('Caught an error:', error.message);
}
}
Here, an error within an async function is caught by the catch
block, and the error message is logged using Winston. This ensures that errors in asynchronous code are handled and logged consistently.
5. Custom Error Handling Middleware (For Web Servers)
If you’re using Winston in a web application (e.g., with Express), it’s a good practice to create custom error-handling middleware that logs errors from routes or middleware.
const app = express();
app.get('/error', (req, res) => {
throw new Error('Something went wrong!');
});
// Custom error handling middleware
app.use((err, req, res, next) => {
logger.error(`Error occurred: ${err.message}`);
res.status(500).send('Something went wrong!');
});
In this example, if an error is thrown in any route, the custom error-handling middleware logs the error using Winston and sends a generic response to the client.
Best Practices for Winston Logging in Node.js
While Winston offers flexibility, here are some best practices to follow for effective logging:
- Use Different Log Levels: Don’t log everything as
info
orerror
. Use a combination ofdebug
,warn
, anderror
to provide more meaningful insights, making it easier to filter logs by severity. - Log Contextually: Include context in your logs, such as user IDs, request IDs, or other relevant details. This extra context can help with troubleshooting.
- Avoid Overusing Debug Logs in Production: While
debug
logs are great for development, they can add unnecessary overhead in production. Adjust your log level in production environments to avoid clutter. - Log Error Stack Traces: Always log error stack traces, especially in asynchronous code. Stack traces can help you quickly pinpoint the root cause of issues.
- Rotate Log Files: Log files can grow large as your application scales. Use
winston-daily-rotate-file
to automatically rotate log files and prevent them from getting too big.
Conclusion
Winston is a versatile and powerful logging tool that helps you monitor your Node.js applications, tracking performance, health, and errors. Its flexibility and ease of use make it an invaluable asset for any Node.js developer, enabling more effective logging and troubleshooting.