We all know time is precious, especially when your application relies on tasks that need to be done repeatedly. The Laravel Scheduler is the tool that helps you automate and manage those tasks effortlessly.
But how does it work, and what makes it so powerful? Don’t worry, we’ve got you covered! In this guide, we’ll walk you through everything you need to know to get started.
What Is the Laravel Scheduler?
The Laravel Scheduler is a feature built on top of the Cron system, allowing developers to define and manage scheduled tasks using a fluent, expressive syntax. Gone are the days of writing complex Cron expressions for each job; Laravel simplifies the process, making it accessible even for beginners.
Why Use the Laravel Scheduler?
- Readability: The syntax is clean and easy to understand.
- Flexibility: Schedule tasks with precision, from every minute to specific days.
- Error Handling: Integrated features like logging and conditional execution make debugging simpler.
How to Setup the Laravel Scheduler
1. Install Laravel
If you haven’t set up Laravel yet, you can create a new Laravel project with the following command:
composer create-project --prefer-dist laravel/laravel scheduler-app
2. Set Up Cron
The Laravel Scheduler relies on a single Cron entry to execute tasks. Add the following line to your server's Cron configuration:
* * * * * php /path-to-your-project/artisan schedule:run >> /dev/null 2>&1
This ensures that Laravel’s scheduler runs every minute to check for any tasks that need to be executed.
Defining Scheduled Tasks
Laravel stores scheduled tasks in the App\Console\Kernel.php
file. Here’s an example of how to schedule a task:
protected function schedule(Schedule $schedule) {
$schedule->command('emails:send')
->hourly();
}
This schedules the emails:send
command to run every hour.
Different Scheduling Options in Laravel Scheduler
Laravel offers a variety of scheduling options to fit different needs:
Time Intervals
To run a task on a specific time interval, you can use:
$schedule->command('backup:run')->daily();
This schedules the backup:run
command to run daily.
Days and Times
If you want to run a task on specific days and times, you can use:
$schedule->command('cleanup:files')->mondays()->at('14:00');
This schedules the cleanup:files
command to run every Monday at 2 PM.
Conditional Execution
Laravel also allows conditional execution of tasks:
$schedule->command('report:generate')
->daily()
->when(fn () => true);
This schedules the report:generate
command to run daily, but only if the condition (in this case, true
) is met.
Advanced Features of Laravel Scheduler
1. Task Output and Logging
Laravel allows you to capture and log task output using the sendOutputTo
method:
$schedule->command('import:data')
->daily()
->sendOutputTo('/path/to/output.log');
This stores the output of the task in a specified log file for later review.
2. Ping Services for Monitoring
You can integrate third-party monitoring tools by pinging a URL after task execution:
$schedule->command('backup:run')
->daily()
->onSuccess(fn () => Http::get('https://my-monitoring-service/ping-success'));
This sends a "success" ping to your monitoring service once the backup task is completed successfully.
3. Dynamic Schedules
Laravel also supports dynamic task scheduling, allowing you to schedule tasks based on data from your database. For example, you can notify users at their preferred time:
$users = User::active()->get();
foreach ($users as $user) {
$schedule->call(fn () => $this->notifyUser($user))
->dailyAt($user->preferred_time);
}
This schedules each user's notification based on their preferred time.
How to Combine Scheduler with Queues
To improve task efficiency, you can combine the Scheduler with Laravel Queues. For example:
$schedule->job(new ProcessDataJob)->everyThirtyMinutes();
This ensures that tasks are queued and processed asynchronously, helping you avoid bottlenecks and improving overall performance.
Maintenance Mode and the Laravel Scheduler
When your Laravel application enters maintenance mode, managing scheduled tasks can get tricky. However, Laravel offers built-in solutions to make sure tasks don’t interfere with maintenance or cause unexpected issues.
