When something goes wrong in a system, figuring out what happened can be a real pain. If you’ve ever spent hours digging through messy, unorganized logs, you know the struggle. Structured logging changes all that by giving your logs a neat, consistent format that’s way easier to work with.
In this blog, we'll talk about structured logging, log formats, some best practices, and more.
What Is Structured Logging?
Structured logging is the practice of recording logs in a consistent, predefined format, typically using key-value pairs. The goal is to ensure that each log entry contains structured information that can be easily queried, filtered, and analyzed—whether by a human or an automated system.
With structured logs, you can:
- Enhance searchability: Each log field is clearly defined, allowing users and systems to filter, sort, and query logs based on specific criteria (e.g., all errors from a particular service).
- Improve automation: Systems can automatically process logs to detect patterns, calculate metrics, or trigger alerts when certain thresholds are reached.
- Support scalability: As systems grow, the ability to manage and analyze vast amounts of log data becomes increasingly important. Structured logs make it easier to scale observability across distributed systems.
- Ensure consistency: Structured logging enforces consistency, ensuring that logs from different services or components follow the same format and include the necessary information for debugging and analysis.
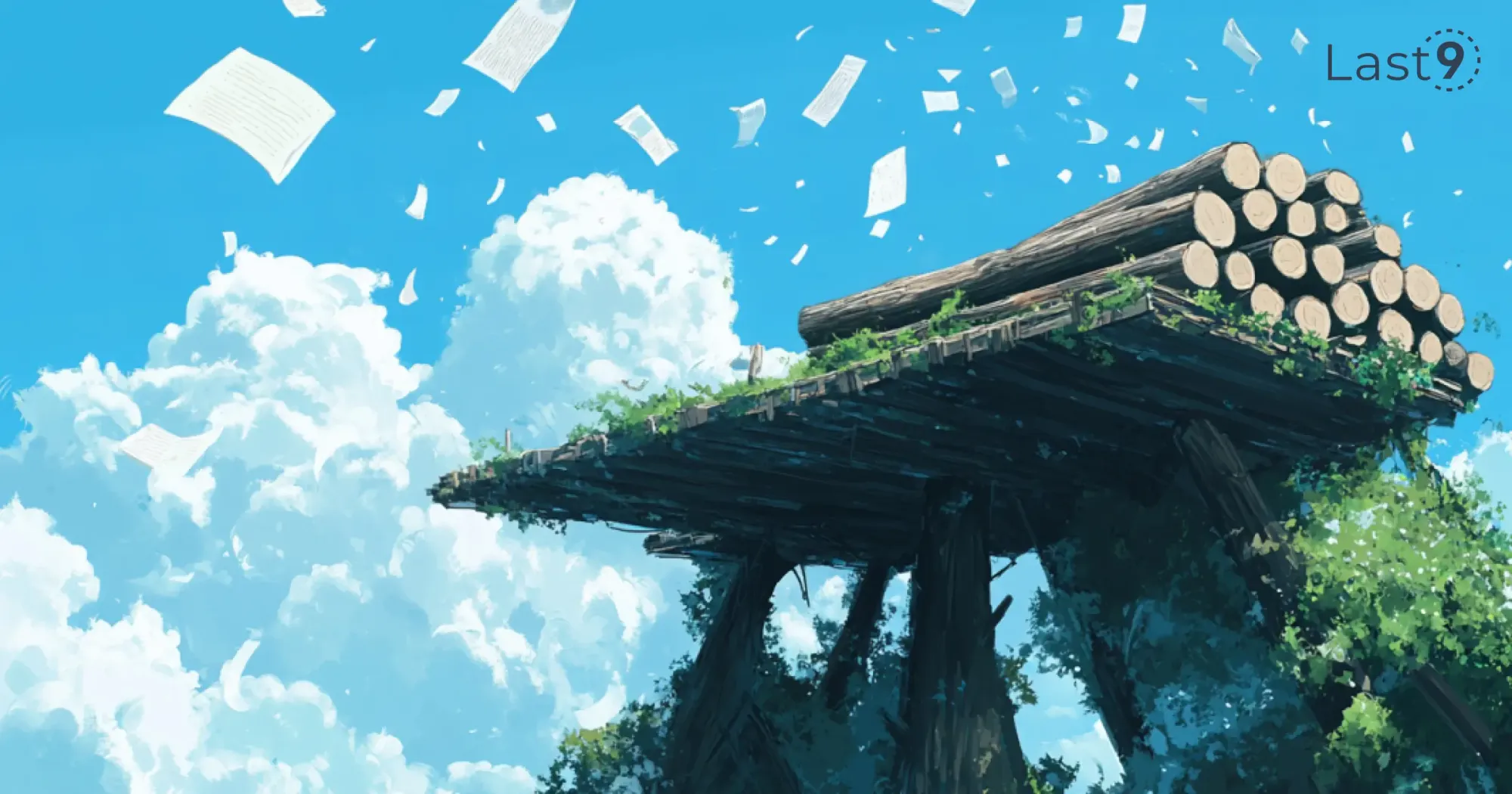
Understanding Log Formats
Logs are critical for understanding how an application behaves in production, especially when diagnosing issues or performing root cause analysis.
