You've heard the term thrown around in meetups and Slack channels, but what exactly is full-stack observability?
Simply put, you can see, understand, and quickly act on everything happening across your entire tech stack—from frontend user interactions to backend services, cloud infrastructure, and third-party integrations.
Full-stack observability isn't just another tech buzzword. It's the difference between being blindsided by outages and catching issues before your users tweet about them. It's about connecting the dots between disparate systems to create a unified view of performance and health.
In today's world of distributed systems, microservices, and cloud-native applications, traditional monitoring falls short. When an issue occurs, you need to understand not just that something broke, but why it broke, how it's affecting users, and what you can do to fix it—fast.
The Three Pillars of Effective Observability
Full-stack observability stands on three core pillars, each providing a unique lens through which to view your systems:
Metrics: Quantifiable Indicators That Signal System Health
Metrics are the numerical time-series data points that tell you how your systems are performing at a glance. They're typically collected at regular intervals and stored for trend analysis and alerting.
Key types of metrics include:
- Resource metrics: CPU, memory, disk usage, network throughput
- Application metrics: Request rates, error rates, response times
- Business metrics: Conversion rates, cart values, user signups
- Custom metrics: Specific to your application's unique requirements
The power of metrics lies in their ability to be aggregated, compared over time, and used to trigger alerts when they cross predefined thresholds.
Logs: Detailed Event Records for Root Cause Analysis
Logs are the timestamped records of discrete events happening in your systems. When metrics show something's wrong, logs help you figure out why by providing context and details.
Effective logging strategies include:
- Structured logging: Using consistent JSON formats instead of plain text
- Contextual logging: Including relevant IDs and metadata with each log
- Log levels: Properly categorizing logs as DEBUG, INFO, WARN, ERROR, etc.
- Centralized log management: Collecting logs from all sources in one place
Logs are particularly valuable during incident investigation, providing the breadcrumb trail that leads to the root cause.
Traces: End-to-End Request Journeys Across Distributed Services
Traces track requests as they travel through your distributed system, showing the entire journey from inception to completion. Each trace consists of multiple spans, representing operations within individual services.
Tracing helps you:
- Visualize request flows across complex service meshes
- Identify bottlenecks and latency issues
- Understand service dependencies
- Pinpoint exactly where errors occur in a request chain
In microservice architectures, tracing is often the only way to understand the complex interactions between dozens or hundreds of services.
Why Full-Stack Observability Is Critical for Modern DevOps Success
As a DevOps engineer, you're the bridge between development and operations, responsible for reliability, performance, and continuous improvement. Full-stack observability transforms how you approach these responsibilities:
Accelerated Incident Response and Resolution
With complete visibility across your stack, you can:
- Reduce mean time to detection (MTTD) by up to 80%
- Cut mean time to resolution (MTTR) from hours to minutes
- Eliminate finger-pointing between teams
- Automate the correlation of signals across different systems
A real-world example: When a payment processing issue occurs, you can immediately see which services are affected, which dependencies are failing, what errors are being logged, and how users are being impacted—all in one place.
Proactive Problem Prevention Through Anomaly Detection
Full-stack observability enables you to:
- Establish normal baseline performance for all systems
- Detect subtle deviations before they become critical
- Identify patterns that precede common failures
- Implement auto-remediation for known issues
Example: Your observability platform notices increasing error rates in your authentication service that historically precede outages, triggering an automated scaling event before users experience any issues.