Skipping Scheduled Tasks
Laravel prevents scheduled tasks from running during maintenance mode by default. This helps avoid unintended errors or background processes during downtime. If your tasks don’t need to run while the app is in maintenance mode, you don’t need to do anything—Laravel will handle it for you.
Overriding the Default Behavior
In some cases, you may want certain tasks—like backups or system health checks—to run even when your app is in maintenance mode. Laravel allows you to override the default behavior with the --onMaintenance
flag in your schedule definition.
Example:
$schedule->command('backup:run')->onMaintenance()->daily();
This ensures that specific tasks, like backups, will run regardless of whether the application is in maintenance mode.
Testing Maintenance-Safe Schedules
If you need tasks to run during maintenance, it's crucial to test them thoroughly. This will confirm they won’t disrupt updates or create data inconsistencies. Careful testing helps ensure your app stays stable while you handle maintenance tasks.
With Laravel’s thoughtful design, managing scheduled tasks during maintenance mode becomes easy, allowing you to focus on keeping your app running smoothly.
How to Prevent Task Overlaps in Laravel Scheduler
When managing scheduled tasks, overlaps can lead to issues like duplicate processes, resource contention, or even crashes. Laravel offers several strategies to ensure tasks run smoothly without stepping on each other's toes.
Using the withoutOverlapping
Method
Laravel’s withoutOverlapping
method is a simple yet powerful tool for preventing multiple instances of the same task from running at the same time. When this method is used, Laravel creates a lock that ensures the task won't start again until the previous instance has finished.
Example:
$schedule->command('emails:send')
->hourly()
->withoutOverlapping();
If the task takes longer than expected, the next scheduled run will wait until the current one is completed, effectively preventing overlaps.
Setting Overlap Expiration
In some rare cases, a lock might persist longer than necessary due to unexpected task failures. Laravel allows you to define a maximum lock duration with the ->onOneServer()->withoutOverlapping($expiresAt)
method.
Example:
$schedule->command('data:process')
->everyTenMinutes()
->withoutOverlapping(30); // Expires after 30 minutes
This ensures that if a lock lasts longer than 30 minutes, it will be released, allowing new instances of the task to run.
Ensuring Locking Works Across Servers
For distributed environments with multiple servers, Laravel’s onOneServer
method ensures that a task only runs on a single server, avoiding overlap issues in multi-server setups.
Example:
$schedule->command('cleanup:run')
->daily()
->onOneServer()
->withoutOverlapping();
Monitoring and Testing
Even with these safeguards in place, regular testing and monitoring are essential. Regularly check your task execution logs to ensure that tasks are completed as expected and that overlaps are properly avoided.
Running Tasks on One Server in Laravel Scheduler
In a multi-server environment, running the same scheduled task on all servers can lead to duplication, wasted resources, and even data conflicts. Laravel provides features to ensure tasks run only on a designated server, keeping everything simplified.
The onOneServer
Method
The onOneServer
method ensures that a scheduled task runs only on one server in your cluster. This is especially useful for tasks like sending emails, generating reports, or processing queues, where duplication would cause significant issues.
Example:
$schedule->command('emails:send')
->hourly()
->onOneServer();
When this method is applied, Laravel uses a distributed lock mechanism to guarantee that only one server runs the task at a time.
Configuring the Cache Driver
For onOneServer
to work, you need a cache driver that supports locks, such as Redis or Memcached. The file or database cache drivers won’t work for this functionality.
Make sure your .env
file is configured for a compatible cache driver:
CACHE_DRIVER=redis
Combining with withoutOverlapping
You can pair onOneServer
with withoutOverlapping
to further ensure no duplicate tasks run, even if a task takes longer than its scheduled interval.
Example:
$schedule->command('data:process')
->everyTenMinutes()
->onOneServer()
->withoutOverlapping();
Using Task Queues
If your scheduled task enqueues jobs for processing, make sure your queue workers are also configured to avoid duplication. Consider using Laravel Horizon for better visibility and control over your queues in a multi-server setup.