While traditional logging methods often work for simple applications, modern distributed systems need more advanced techniques to ensure logs are useful, consistent, and easy to parse. This is where structured logging comes in.
Traditional vs. Structured Logging Approaches
Traditional Logs:
Traditional logs are often simple text-based entries that contain timestamps, log levels, and messages. These logs are human-readable, but they can be difficult to process automatically at scale.
For instance, when logs are written as plain text, tools or systems that aggregate logs might struggle to extract meaningful insights, as they have to rely on regular expressions or string matching, which isn’t always efficient or accurate.
Example of a traditional log format.
2024-03-15 10:23:45 [ERROR] Main service encountered database timeout
While this works for humans reading logs manually, it lacks structure, making it harder for automated systems to parse and analyze efficiently.
For example, there's no easy way to extract information about the service, the event type, or the specific data related to the error (like the duration of the timeout).
Structured Logs:
Structured logging, on the other hand, uses a standardized format (like JSON, XML, or even key-value pairs) to organize log data into discrete, machine-readable fields. This allows logs to be easily parsed, queried, and analyzed by both humans and machines.
Here’s an example of structured logging in JSON format:
{
"timestamp": "2024-03-15T10:23:45Z",
"level": "ERROR",
"service": "main",
"event": "database_timeout",
"duration_ms": 5000,
"structured_data": {
"connection_pool": "primary",
"attempt": 3
}
}
This JSON structure is much richer and more detailed than the plain-text log. It includes:
timestamp
: The precise date and time the event occurred.level
: The severity of the log (in this case, an error).service
: Which part of the application does the log come from.event
: A brief description of the event, making it clear that this log relates to a database timeout.duration_ms
: The duration of the event, which could help understand performance issues.structured_data
: An optional field to include any additional contextual data (like which connection pool was involved or how many attempts were made).
The format itself can be expanded with any number of fields, making structured logging incredibly flexible.
