As big data continues to grow, streaming aggregation has become a game-changer for handling and analyzing real-time data. Whether you’re a veteran data engineer, a DevOps expert, or just getting started with real-time analytics, mastering streaming aggregation is essential for building robust and scalable data pipelines.
In this blog, we'll explore the fundamentals of streaming aggregation, how it can transform your approach to real-time data processing, and best practices to manage and analyze large volumes of real-time information.
What is Streaming Aggregation?
Streaming aggregation is the process of continuously collecting, analyzing, and summarizing real-time data from multiple sources as it flows through a data pipeline.
Unlike batch processing, which operates on static datasets, streaming aggregation works on live, unbounded data streams, providing insights and actionable intelligence in real-time.
Key Components of Streaming Aggregation
- Data Ingestion: Capturing data from various sources in real-time
- Processing: Applying aggregation functions and transformations on the fly
- State Management: Maintaining aggregate states for continuous updates
- Output: Delivering aggregated data to downstream systems or applications
The Streaming Aggregation Workflow
To truly grasp streaming aggregation, let's break down the typical workflow:
- Data Collection: Instrumenting your systems to capture relevant metrics and events
- Ingestion: Streaming input data into your processing pipeline
- Processing: Applying aggregation functions and transformations
- State Management: Maintaining aggregate states for continuous updates
- Output: Delivering results to downstream systems or dashboards for observability
Streaming Aggregation vs. Batch Processing
While both streaming and batch processing have their place in data analytics, stream processing offers several advantages for real-time use cases:
Feature | Streaming Aggregation | Batch Processing |
---|---|---|
Latency | Low (milliseconds to seconds) | High (minutes to hours) |
Use Cases | Real-time monitoring, instant alerts | Historical analysis, daily reports |
Resource Usage | Consistent, predictable | Spiky, periodic |
Memory Usage | Requires efficient management | Can use more memory for large batches |
Flexibility | Adapts to changing data patterns | Fixed processing windows |
Complexity | Higher (needs robust error handling) | Lower (retries are easier) |
Streaming aggregation tackles high cardinality at its source—during data ingestion, before metrics are stored. This timing is the key differentiator compared to other approaches we've discussed in previous posts.
Technologies for Streaming Aggregation
Several technologies and frameworks support streaming aggregation. Let's explore some of the most popular ones:
Apache Spark Structured Streaming
Apache Spark with its Structured Streaming module is a powerhouse for streaming aggregation. It provides an SQL-like API for defining streaming computations, making it easier for developers familiar with SQL to transition to streaming workflows.
from pyspark.sql.functions import *
from pyspark.sql.types import *
# Create a streaming DataFrame
streamingDF = spark \
.readStream \
.format("kafka") \
.option("kafka.bootstrap.servers", "host1:port1,host2:port2") \
.option("subscribe", "topic1") \
.load()
# Define schema for the input data
schema = StructType([
StructField("user_id", StringType()),
StructField("event_type", StringType()),
StructField("timestamp", TimestampType())
])
# Parse the JSON data
parsedDF = streamingDF.select(
from_json(col("value").cast("string"), schema).alias("data")
).select("data.*")
# Apply streaming aggregation
aggregatedDF = parsedDF \
.withWatermark("timestamp", "10 minutes") \
.groupBy(
window(col("timestamp"), "1 hour"),
"user_id",
"event_type"
) \
.agg(count("*").alias("event_count"))
# Output the results
query = aggregatedDF \
.writeStream \
.outputMode("update") \
.format("console") \
.start()
query.awaitTermination()
This example demonstrates how to use Spark Structured Streaming to perform windowed aggregations on streaming data from Kafka. The aggregated data can be used for real-time analytics and observability.
For more detailed information and advanced configurations, refer to the Spark Structured Streaming documentation.
Databricks Delta Live Tables
Databricks extends Spark's capabilities with Delta Live Tables, which simplify the creation of reliable streaming data pipelines. They provide a declarative approach to defining your data transformations and aggregations.
CREATE STREAMING LIVE TABLE events_raw
COMMENT "Raw events from Kafka"
AS
SELECT *
FROM cloud_files("/path/to/events", "json");
CREATE STREAMING LIVE TABLE events_cleaned
COMMENT "Cleaned and validated events"
AS
SELECT
user_id,
event_type,
CAST(eventtime AS TIMESTAMP) AS event_time
FROM STREAM(LIVE.events_raw)
WHERE user_id IS NOT NULL
AND event_type IS NOT NULL;
CREATE STREAMING LIVE TABLE events_aggregated
COMMENT "Aggregated event counts by user and type"
AS
SELECT
user_id,
event_type,
count(*) AS event_count,
max(event_time) AS last_event_time
FROM STREAM(LIVE.events_cleaned)
GROUP BY user_id, event_type;
This SQL-like syntax defines a streaming pipeline that ingests raw events, cleans and validates the data, and then performs aggregations. Delta Live Tables handle the complexities of managing the streaming state and ensuring data consistency.