Testing in Multi-Server Environments
Always test your configuration thoroughly in a staging environment before deploying it to production. This helps ensure that cache locks are correctly implemented and tasks only run on one server as intended.
With onOneServer
, Laravel makes it simple to prevent task duplication, keeping your operations efficient and conflict-free.
How to Schedule Artisan Commands in Laravel
Artisan commands are key to automation in Laravel, allowing developers to perform tasks like database migrations, sending emails, or cleaning up logs. Scheduling these commands automates repetitive tasks, saving both time and effort.
Here’s how you can schedule Artisan commands using Laravel’s scheduler.
Defining Scheduled Commands
Laravel’s task scheduler relies on the schedule
method within the App\Console\Kernel
class. To schedule an Artisan command, you simply use the command
method.
Example: To schedule a custom Artisan command to run daily:
protected function schedule(Schedule $schedule)
{
$schedule->command('emails:send')->daily();
}
In this example, emails:send
refers to the Artisan command you've defined, or it could be one of Laravel’s built-in commands.
Customizing Execution Times
Laravel provides a variety of frequency methods to control when a command runs. Here are some options:
->hourly()
– Runs every hour.->daily()
– Runs every day at midnight.->weeklyOn(1, '8:00')
– Runs every Monday at 8:00 AM.->everyMinute()
– Runs every minute (use with caution).
Example:
$schedule->command('reports:generate')->weeklyOn(5, '17:00'); // Every Friday at 5 PM
Adding Additional Options
You can pass flags or parameters to your Artisan commands directly in the scheduler for more control:
Example:
$schedule->command('cache:clear --force')->dailyAt('2:00');
This allows you to fine-tune the behavior of your commands.
Logging Command Output
To keep track of the output of your scheduled commands, you can use the ->sendOutputTo
or ->appendOutputTo
methods:
Example:
$schedule->command('data:import')
->daily()
->sendOutputTo(storage_path('logs/data_import.log'));
Testing Scheduled Commands
Before putting your scheduled commands into production, it's a good idea to test them manually using Artisan’s schedule:run
command:
php artisan schedule:run
This simulates the execution of tasks that are scheduled to run at the current time.
Monitoring and Debugging