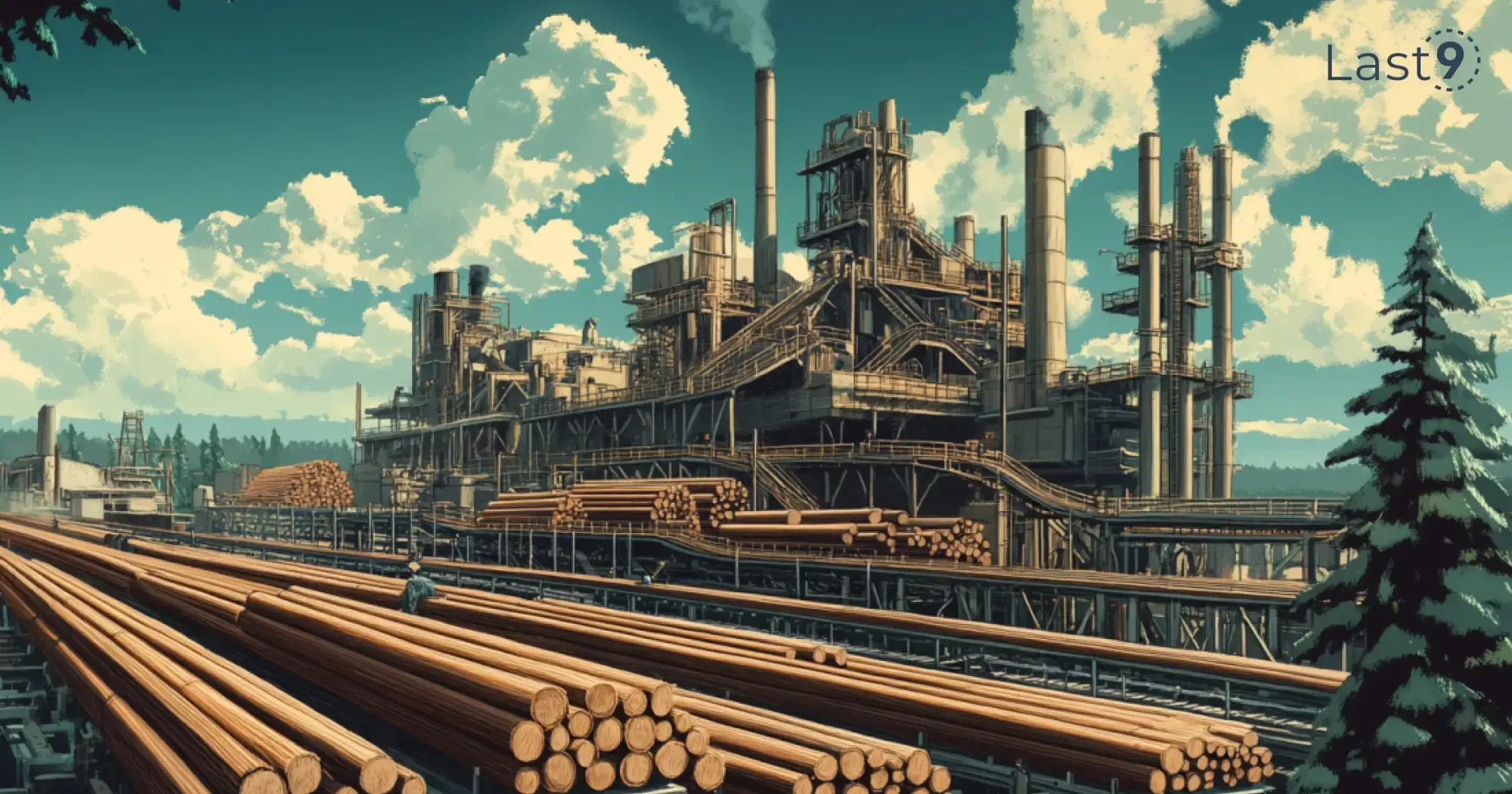
Alternative formats like XML or key-value pairs are also used in some systems, though JSON is the most commonly supported and widely used in modern applications.
Example of structured logging in XML format:
<log>
<timestamp>2024-03-15T10:23:45Z</timestamp>
<level>ERROR</level>
<message>Database timeout</message>
</log>
Why Use Structured Logging?
Today applications are often distributed across many services, containers, and environments, which makes debugging and monitoring challenging.
Structured logging helps to:
Ensure consistent log formats:
When logs are written in a standardized format, they can be processed by log aggregators, alerting systems, and monitoring tools without worrying about different formats or structures.
Support advanced querying and analysis:
With structured data, you can utilize powerful tools like Elasticsearch or Splunk to query logs based on specific fields, allowing you to identify patterns, errors, or performance bottlenecks quickly.
Simplify debugging:
Including key contextual information, such as service names, request IDs, or error codes, in each log entry makes it easier to trace the root cause of issues in complex, distributed systems. This structured approach helps you quickly pinpoint where things went wrong and simplify troubleshooting.
Implementation Fundamentals
To implement structured logging effectively, there are several core components to consider, from the data structure itself to the tools you’ll use for logging. Below, we break down key elements for success.
Structured Data Components
Every structured log entry should contain a well-defined set of fields to ensure consistency and provide essential context for analysis.
