When a Java application starts slowing down, garbage collection is often a good place to look. For engineers responsible for keeping systems stable and responsive, understanding GC logs can make a real difference. This guide walks through the basics—what to look for, what the logs mean, and how to troubleshoot common issues—so you can get ahead of problems before they impact performance.
What Are Java GC Logs?
Java GC logs are detailed records of how the Java Virtual Machine (JVM) manages memory. They show when garbage collection happens, how long it takes, and how much memory is freed up. Think of them as your application's memory health report – they tell you if your app's memory diet is working or if it's time for a change.
These logs track the JVM's automatic memory management system that identifies and removes objects no longer needed by your application, freeing up space for new objects. Without garbage collection, Java apps would eventually consume all available memory and crash.
These logs contain valuable data about:
- Collection types (minor, major, full)
- Collection duration
- Memory before and after collection
- Memory allocation and reclamation patterns
- Pause times that affect application responsiveness
- Memory regions affected (Eden space, Survivor spaces, Old generation)
- Specific collection phases (marking, sweeping, compacting)
- Concurrent vs. stop-the-world activities
Each line in a GC log represents a specific garbage collection event, with timestamps, memory measurements, and duration metrics that help you understand exactly what's happening under the hood of your Java application.
Why You Should Care About Java GC Logs
As a DevOps engineer, you might wonder why you should add another log type to your monitoring stack. Here's why Java GC logs deserve your attention:
- Performance troubleshooting – Identify when garbage collection is slowing down your app
- Memory leak detection – Spot patterns that suggest memory isn't being properly released
- Capacity planning – Get hard data on memory usage to inform infrastructure decisions
- JVM tuning – Use concrete evidence to adjust heap sizes and collection algorithms
How to Enable Java GC Logs
Turning on Java GC logs is straightforward. You just need to add the right flags to your Java command line. The exact flags vary by Java version, so make sure you're using the correct syntax.
Java 8 GC Logging Options
Basic logging:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:/path/to/gc.log
Enhanced logging with causes and timestamps:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -XX:+PrintGCCause -XX:+PrintTenuringDistribution -Xloggc:/path/to/gc.log
With application stops tracking:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -XX:+PrintGCApplicationStoppedTime -Xloggc:/path/to/gc.log
Java 11 and Newer GC Logging Options
Basic logging:
-Xlog:gc*=info:file=/path/to/gc.log:time,uptime,level,tags
Detailed logging:
-Xlog:gc*=info,gc+phases=debug:file=/path/to/gc.log:time,uptime,level,tags
Complete debug-level logging:
-Xlog:gc*=debug:file=/path/to/gc.log:time,uptime,level,tags
Log Rotation Settings
For Java 8:
-XX:+UseGCLogFileRotation -XX:NumberOfGCLogFiles=10 -XX:GCLogFileSize=50M
For Java 11+:
-Xlog:gc*=info:file=/path/to/gc.log:time,uptime,level,tags:filecount=10,filesize=50M
Enabling in Production Environments
For containerized applications (Docker, Kubernetes), add these flags to your JAVA_OPTS
environment variable or directly in your Dockerfile:
ENV JAVA_OPTS="$JAVA_OPTS -Xlog:gc*=info:file=/logs/gc.log:time,uptime,level,tags:filecount=10,filesize=50M"
Make sure your container has a volume mounted for the logs directory to persist the GC logs outside the container.
Understanding the Different Garbage Collectors
The way your GC logs look depends on which garbage collector you're using. Each collector has distinct strategies, strengths, and weaknesses, which directly affect your application's performance profile. Let's explore each one in detail:
Serial Collector
Flag to enable: -XX:+UseSerialGC
The simplest garbage collector that uses a single thread for all collection activities. It completely stops the application during garbage collection (stop-the-world).
Best for:
- Small applications (less than 100MB heap)
- Systems with a single CPU/core
- Batch processing jobs where pauses don't matter
- Applications with very small memory footprints
GC Log Characteristics:
- Simpler log entries without parallel thread information
- Usually longer pause times but less CPU overhead
- Log lines typically include "[DefNew" for young generation collections
Example log entry:
2023-05-15T14:22:33.156+0000: 2.729: [GC (Allocation Failure) 33280K->5096K(125952K), 0.0072848 secs]
Parallel Collector
Flag to enable: -XX:+UseParallelGC
Uses multiple threads for collection, which speeds up the collection process. Still causes stop-the-world pauses but they're shorter than with the Serial collector.