Key Concepts in Streaming Aggregation
Windowing
Windowing is a crucial concept in streaming aggregation, allowing you to group data into finite time buckets for processing. This is particularly useful for time series data. Common window types include:
- Tumbling Windows: Fixed-size, non-overlapping time intervals
- Sliding Windows: Fixed-size windows that slide by a specified interval
- Session Windows: Dynamic-size windows that capture periods of activity
Here's an example of implementing a sliding window in Spark Structured Streaming:
from pyspark.sql.functions import window
windowedCounts = parsedDF \
.groupBy(
window("timestamp", "1 hour", "15 minutes"),
"user_id"
) \
.count()
This code creates 1-hour windows that slide every 15 minutes, allowing for overlapping aggregations on time series data.
Watermarking
Watermarking is a technique used to handle late-arriving data in streaming systems. It defines how long to wait for late data before finalizing aggregation results for a specific time window.
from pyspark.sql.functions import *
windowedCounts = streamingDF \
.withWatermark("timestamp", "10 minutes") \
.groupBy(
window("timestamp", "1 hour", "5 minutes"),
"user_id"
) \
.count()
This Spark example sets a 10-minute watermark and performs a sliding window aggregation. The watermark ensures that the system won't wait indefinitely for late data, balancing between result completeness and timeliness.
State Management
Streaming aggregations often require maintaining a state across micro-batches. Frameworks like Spark use a state store to manage this efficiently, allowing for incremental updates to aggregations as new data arrives.
In Spark Structured Streaming, the state store is managed automatically for aggregation queries. For more complex stateful operations, you can use the mapGroupsWithState or flatMapGroupsWithState operations to explicitly manage the state.
from pyspark.sql.functions import *
from pyspark.sql.streaming import GroupState
def update_user_state(key, values, state):
if state.exists:
old_count = state.get
else:
old_count = 0
new_count = old_count + sum(value.count for value in values)
state.update(new_count)
return (key.user_id, new_count)
userCounts = parsedDF \
.groupBy("user_id") \
.applyInPandasWithState(
update_user_state,
outputStructType,
"append",
GroupStateTimeout.NoTimeout()
)
This example demonstrates how to use stateful processing to maintain a running count of events per user across multiple batches of streaming data.
Aggregation Functions in Streaming Contexts
Streaming aggregation supports various aggregation functions similar to those in batch processing:
- count(): Count the number of items
- sum(): Calculate the sum of values
- avg(): Compute the average of values
- min() and max(): Find minimum and maximum values
- approx_count_distinct(): Estimate the number of distinct items
These functions can be applied to time series data to generate real-time insights.
Use Cases for Streaming Aggregation
Streaming aggregation finds applications across various industries:
- Real-time Analytics: Continuously update dashboards with the latest metrics
- Anomaly Detection: Identify unusual patterns in real-time data streams
- IoT Monitoring: Aggregate sensor data for immediate insights
- Financial Trading: Process market data for split-second decision making
- User Behavior Analysis: Track and respond to user actions in real-time
- Network Monitoring: Aggregate network traffic data to detect security threats
- Supply Chain Management: Real-time inventory and logistics tracking
Best Practices for Implementing Streaming Aggregation
- Choose the Right Tools: Select technologies that match your use case and scale
- Design for Scalability: Ensure your pipeline can handle increasing data volumes
- Implement Error Handling: Prepare for network issues and data inconsistencies
- Monitor Performance: Keep an eye on throughput, latency, and resource usage
- Optimize Data Serialization: Use efficient formats like Avro or Parquet
- Handle Late Data: Implement watermarking to manage delayed events
- Test Thoroughly: Simulate various scenarios to ensure robustness