Here are the fundamental components:
- Timestamp: The exact time when the log entry was created. This is crucial for understanding the sequence of events and diagnosing issues in real time.
- Log Level: Indicates the severity of the log. Common log levels include
DEBUG
,INFO
,WARN
,ERROR
, andFATAL
. This allows you to filter logs based on the importance or urgency of the event. - Key-Value Pairs for Context: These are the additional fields that give meaningful context to the log. For example, request IDs, user information, service name, or event-specific details (like transaction amount or error codes). This extra information helps in tracing issues and understanding system behavior.
- Event Identifier: A unique identifier for the event being logged (e.g.,
order_processing_started
,database_timeout
). This helps categorize and quickly identify the nature of the logged event. - Application Logs Source: The source or component of the application generating the log (e.g., service name, environment, or module). This is useful for filtering logs from specific parts of a system or understanding which part of the application encountered an issue.
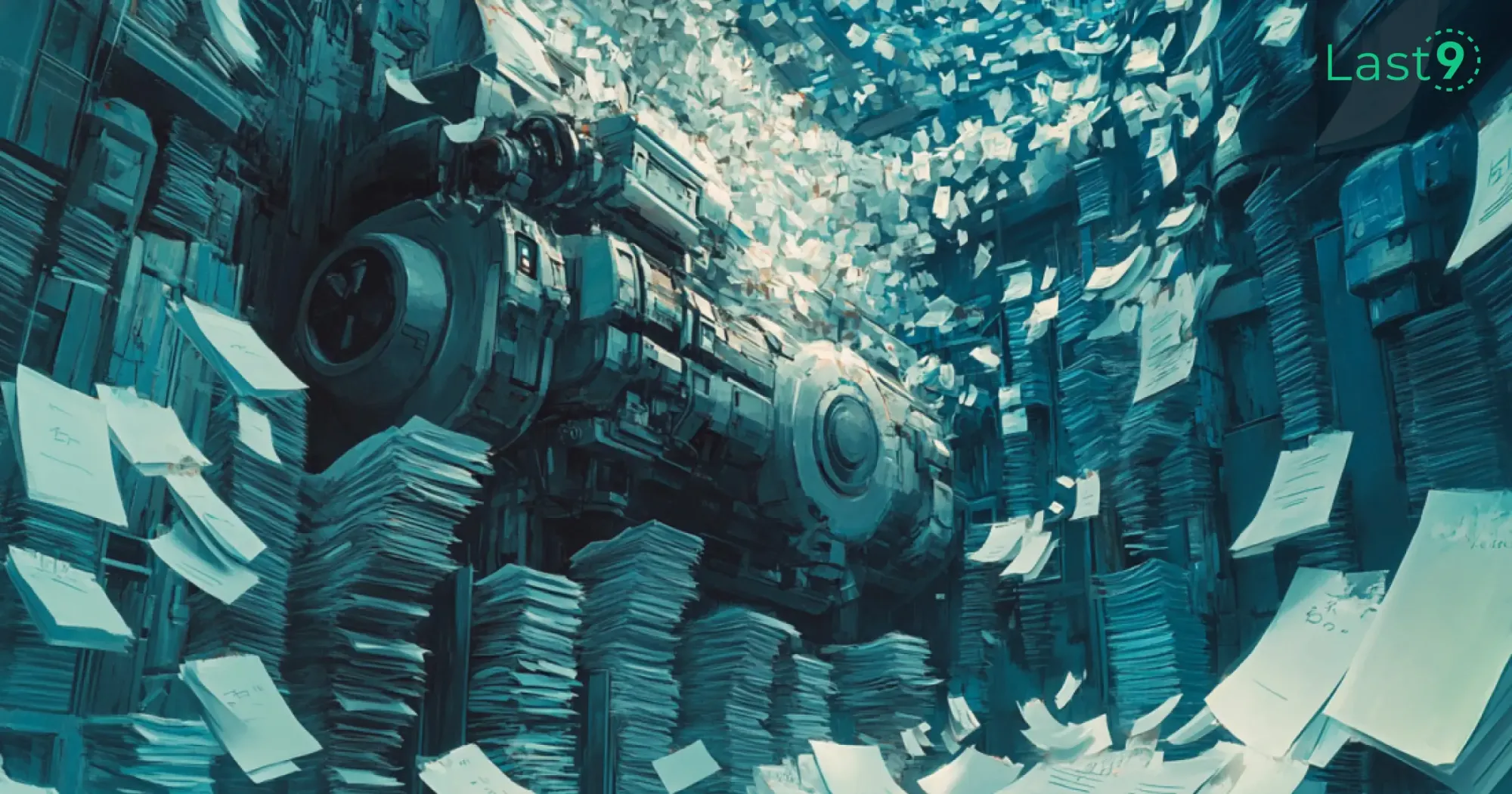
Logging Library Selection
Choosing the right logging library is key to implementing structured logging that works well with your application. When selecting a logging library, consider the following factors:
- Performance Characteristics: Logs can quickly pile up, especially in high-throughput systems. The library you choose should be lightweight and optimized for low overhead while capturing the necessary data.