Best for:
- Applications where throughput is more important than response time
- Batch processing systems
- Applications with multi-core systems that can benefit from parallel processing
- Systems with adequate CPU resources
GC Log Characteristics:
- Log entries include "[PSYoungGen" for young gen collections
- Times include user/sys CPU time, which is often higher than real time
- Log lines may show thread counts and thread-specific timings
Example log entry:
2023-05-15T14:22:33.156+0000: 2.729: [GC (Allocation Failure) [PSYoungGen: 33280K->5088K(38400K)] 33280K->5096K(125952K), 0.0072848 secs] [Times: user=0.02 sys=0.00, real=0.01 secs]
CMS (Concurrent Mark Sweep)
Flag to enable: -XX:+UseConcMarkSweepGC
Minimizes pause times by performing most of its work concurrently while the application runs. Only stops the application for short periods during collection.
Best for:
- Applications requiring low latency response times
- Interactive applications with users waiting for responses
- Systems where user experience is critical
- Applications that can spare some CPU resources for concurrent GC work
GC Log Characteristics:
- Complex log entries with multiple phases (initial mark, concurrent mark, remark, concurrent sweep)
- Lower pause times but higher overall CPU usage
- Logs show concurrent phases with timestamps for each phase
Example log entry:
2023-05-15T14:22:33.156+0000: 2.729: [GC (CMS Initial Mark) [1 CMS-initial-mark: 10984K(87424K)] 16078K(126848K), 0.0023213 secs] [Times: user=0.00 sys=0.00, real=0.00 secs]
2023-05-15T14:22:33.159+0000: 2.732: [CMS-concurrent-mark-start]
2023-05-15T14:22:33.209+0000: 2.782: [CMS-concurrent-mark: 0.050/0.050 secs] [Times: user=0.05 sys=0.00, real=0.05 secs]
G1 (Garbage First)
Flag to enable: -XX:+UseG1GC
(default in Java 9+)
Designed for large heaps, G1 divides the heap into regions and collects regions with the most garbage first. It aims to provide a balance between latency and throughput.
Best for:
- Applications with large heaps (>4GB)
- Systems requiring predictable pause times
- Applications needing both good throughput and low pause times
- Modern microservices and containerized applications
GC Log Characteristics:
- Log entries include region information
- Evacuation pauses and mixed collections clearly identified
- Concurrent marking phases similar to CMS but with different terminology
- Detailed information about regions collected
Example log entry:
[2023-05-15T14:22:33.156+0000] GC(10) Pause Young (Normal) (G1 Evacuation Pause) 33M->5M(128M) 7.285ms
[2023-05-15T14:22:35.256+0000] GC(11) Pause Young (Mixed) (G1 Evacuation Pause) 45M->10M(128M) 12.785ms
ZGC (Z Garbage Collector)
Flag to enable: -XX:+UseZGC
(Java 11+, production-ready in Java 15+)
Designed for extremely low pause times (sub-millisecond) regardless of heap size. Uses colored pointers and load barriers.
Best for:
- Applications requiring very low latency (<10ms pauses)
- Systems with very large heaps (terabytes)
- Real-time data processing systems
- User-facing applications with strict SLAs
GC Log Characteristics:
- Very short pause times
- Concurrent operations dominate the logs
- Detailed phase information with memory relocation data
- Multiple small pauses rather than fewer large ones
Example log entry:
[2023-05-15T14:22:33.156+0000] GC(10) Pause Mark Start 0.014ms
[2023-05-15T14:22:33.256+0000] GC(10) Concurrent Mark 33.285ms
[2023-05-15T14:22:33.356+0000] GC(10) Pause Mark End 0.008ms
Shenandoah
Flag to enable: -XX:+UseShenandoahGC
(Java 12+, backported to OpenJDK 8)
Similar to ZGC in goals, but with a different implementation approach. Uses Brooks pointers and a concurrent copying algorithm.