- Consider Data Quality: Implement data validation and cleansing steps
- Optimize State Management: Minimize state size and handle state cleanup
- Plan for Recovery: Implement checkpointing and recovery mechanisms
Challenges in Streaming Aggregation
While powerful, streaming aggregation comes with its own set of challenges:
- Out-of-Order Events: Handling data that arrives later than expected
- State Management: Efficiently maintaining and updating aggregate states
- Exactly-Once Processing: Ensuring each event is processed once and only once
- Scaling: Handling increasing data volumes and velocity
- Debugging: Troubleshooting issues in a continuously running system
- Data Skew: Managing uneven distribution of data across partitions
- Resource Management: Balancing CPU, memory usage, and network usage
- Schema Evolution: Handling changes in input data structure over time
Future Trends in Streaming Aggregation
As the field evolves, several trends are shaping the future of streaming aggregation:
- Edge Computing: Processing data closer to the source for reduced latency
- AI Integration: Using machine learning models for adaptive aggregations
- Serverless Architectures: Simplifying deployment and scaling of streaming pipelines
- Unified Batch and Streaming: Frameworks that seamlessly handle both paradigms
- Real-time Feature Stores: Integrating streaming aggregations with ML feature stores
- Streaming SQL Engines: Advanced SQL support for complex streaming queries
- Automated Optimization: AI-driven performance tuning for streaming pipelines
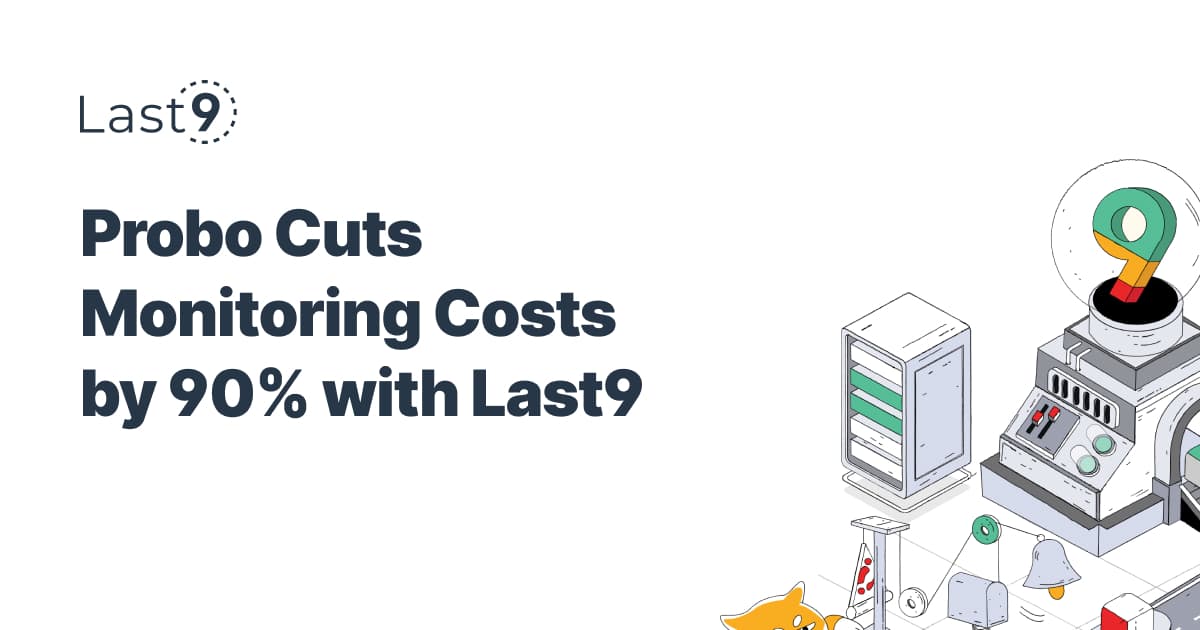
Conclusion:
Streaming aggregation is more than just a trend; it's a crucial shift in how we manage and analyze data. As you explore streaming aggregation, it's important to grasp both the core ideas and the practical steps involved.
When working with tools like Spark Structured Streaming or Databricks Delta Live Tables, prioritize efficient data processing, careful state management, and effective error handling. These principles will help you navigate the world of streaming data and make the most of the powerful capabilities it offers.
Join us on the SRE Discord community to share your experiences and thoughts on reliability, observability, and monitoring. We’d love to hear from you and connect!
FAQs related to Streaming Aggregation
Q: How does streaming aggregation handle high-cardinality data?
A: High-cardinality data can be challenging in streaming contexts. Techniques like approximate aggregations (e.g., HyperLogLog for distinct counts) and careful key management are often used to handle such scenarios efficiently.
Q: Can streaming aggregation work with historical data?
A: Yes, many streaming frameworks allow for combining historical (batch) data with live streams. This is often referred to as lambda architecture or kappa architecture, depending on the specific approach.
Q: How do you ensure data quality in streaming aggregations?
A: Implementing data validation at ingestion points, using schema registries, and setting up real-time data quality checks are common practices. Additionally, having a robust error handling and dead-letter queue system helps manage invalid data.
Q: What's the difference between complete, update, and append output modes in streaming?
A: These modes define how results are written:
- Complete: Entire result table is written every time
- Update: Only changed rows are written
- Append: Only new rows are written (used for aggregations on event time with watermarking)
Q: How do you optimize performance in streaming aggregations?
A: Key strategies include proper partitioning, efficient windowing, using appropriate watermarks, optimizing state management, and carefully tuning your streaming engine's configuration parameters. Monitoring memory usage and runtime performance is crucial for optimization.
Q: How does streaming aggregation contribute to system observability? A: Streaming aggregation provides real-time insights into system behavior, allowing for immediate detection of anomalies, performance issues, and other critical events. This visibility is essential for maintaining high levels of system observability.