Monitor your scheduled commands using Laravel’s logs or tools like Horizon to ensure they execute as expected. Debugging becomes much easier with these tools, ensuring your scheduled tasks run smoothly.
Scheduled Artisan commands are a powerful way to automate tasks and keep your Laravel application running efficiently.
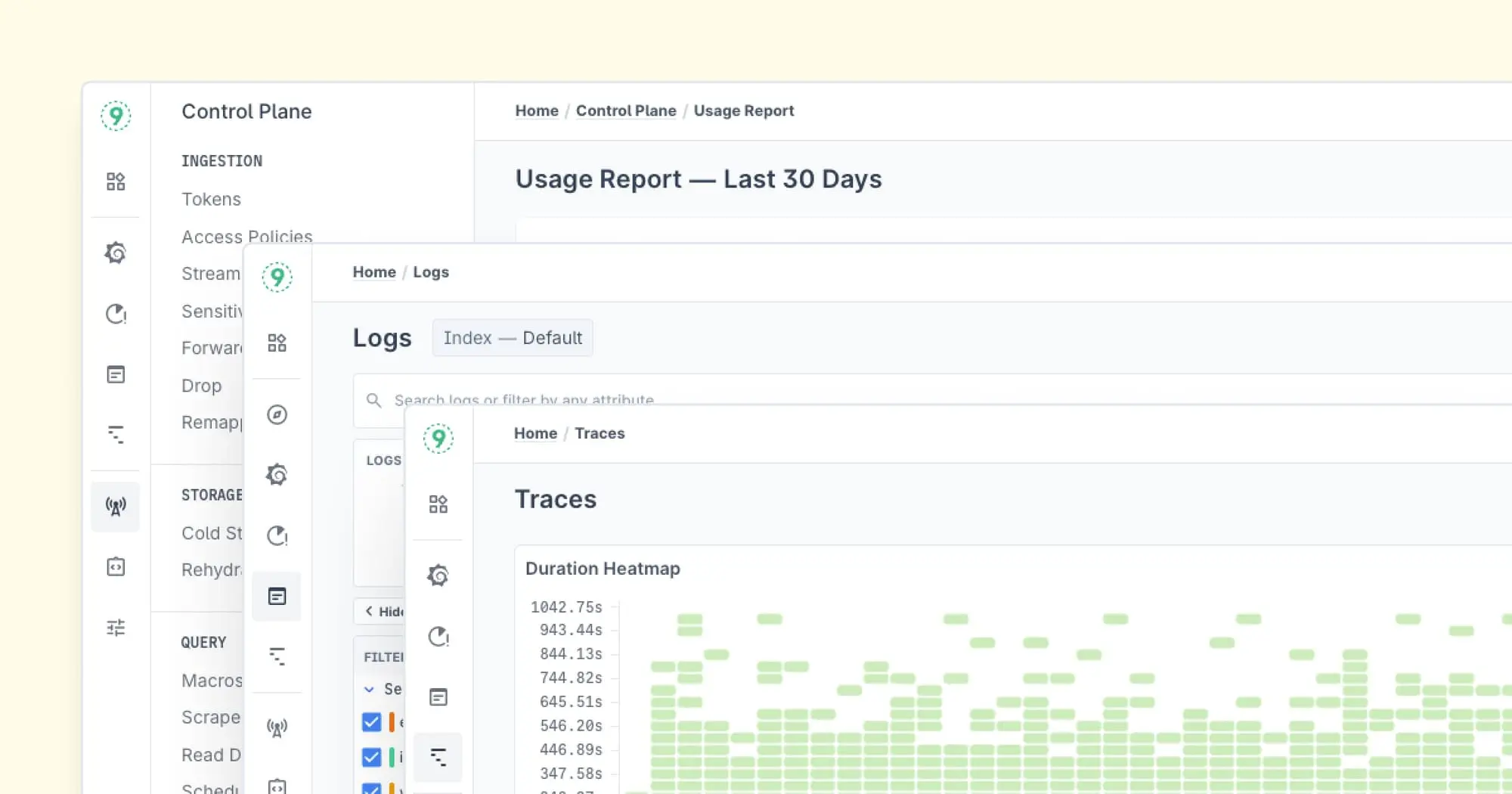
What are Task Hooks in Laravel Scheduler?
Task hooks in Laravel provide actions that can run before or after a scheduled task, adding flexibility and making it easier to prepare for tasks or respond to their outcomes. Let’s explore how they work and how to use them effectively.
Available Task Hooks
Laravel provides two main hooks for scheduled tasks:
- before Hook: Runs just before the scheduled task begins. It's useful for setup or initialization tasks.
- after Hook: Runs immediately after the scheduled task completes. Perfect for cleanup or logging actions.
Using before and after Hooks
To attach hooks to a scheduled task, use the before
and after
methods in the scheduler:
Example:
$schedule->command('emails:send')
->daily()
->before(function () {
// Action to take before the task runs
Log::info('Preparing to send emails...');
})
->after(function () {
// Action to take after the task completes
Log::info('Emails sent successfully!');
});
Handling Failures with onFailure
If you want to take specific actions when a task fails, you can use the onFailure
hook. This is great for error handling or notifying your team:
Example:
$schedule->command('data:import')
->hourly()
->onFailure(function () {
Log::error('Data import failed!');
});
Adding onSuccess Hooks
Similarly, you can use the onSuccess
hook to trigger actions when a task finishes without errors:
Example:
$schedule->command('backup:run')
->weekly()
->onSuccess(function () {
Log::info('Backup completed successfully.');
});
Combining Hooks
You can combine all hooks to create a comprehensive task management process:
Example:
$schedule->command('reports:generate')
->dailyAt('23:00')
->before(function () {
Log::info('Starting report generation...');
})
->onSuccess(function () {
Log::info('Report generated successfully.');
})
->onFailure(function () {
Log::error('Failed to generate report.');
})
->after(function () {
Log::info('Report generation process finished.');
});
Practical Use Cases
- before: Load configurations, clean temporary files, or notify stakeholders.