Best for:
- Applications needing consistent low pause times
- Systems with varying heap sizes
- Applications where responsiveness is critical
- Real-time systems with strict timing requirements
GC Log Characteristics:
- Very short pause times similar to ZGC
- Concurrent evacuation clearly shown in logs
- Detailed breakdown of each collection phase
- Information about memory regions copied concurrently
Example log entry:
[2023-05-15T14:22:33.156+0000] GC(10) Pause Init Mark 0.087ms
[2023-05-15T14:22:33.256+0000] GC(10) Concurrent Marking 94.219ms
[2023-05-15T14:22:33.356+0000] GC(10) Concurrent Evacuation 157.982ms
Reading Java GC Logs: The Basics
Let's look at some example log entries and break them down:
Java 8 GC Log Sample (Parallel GC)
2023-05-15T14:22:33.156+0000: 2.729: [GC (Allocation Failure) [PSYoungGen: 33280K->5088K(38400K)] 33280K->5096K(125952K), 0.0072848 secs] [Times: user=0.02 sys=0.00, real=0.01 secs]
What this tells us:
- Timestamp:
2023-05-15T14:22:33.156+0000
- Seconds since JVM start:
2.729
- Cause:
Allocation Failure
(couldn't allocate new object in eden space) - Young generation: Went from
33280K
to5088K
(total size38400K
) - Total heap: Went from
33280K
to5096K
(total size125952K
) - Collection time:
0.0072848 secs
(about 7ms)
Java 11+ GC Log Sample (G1 GC)
[2023-05-15T14:22:33.156+0000] GC(10) Pause Young (Normal) (G1 Evacuation Pause) 33M->5M(128M) 7.285ms
What this tells us:
- Timestamp:
[2023-05-15T14:22:33.156+0000]
- Collection number:
GC(10)
(the 10th collection) - Type:
Pause Young (Normal)
(a normal young generation collection) - Collector:
(G1 Evacuation Pause)
(G1 collector) - Memory change:
33M->5M(128M)
(from 33MB to 5MB, total heap 128MB) - Duration:
7.285ms
Common GC Log Patterns and What They Mean
Frequent Minor Collections
[2023-05-15T14:22:33.156+0000] GC(10) Pause Young (Normal) (G1 Evacuation Pause) 33M->5M(128M) 7.285ms
[2023-05-15T14:22:33.956+0000] GC(11) Pause Young (Normal) (G1 Evacuation Pause) 34M->6M(128M) 8.123ms
[2023-05-15T14:22:34.756+0000] GC(12) Pause Young (Normal) (G1 Evacuation Pause) 35M->7M(128M) 7.942ms
What it means: Your application is creating many short-lived objects. This isn't necessarily bad, but if the frequency is too high, consider increasing young generation size.
Long GC Pauses
[2023-05-15T14:25:13.156+0000] GC(45) Pause Full (System.gc()) 120M->60M(128M) 1325.285ms
What it means: Full GC operations taking over 1 second. This will cause noticeable application pauses. Check if explicit System.gc()
calls exist in your code, or consider using a low-pause collector like G1, ZGC, or Shenandoah.
Growing Old Generation
[2023-05-15T14:22:33.156+0000] GC(10) Pause Young (Normal) (G1 Evacuation Pause) 33M->15M(128M) 7.285ms
[2023-05-15T14:25:33.156+0000] GC(20) Pause Young (Normal) (G1 Evacuation Pause) 63M->45M(128M) 8.721ms
[2023-05-15T14:28:33.156+0000] GC(30) Pause Young (Normal) (G1 Evacuation Pause) 93M->75M(128M) 9.542ms
What it means: Objects are moving to the old generation but not being collected. This could indicate a memory leak or just long-lived objects. Monitor to see if it stabilizes or continues growing.
Troubleshooting Common GC Issues
High GC Overhead
When your application spends more than 10% of its time in garbage collection, it's a sign that something's wrong.
Symptoms in GC logs:
- Frequent collections
- Long pause times
- High proportion of "real" time spent in GC
Solutions:
- Increase heap size (
-Xmx
) - Check for memory leaks
- Consider using a different collector
- Profile to find excessive object creation
Memory Leaks
Symptoms in GC logs:
- Old generation size grows continuously
- Full GC events don't free much memory
- Eventually leads to OutOfMemoryError
Solutions:
- Take heap dumps with
jmap -dump:format=b,file=heap.bin <pid>
- Analyze with tools like Eclipse Memory Analyzer
- Fix the code that's holding references too long
Long Pause Times
Symptoms in GC logs:
- GC pauses over 200-300ms that impacts user experience
- Full GC events take seconds
Solutions:
- Switch to low-latency collector (G1, ZGC, Shenandoah)
- Increase heap size to reduce collection frequency
- Set maximum pause time goal (
-XX:MaxGCPauseMillis=200
)
GC Log Analysis Tools
Why stare at raw logs when tools can help?