Data-Driven Infrastructure and Application Optimization
With comprehensive observability data, you can:
- Right-size your infrastructure based on actual usage patterns
- Identify performance bottlenecks in application code
- Quantify the impact of proposed changes before implementation
- Measure the ROI of optimization efforts
// Suggested image: A before/after comparison showing infrastructure cost reduction and performance improvement after optimization based on observability data
Enhanced Cross-Team Collaboration and Communication
Full-stack observability creates a common language between teams:
- Developers see how their code performs in production
- Operations teams understand application requirements better
- Business stakeholders connect technical metrics to business outcomes
- Security teams identify potential vulnerabilities faster
Full-Stack Observability vs. Traditional Monitoring
Traditional Monitoring Approach | Full-Stack Observability Approach |
---|---|
Focuses primarily on infrastructure health | Covers the entire application delivery chain |
Siloed tools for different layers (network, servers, applications) | Unified view with context across all layers |
Alert-based and reactive to problems after they occur | Insight-based and proactive, catching issues early |
Primarily threshold-based alerting on individual metrics | Correlation and anomaly detection across multiple signals |
Limited historical data for trend analysis | Rich historical context for better pattern recognition |
Separated technical and business metrics | Integrated view of technical performance and business impact |
Requires manual correlation between different data sources | Automated correlation with AI/ML assistance |
Little to no end-user experience visibility | Complete visibility into real user experience |
How to Implement Full-Stack Observability
Step 1: Defining Clear Observability Objectives and Requirements
Before selecting tools, it's essential to understand what you need to observe and why. Start by:
- Mapping your critical services and their dependencies
- Identifying key performance indicators (KPIs) for each service
- Determining which user journeys are most important to monitor
- Establishing SLOs (Service Level Objectives) for critical paths
- Understanding regulatory and compliance requirements for monitoring
Questions to ask:
- What represents a good user experience for your application?
- Which services have the biggest impact on business outcomes?
- What types of incidents have caused the most pain in the past?
- Who needs access to observability data and for what purpose?
Step 2: Selecting the Right Tooling for Your Environment
The observability tools landscape is vast, but your selection should be guided by specific criteria:
Key Evaluation Criteria:
- Support for all three observability pillars (metrics, logs, traces)
- Integration capabilities with your existing technology stack
- Scalability to handle your data volume without breaking the bank
- Visualization tools that make complex data understandable
- Correlation capabilities across different signal types
- Alerting intelligence to reduce noise and alert fatigue
- Long-term data retention options for trend analysis
- Support for OpenTelemetry and other open standards
Popular Observability Platforms to Consider:
- Open-source combinations (Prometheus, Grafana, Jaeger, ELK)
- Commercial solutions (Last9, Dynatrace, Splunk)
- Cloud provider offerings (AWS CloudWatch, Azure Monitor, Google Cloud Operations)
Step 3: Implementing Proper Instrumentation Across Your Stack
The value of your observability platform depends entirely on the quality of data you feed into it. Effective instrumentation requires:
Application Instrumentation Best Practices:
- Adopting OpenTelemetry for standardized, vendor-neutral instrumentation
- Using automatic instrumentation where possible for common frameworks
- Adding custom instrumentation for business-specific insights
- Implementing distributed tracing with context propagation
- Using structured logging with consistent formats
- Adding business context to technical metrics
Infrastructure Instrumentation Considerations:
- Agent-based vs. agentless monitoring approaches
- Container and Kubernetes-specific observability
- Cloud service monitoring integration
- Network performance visibility
- Database query performance tracking
Code example for OpenTelemetry instrumentation in Node.js:
const { NodeTracerProvider } = require('@opentelemetry/node');
const { SimpleSpanProcessor } = require('@opentelemetry/tracing');
const { JaegerExporter } = require('@opentelemetry/exporter-jaeger');
// Set up the tracer provider
const provider = new NodeTracerProvider();
// Configure how spans are processed and exported
const exporter = new JaegerExporter({
serviceName: 'payment-service',
});
// Add the exporter to the provider
provider.addSpanProcessor(new SimpleSpanProcessor(exporter));
// Register the provider globally
provider.register();
// Later in your code, create and use spans
const tracer = provider.getTracer('payment-operations');
const span = tracer.startSpan('processPayment');
try {
// Process payment logic here
span.setStatus({ code: SpanStatusCode.OK });
} catch (error) {
span.setStatus({
code: SpanStatusCode.ERROR,
message: error.message
});
span.recordException(error);
throw error;
} finally {
span.end();
}
Step 4: Designing Actionable Dashboards and Visualizations
Great data is useless without great visualization. Your dashboards should:
Dashboard Design Principles:
- Present a clear hierarchy of information (overview → details)
- Show correlation between related metrics
- Highlight anomalies and deviations from normal
- Include business context alongside technical metrics
- Support drill-down from high-level metrics to detailed traces
- Provide both real-time and historical views
Types of Essential Dashboards:
- Executive dashboards for high-level service health
- Service-specific dashboards for engineering teams
- User journey dashboards tracking end-to-end experiences
- Infrastructure dashboards for resource utilization
- SLO/SLA dashboards for compliance tracking
Step 5: Implementing Intelligent Alerting to Reduce Noise
Alert fatigue is a major problem in DevOps. Your observability platform should help you:
Advanced Alerting Strategies:
- Moving from threshold-based to anomaly-based alerting
- Implementing alert correlation to reduce duplicate notifications
- Using different alert channels based on severity and time of day
- Automating triage and initial diagnostic steps
- Creating runbooks that link directly from alerts
Alert Prioritization Framework:
- P1: Service down, affecting all users
- P2: Degraded performance, affecting many users
- P3: Minor issues affecting some functionality
- P4: Potential issues that need investigation but aren't user-impacting
Common Full-Stack Observability Pitfalls and How to Avoid Them
Data Volume Overload and Cost Management Challenges
More data isn't always better. Without proper planning, you can end up with:
- Unmanageable storage costs
- Performance issues in your observability platform
- Too much noise to find useful signals
Solutions:
- Implement sampling strategies for high-volume data
- Use dynamic sampling that increases fidelity during incidents
- Set up data retention policies based on data importance
- Consider cardinality limits when designing custom metrics
- Regularly audit and clean up unused dashboards and alerts
Frontend and User Experience Monitoring Gaps
Many observability implementations focus heavily on backend systems while neglecting what users experience.