- after: Archive old logs, free up resources, or send completion notifications.
- onSuccess: Update dashboards, mark tasks as completed, or trigger subsequent jobs.
- onFailure: Send alerts, retry tasks, or log detailed error information.
Task hooks allow you to orchestrate related actions, keeping your scheduled tasks organized and adaptable.
Wrapping Up
The Laravel Scheduler is a game-changer for handling repetitive tasks in your app. Its clean syntax and powerful features make automating everything—from sending emails to cleaning up old files—a breeze.
The key to getting the most out of it is in the details and exploring those advanced features.
FAQs
What is the Laravel Scheduler?
The Laravel Scheduler is a built-in feature in Laravel that allows you to automate the execution of tasks like running commands, cleaning up files, or sending emails at specified intervals. It simplifies scheduling and managing repetitive tasks within your application.
How do I set up the Laravel Scheduler?
To set up the Scheduler, you need to add a Cron entry to your server that runs Laravel’s schedule:run command every minute. This ensures the scheduler can execute tasks defined in your App\Console\Kernel.php
file.
How can I schedule custom Artisan commands?
You can schedule custom Artisan commands by using the schedule
method inside the App\Console\Kernel
class. For example, to run a custom command daily, you can use:
$schedule->command('emails:send')->daily();
What are task hooks, and how do they work?
Task hooks allow you to add actions that run before or after a scheduled task. For example, the before
hook runs before the task starts, and the after
hook runs when the task finishes. They help you manage setup, logging, or cleanup around your tasks.
Can I prevent overlapping tasks in Laravel Scheduler?
Yes, you can prevent overlapping tasks by using the withoutOverlapping
method. This ensures that a task won’t start until the previous instance has finished running, which is helpful for long-running tasks.
How do I run tasks on a single server in a multi-server environment?
In a multi-server setup, you can use the onOneServer
method to ensure a scheduled task runs only on one server. This avoids duplication and ensures tasks like backups or emails are not processed on multiple servers at once.
Can I customize when scheduled tasks run?
Yes, Laravel offers various methods to customize when tasks run. For example, you can schedule tasks to run hourly, daily, weekly, or even at specific times with methods like ->hourly()
, ->daily()
, or ->weeklyOn()
.
How can I log the output of scheduled tasks?
You can log the output of scheduled tasks using methods like sendOutputTo()
or appendOutputTo()
. For instance:
$schedule->command('data:import')->daily()->sendOutputTo(storage_path('logs/data_import.log'));
What happens when the app is in maintenance mode?
By default, Laravel prevents scheduled tasks from running when the app is in maintenance mode. However, you can override this behavior for critical tasks, like backups, by using the onMaintenance
flag.
How do I monitor scheduled tasks?
You can monitor scheduled tasks using Laravel’s logging system or third-party tools like Laravel Horizon. Horizon provides a dashboard for better visibility and control over queue workers, especially in multi-server environments.
Can I combine multiple hooks for one task?
Yes, you can combine multiple hooks like before
, after
, onFailure
, and onSuccess
for one task. This gives you complete control over what happens before, during, and after the execution of your scheduled tasks.
How do I handle task failures in Laravel Scheduler?
You can use the onFailure
hook to define actions when a task fails. This can be used for error logging, notifications, or retries, ensuring that you can handle failures gracefully.