Here's a comprehensive look at the tools that can turn your cryptic GC logs into actionable insights:
GCeasy
Type: Web-based service (free tier available)
Features:
- Upload and analyze GC logs from any Java version
- Interactive dashboards showing GC metrics
- Automatic detection of memory leaks and other problems
- Recommendations for JVM tuning parameters
- Comparison between different log files
- API available for automation
Best for:
- Quick analysis without installing software
- Getting specific JVM tuning recommendations
- One-off troubleshooting sessions
- Teams without dedicated monitoring infrastructure
Example insights:
- Memory leak probability score
- GC pause time distribution
- Heap usage before and after GC events
- Overall GC efficiency metrics
GCViewer
Type: Open-source desktop application
Features:
- Local analysis of GC log files
- Detailed visualization of GC events over time
- Supports multiple JVM vendors and versions
- Summary statistics of GC performance
- Comparison between different collectors
- Free and open-source
Best for:
- Developers wanting to analyze logs on their local machine
- Visual learners who prefer interactive charts
- Cross-collector comparisons
- Teams on a budget
Example insights:
- Pause time distribution charts
- Memory usage trends over time
- Frequency of different GC event types
- Throughput calculations
Censum
Type: Commercial desktop application
Features:
- Advanced analysis of complex GC patterns
- Predictive analytics for future GC behavior
- Correlation between GC events and other JVM metrics
- Automated root cause analysis
- Support for custom dashboards
- Enterprise-grade reports
Best for:
- Large enterprises with mission-critical Java applications
- Teams needing comprehensive GC analysis
- Organizations willing to pay for premium insights
- Production issues requiring deep investigation
Example insights:
- Multivariate correlation between GC triggers
- Anomaly detection in GC patterns
- Predictive modeling of future memory usage
- Impact of GC on application performance
jClarity (Acquired by Microsoft)
Type: Commercial, now part of Microsoft's Java tooling [Part of Microsoft's Azure services]
Features:
- AI-driven analysis of GC behavior
- Integration with cloud monitoring tools
- Automated recommendations
- Machine learning anomaly detection
- Real-time monitoring capabilities
- Integration with Azure services
Best for:
- Azure customers
- Real-time monitoring needs
- Teams already in the Microsoft ecosystem
- Enterprise environments
Example insights:
- Automated root cause detection
- Performance impact predictions
- Integration with system-wide metrics
- Correlation with application-level events
VisualVM
Type: Free, bundled with JDK (or downloadable)
Features:
- Real-time JVM monitoring including GC
- Heap dump analysis
- Thread monitoring alongside GC
- Profiling capabilities
- Plugin system for extensions
- Built-in to many JDK distributions
Best for:
- Developers who need an all-in-one tool