Solutions:
- Implement Real User Monitoring (RUM) to capture actual user interactions
- Track key frontend metrics like page load time, time to interactive, and client-side errors
- Capture user journey metrics across multiple pages and interactions
- Record session replays for understanding complex user issues
- Correlate frontend issues with backend services
Tool Fragmentation Leading to Context Switching
Having separate tools for different observability signals creates friction during incident response.
Solutions:
- Prioritize platforms that integrate all three observability pillars
- Use open standards like OpenTelemetry to avoid vendor lock-in
- Implement cross-linking between tools where possible
- Create unified dashboards that pull data from multiple sources
- Establish consistent naming conventions across all tools
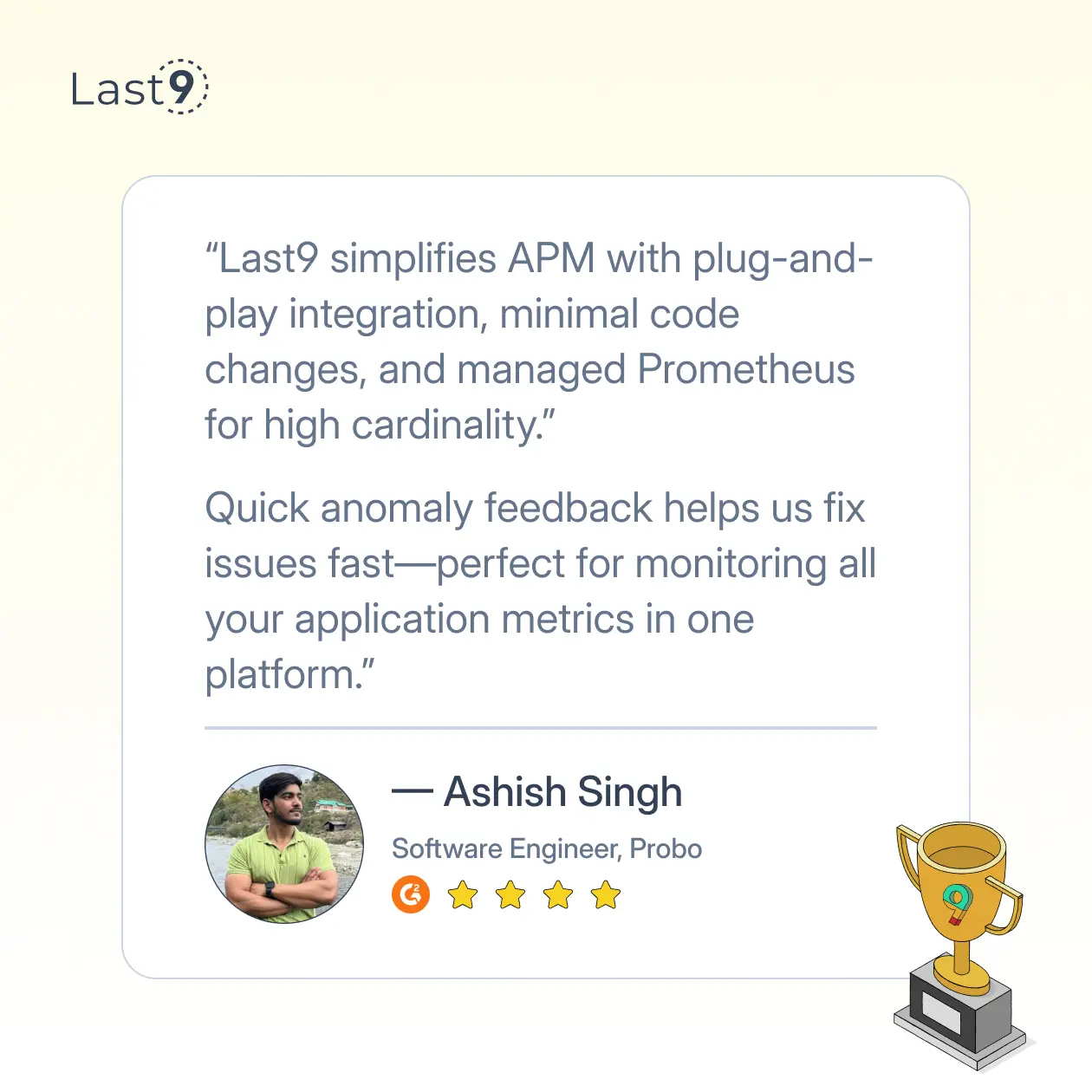
Missing Business Context in Technical Monitoring
Technical metrics mean little without connecting them to business outcomes.
Solutions:
- Tag all services with their business capabilities
- Track business KPIs alongside technical metrics
- Map user journeys to the underlying services that support them
- Calculate the revenue impact of performance issues
- Create executive dashboards that translate technical issues into business terms
Full-Stack Observability in Action During a Critical Incident
Picture this: Your e-commerce platform experiences a sudden 30% drop in checkout completions during a major promotion. Revenue is dropping by thousands of dollars per minute.
Without Full-Stack Observability:
- Users report checkout problems to customer service
- Customer service escalates to the web team
- The web team confirms the issue but sees no frontend errors
- Web team escalates to the backend team
- The backend team checks their services, which appear healthy
- After hours of investigation, someone discovers that a third-party payment API is rate-limiting requests
- A temporary fix is implemented, but you've lost significant revenue and customer trust
Time to resolution: 3+ hours
With Full-Stack Observability:
- Your anomaly detection alerts on the drop in checkout completion rate
- Your unified dashboard immediately shows:
- Frontend: Users getting payment errors