- Format Support: Ensure the library supports the structured format that fits your needs, whether it’s JSON, XML, key-value pairs, or others. JSON is widely used because it integrates well with log aggregation tools.
- Integration Capabilities: Your logging library should integrate seamlessly with your existing monitoring and log aggregation systems, such as ELK Stack, Prometheus, or Grafana. This makes it easier to centralize and analyze logs.
- Open Source Community Support: Open-source libraries often come with community-driven improvements, bug fixes, and additional features. Look for libraries with a strong, active community.
Example Implementation
Let’s take a practical example to demonstrate how structured logging is implemented in Python using a hypothetical logging library:
from structured_logger import Logger
from datetime import datetime
# Initialize logger with application name and environment details
logger = Logger(app_name="order-service", environment="production")
def process_order(order_id):
try:
# Log the start of the order processing with relevant context
logger.info("order_processing_started", context={
"order_id": order_id,
"timestamp": datetime.utcnow()
})
# Processing logic (e.g., interacting with a database or external service)
# Imagine an exception occurs here...
except Exception as e:
# Log the failure with detailed error information
logger.error("order_processing_failed", error_details=str(e))
In this example:
- The logger is initialized with
app_name
andenvironment
for context. - The
process_order
function logs the start of order processing (INFO
level) and captures relevant context such as theorder_id
and timestamp. - If an error occurs during processing, it logs an
ERROR
level entry with detailed exception information.
This structured format makes it easy to track, search, and correlate logs, especially when dealing with high volumes of log data.
Log Management and Analysis
With structured logging in place, effective management and analysis come next. Modern log management systems handle vast log data and automate key processes.
Log Data Processing:
- Collection: Gathers logs from multiple sources in real time.
- Processing: Transforms raw logs into structured data with parsing and enrichment.
- Storage: Uses scalable, accessible storage, often cloud-based.
- Alerting: Sets automated alerts based on specific log patterns (e.g., error spikes).
- Automation: Automates responses like restarting services or scaling resources.
Root Cause Analysis:
Structured logs simplify root cause analysis by:
- Consistent Structure: Standardized format aids pattern recognition across systems.
- Searchable Fields: Find logs by fields (e.g.,
service_name
ortimestamp
). - Correlation: Trace requests across systems using identifiers like
transaction_id
. - Pattern Recognition: Many tools use machine learning to detect anomalies.