- Correlating GC with other JVM events
- Local application analysis
- Quick troubleshooting during development
Example insights:
- Real-time visualization of memory usage
- Collection event timing
- Thread states during GC
- Object allocation rates
Last9
Type: Commercial observability platform with native GC and JVM support
Features:
- Out-of-the-box JVM and GC visibility
- No need for custom parsing or dashboard setup
- Pre-built views for GC pauses, frequency, and memory reclamation
- Alerting on abnormal GC behavior
- Otel-native and supports structured logs
- Built-in correlation with SLOs and service health
Best for:
- Teams who want fast, ready-to-use GC insights
- JVM-heavy environments with uptime goals
- Engineers looking to reduce MTTR from GC-related slowdowns
- Observability setups using OpenTelemetry
Example insights:
- Identify GC spikes before they affect latency
- Track GC behavior by service, environment, or deployment
- Catch slow memory leaks with real-time trends
- Surface GC as a contributor to SLO violations
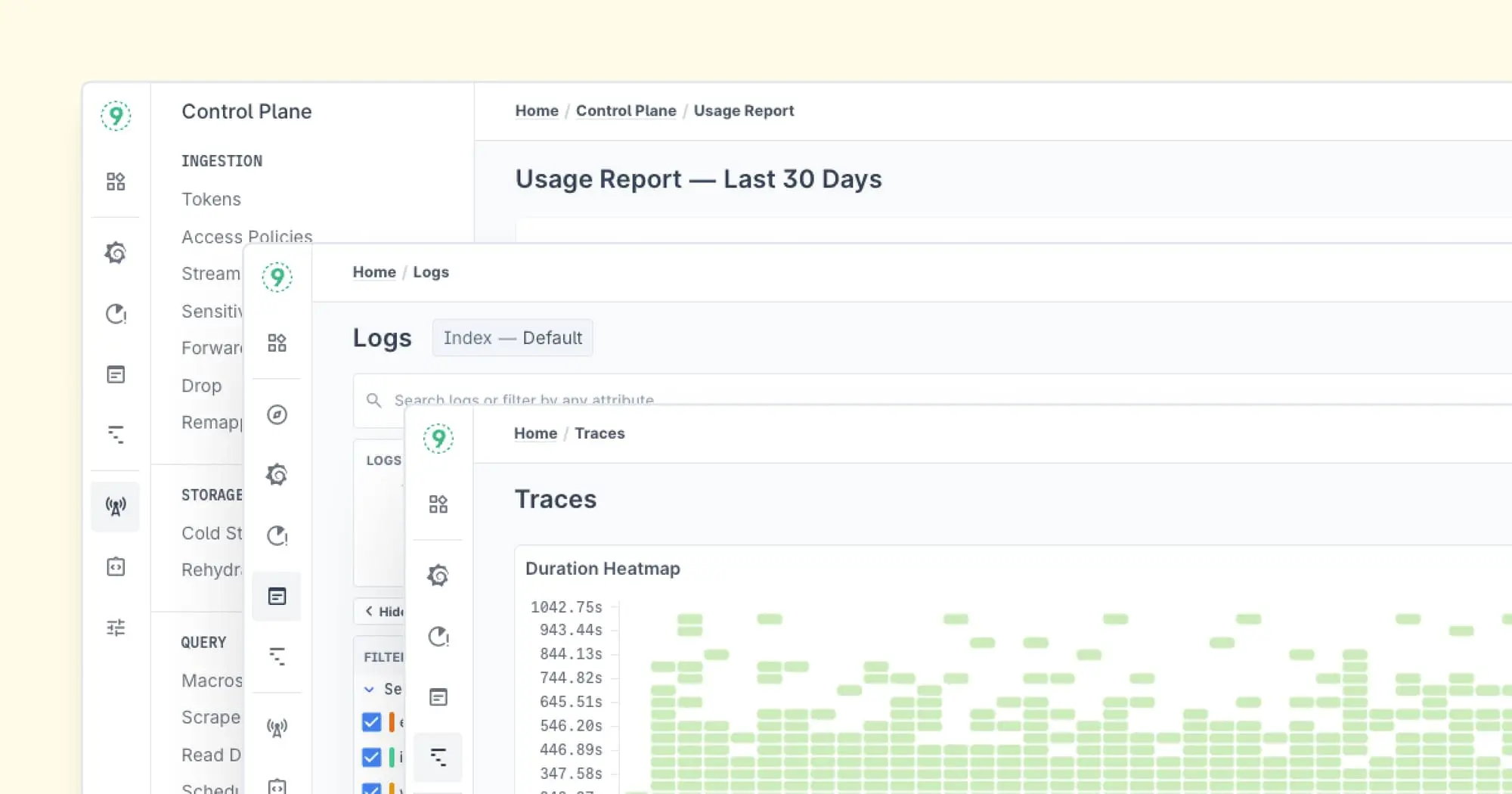
ELK Stack (Elasticsearch, Logstash, Kibana)
Type: Open-source log analysis platform
Features:
- Centralized log collection and analysis
- Custom dashboards for GC metrics
- Correlation with application logs
- Alerting on GC anomalies
- Scalable for large environments
- Historical trend analysis
Best for:
- Teams already using ELK for other logs
- Organizations needing centralized monitoring
- DevOps environments
- Custom dashboard requirements
Example insights:
- Long-term GC trends across application fleet
- Correlation between GC events and business metrics
- Custom alerting on specific GC patterns
- Infrastructure-wide memory usage analysis
Prometheus + Grafana
Type: Open-source monitoring stack
Features:
- Time-series metrics from JMX/JVM
- Real-time monitoring dashboards
- Alerting based on GC metrics
- Integration with other systems metrics
- Powerful query language
- Wide community support
Best for:
- Kubernetes environments
- Teams focused on observability
- Organizations using modern monitoring practices
- Correlating GC with system and application metrics
Example insights:
- GC duration and frequency over time
- Memory usage patterns
- Alerting on GC thresholds
- Correlation with application throughput
GCplot
Type: Web service with agent
Features:
- Continuous monitoring of GC behavior
- Analysis across JVM fleets
- Historical trend analysis
- Recommendations engine
- API access
- Support for containerized environments
Best for:
- Continuous monitoring in production
- Multi-JVM environments
- Containerized applications
- Teams wanting ongoing optimization
Example insights:
- Fleet-wide GC behavior
- Comparative analysis between application instances
- Long-term memory trend analysis
- Optimization recommendations
GC Log Best Practices for DevOps Teams
- Always enable GC logs in production – The performance impact is minimal, and the insights are invaluable.