- Backend: Payment service calls taking 10x longer than normal
- Traces: Requests to third-party payment API timing out
- Logs: Rate limiting errors from the payment provider
- You implement a queueing mechanism to stay within rate limits
- You contact the payment provider to increase your limit
- You add a user-friendly message explaining the brief delay in processing
Time to resolution: 15 minutes
Future Trends in Full-Stack Observability Worth Watching
The observability landscape continues to evolve. Keep an eye on these emerging trends:
AIOps and Intelligent Observability
Machine learning algorithms are increasingly being applied to observability data to:
- Predict potential failures before they occur
- Automatically correlate related issues
- Suggest root causes during incidents
- Optimize system performance based on historical patterns
- Reduce alert noise through intelligent filtering
OpenTelemetry Becoming the Industry Standard
The OpenTelemetry project is unifying observability data collection:
- Vendor-neutral instrumentation
- Support across multiple languages and frameworks
- Consistent context propagation
- Unified metadata and semantic conventions
- Reduced need for proprietary agents
Observability-Driven Development
Observability is shifting left in the development process:
- Instrumentation as a first-class concern during development
- Pre-production observability testing
- Observability-as-code alongside infrastructure-as-code
- Developer-focused observability tools
- Performance testing based on production observability data
Wrapping Up
As systems grow more complex and distributed, your ability to understand what's happening across that distributed environment becomes your superpower.
Remember, the goal isn’t perfect observability of everything. On the other hand, if you need a cost-effective managed solution without compromising full-stack observability, Last9 is worth a try.
As a telemetry data platform, we’ve monitored 11 of the 20 largest live-streaming events in history. Integrating with OpenTelemetry and Prometheus, Last9 unifies metrics, logs, and traces—optimizing performance, cost, and real-time insights for correlated monitoring & alerting.
Schedule a demo or start for free today!