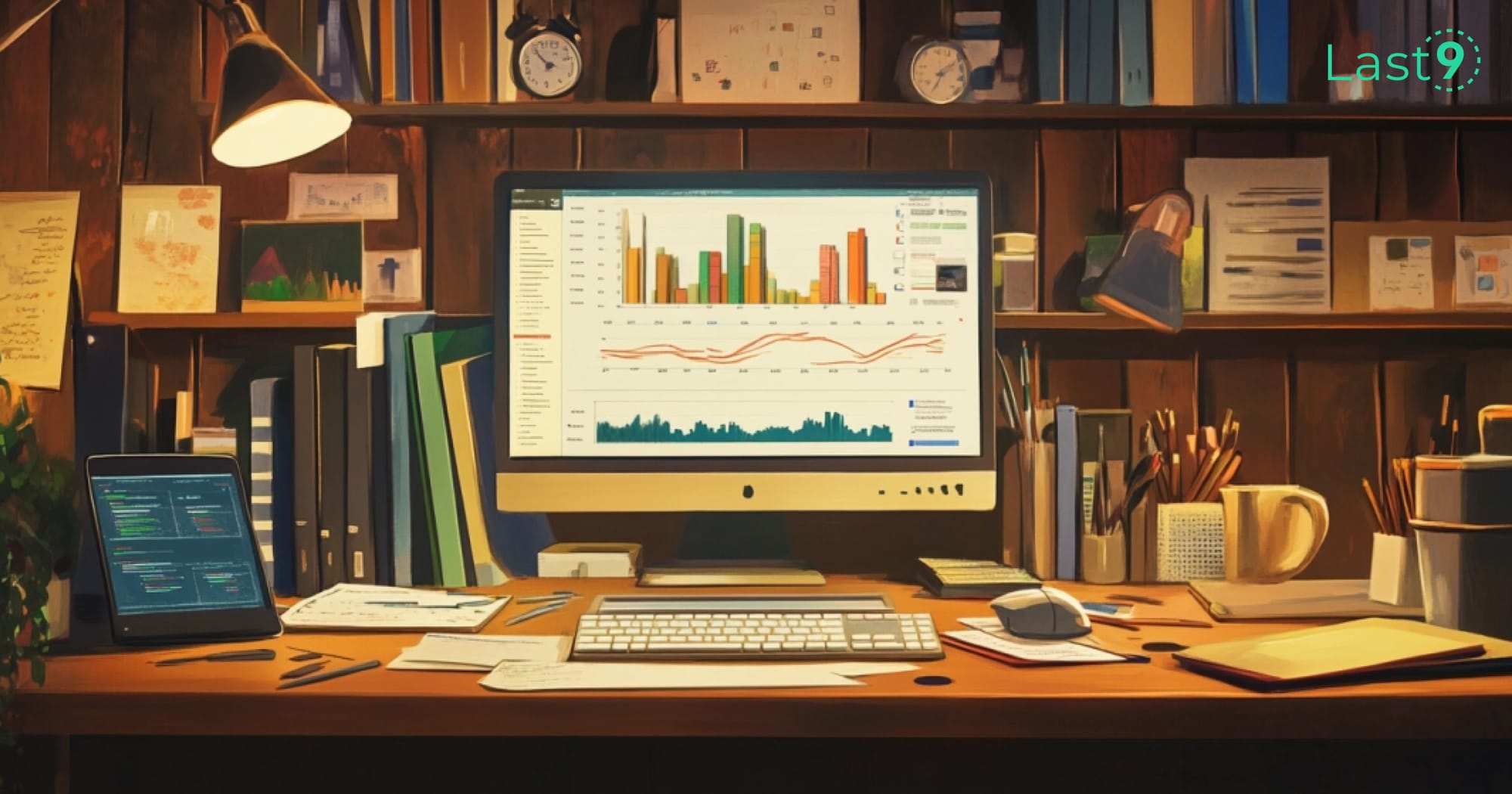
DevOps Integration:
Structured logging supports DevOps by:
- Automated Monitoring: Logs work with monitoring tools for real-time insights.
- Incident Response: Enables faster diagnosis and resolution with relevant data.
- Performance Analysis: Logs track performance, latency, and bottlenecks.
- Capacity Planning: Logs provide metrics for anticipating scalability needs.
Conclusion
Structured logging is like giving your logs a real purpose. Instead of sifting through piles of random text, you get clear, consistent details that help you solve problems faster and keep things running smoothly.
To take your observability to the next level, Last9 - an opentelemetry data warehouse can help you manage metrics, traces, and logs in one place. You can schedule a demo to learn more or start a free trial to explore the platform yourself.