- Set up log rotation – Don't let GC logs fill up your disk.
- Establish baselines – Know what "normal" looks like for your application.
- Automate analysis – Set up alerts for unusual GC patterns.
- Keep historical data – Store processed GC metrics to track changes over time.
- Correlate with application metrics – Connect GC events with response times and throughput.
- Document JVM settings – Know which flags are in use and why.
GC Settings Cheat Sheet
This expanded cheat sheet covers essential JVM flags for controlling garbage collection behavior:
Goal | JVM Flag | Value | Notes |
---|---|---|---|
Logging Settings | |||
Enable logs (Java 8) | -XX:+PrintGCDetails -Xloggc:/path/to/gc.log |
- | Basic GC logging |
Enable logs (Java 11+) | -Xlog:gc*=info:file=/path/to/gc.log |
- | Modern GC logging |
Log with timestamps | -XX:+PrintGCDateStamps |
- | Java 8 only |
Log application stops | -XX:+PrintGCApplicationStoppedTime |
- | Track all pauses |
Log causes | -XX:+PrintGCCause |
- | Show GC trigger reasons |
Log with time details | -Xlog:gc*:file=gc.log:time,uptimemillis |
- | Java 11+ format |
Collector Selection | |||
Use Serial collector | -XX:+UseSerialGC |
- | Single-threaded collector |
Use Parallel collector | -XX:+UseParallelGC |
- | Multi-threaded, throughput focused |
Use CMS collector | -XX:+UseConcMarkSweepGC |
- | Concurrent low-pause collector |
Use G1 collector | -XX:+UseG1GC |
- | Default in newer Java |
Use ZGC | -XX:+UseZGC |
- | Ultra-low pause collector (Java 11+) |
Use Shenandoah | -XX:+UseShenandoahGC |
- | Ultra-low pause alternative |
Memory Settings | |||
Set heap size | -Xms -Xmx |
2g, 8g | Initial and max heap |
Young gen size | -Xmn |
1g | Fixed young generation size |
Young gen ratio | -XX:NewRatio |
2 | Ratio of old/young gen (2 means 1/3 young) |
Survivor ratio | -XX:SurvivorRatio |
8 | Eden/Survivor space ratio |
MetaSpace size | -XX:MetaspaceSize -XX:MaxMetaspaceSize |
256m | For class metadata |
Performance Tuning | |||
Limit pause times | -XX:MaxGCPauseMillis |
200 | Target, not guarantee |
Set parallel threads | -XX:ParallelGCThreads |
8 | Number of threads for parallel phases |
Set concurrent threads | -XX:ConcGCThreads |
2 | Threads for concurrent phases |
G1 region size | -XX:G1HeapRegionSize |
1m | Size of G1 regions in bytes |
CMS init at usage | -XX:CMSInitiatingOccupancyFraction |
70 | Start CMS when old gen is 70% full |
G1 init at usage | -XX:InitiatingHeapOccupancyPercent |
45 | Start G1 concurrent cycle at 45% heap |
Advanced Tuning | |||
Adaptive sizing | -XX:+UseAdaptiveSizePolicy |
- | Let JVM adjust gen sizes |
Explicit GC concurrent | -XX:+ExplicitGCInvokesConcurrent |
- | Make System.gc() concurrent |
String deduplication | -XX:+UseStringDeduplication |
- | G1 only, reduces duplicate strings |
Aggressive heap | -XX:+AggressiveHeap |
- | Optimize for throughput on large memory |
TLAB size | -XX:TLABSize |
512k | Thread-local allocation buffer size |
Debugging | |||
Heap dump on OOM | -XX:+HeapDumpOnOutOfMemoryError |
- | Create heap dump file on OOM |
Heap dump path | -XX:HeapDumpPath |
/path/to/dumps | Where to write heap dumps |
Enable JMX monitoring | -Dcom.sun.management.jmxremote |
- | Enable remote monitoring |
Conclusion
Java GC logs might seem cryptic at first, but they're packed with insights that can help you keep your Java applications running smoothly. By understanding what the logs tell you and setting up proper monitoring, you can catch issues early, tune your JVM effectively, and avoid those dreaded 3 AM calls about application slowdowns.
FAQs
Why are my Java GC logs showing frequent Full GC events?
Frequent Full GC events typically indicate one of these issues:
- Your application might have a memory leak
- The heap size is too small for your workload
- You're creating too many long-lived objects
- There might be explicit
System.gc()
calls in your code or third-party libraries
Try increasing heap size, checking for memory leaks with a profiler, or adding -XX:+DisableExplicitGC
to ignore System.gc()
calls.
What's the difference between minor and major garbage collections?
Minor collections clean up the young generation (Eden and Survivor spaces) where most short-lived objects live. They're quick and happen frequently.
Major collections (or Full GC) clean up both young and old generations. They take longer because they process the entire heap and typically cause noticeable application pauses.
How can I tell if my application has a memory leak from GC logs?
Look for these patterns:
- Old generation usage that increases steadily even after Full GC events
- Full GC frequency that increases over time
- Longer and longer GC pause times
- Decreasing amount of reclaimed memory after each collection
If memory usage keeps growing without stabilizing, you likely have a leak.
What are "stop-the-world" pauses and how can I minimize them?
"Stop-the-world" pauses occur when the JVM temporarily halts all application threads to perform garbage collection safely. These pauses directly impact application responsiveness.
To minimize them:
- Use low-latency collectors like G1, ZGC, or Shenandoah
- Increase heap size to reduce collection frequency
- Tune generation sizes to match your application's memory profile
- Set an appropriate
-XX:MaxGCPauseMillis
target - Reduce object allocation rates in your code
How do I interpret GC efficiency from the logs?
GC efficiency can be measured by:
- Throughput: Percentage of time spent running application vs. garbage collection
- Pause times: Duration of individual GC events
- Memory reclamation: How much memory is freed relative to total heap
Good efficiency typically means:
- Less than 10% of time spent in GC
- Pause times appropriate for your application SLAs
- Memory usage that stabilizes over time
Should I be worried about "Allocation Failure" messages in GC logs?
No, "Allocation Failure" is a normal trigger for garbage collection. It simply means the JVM needed to allocate memory for a new object but couldn't find enough contiguous space in the current generation, so it initiated a garbage collection cycle.
This is part of normal JVM operation, not an error condition.
How do I choose the right garbage collector for my application?
Consider these factors:
- Response time requirements: For low latency, use G1, ZGC, or Shenandoah
- Throughput needs: For maximum throughput, use Parallel GC
- Heap size: For large heaps (>4GB), prefer G1, ZGC, or Shenandoah
- CPU resources: Low-latency collectors use more CPU
- Java version: Newer collectors require newer Java versions
Test different collectors with your actual workload and compare metrics.
What should I do when GC logs show "Concurrent Mode Failure"?
Concurrent Mode Failure occurs when the CMS collector can't finish its concurrent collection phase before the old generation fills up. This forces a stop-the-world full collection.
Solutions include:
- Increase heap size
- Start CMS collection earlier with
-XX:CMSInitiatingOccupancyFraction=<percent>
- Allocate more CPU resources to concurrent GC with
-XX:ConcGCThreads=<num>
- Consider switching to G1 collector
How much disk space do GC logs typically use?
GC log size depends on your application's GC activity, log detail level, and runtime duration. A busy application with detailed logging can generate several GB per day.
Always use log rotation to avoid filling up your disk:
- Java 8:
-XX:+UseGCLogFileRotation -XX:NumberOfGCLogFiles=10 -XX:GCLogFileSize=50M
- Java 11+:
-Xlog:gc*:file=gc.log::filecount=10,filesize=50M
Can GC logs help me determine the right heap size for my application?
Yes, GC logs are excellent for heap sizing. Look for:
- GC frequency: Too frequent minor GCs suggest increasing young generation
- Long full GCs: May indicate undersized heap
- Memory usage after Full GC: Shows your application's memory footprint
- Stable memory usage patterns: Help identify optimal heap size
Start with a larger heap and reduce it based on observed usage patterns.