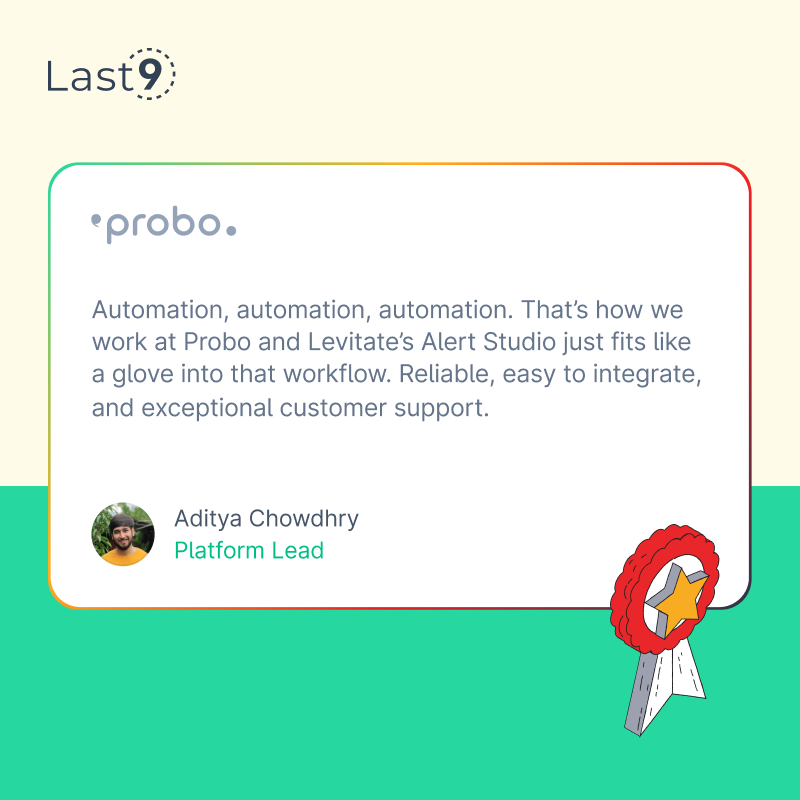
FAQs
What is the difference between structured and unstructured logging?
Structured logging organizes data into a predefined schema (key-value pairs), making it easier to parse, search, and analyze compared to unstructured logs, which are freeform text.
How do I implement structured logging?
- Choose a logging library
- Define your log schema
- Update logging calls to use the structured format
- Set up log shipping, storage, and management tools
Can I mix structured and unstructured logs?
Yes, but it’s better to maintain consistency. Use structured logs for important events and unstructured logs for simple debugging.
Which log format is better, JSON or XML?
JSON is preferred because it's lighter, more widely supported, and easier to work with in modern tools.
How do I handle high-volume logging in production?
Use log buffering, async logging, sampling, and proper log rotation to manage high-volume logs effectively.
What’s the impact on application performance?
Structured logging has minimal impact if implemented correctly. Use async logging, log buffering, and sampling to reduce overhead.
How does structured logging improve root cause analysis?
It provides consistent data formats, enables cross-service correlation, supports advanced search, and facilitates automated analysis.
What are best practices for log retention?
Define retention periods based on compliance, costs, and troubleshooting needs. Implement log rotation and backups.
How does structured logging fit into DevOps?
It enhances DevOps with automated monitoring, alerting, CI/CD integration, and faster incident response.
Which open-source tools are recommended?
For logging, try Winston (Node.js), Serilog (.NET), or Logback (Java). For log management, use ELK Stack, Grafana Loki, or Fluentd.
How should I handle sensitive data in structured logs?
Use data masking, field-level encryption, and access controls to protect sensitive information in logs.
Can machine learning be applied to structured logs?
Yes, machine learning can be used for anomaly detection, pattern recognition, and automated root cause analysis.
How should I structure logs in a microservices architecture?
Ensure consistent formatting, propagate correlation IDs, and centralize log aggregation.
What’s the recommended approach for logs in containerized environments?
Write logs to stdout/stderr, use container runtime logging drivers, and implement log aggregation for scalability.
How do I migrate from traditional to structured logging?
Start with new features, gradually update existing code, and use parallel logging during the transition.
How often should I review my logging strategy?
Review regularly based on system changes, tool capabilities, performance metrics, and storage costs.