When building robust, maintainable, and scalable Java applications, logging plays an essential role in debugging, monitoring, and ensuring smooth performance. Two of the most widely used logging frameworks in the Java ecosystem are SLF4J and Log4j.
While both serve similar purposes, they offer different approaches and features, making it important to understand their differences before making a choice.
In this guide, we will explore SLF4J and Log4j in-depth, compare their key features, and help you make an informed decision on which framework best suits your project needs.
What is SLF4J?
SLF4J (Simple Logging Facade for Java) is not a logging framework itself but rather a logging abstraction layer. It provides a simple interface that allows developers to plug in different logging frameworks like Log4j, Logback, or Java Util Logging (JUL).
SLF4J enables developers to change logging implementations without modifying the source code, offering greater flexibility and decoupling.
Key Features of SLF4J:
- Abstraction Layer: SLF4J serves as a façade, offering a unified API for logging. It doesn't provide the actual logging mechanism but allows integration with different logging frameworks.
- Compatibility: It supports a wide variety of popular logging frameworks (Log4j, Logback, etc.), making it versatile for different use cases.
- Lightweight: SLF4J is relatively lightweight because it only provides an abstraction layer and does not include any additional logging functionality.
- Simple API: SLF4J has a straightforward and easy-to-use API for logging messages.
What is Log4j?
Log4j is one of the oldest and most widely used logging frameworks for Java. It provides a comprehensive set of features for logging, including support for logging to various output destinations such as console, file, or remote servers.
Log4j comes with its own configuration options, such as XML, JSON, or properties-based configurations.
Key Features of Log4j:
- Rich Functionality: Log4j offers advanced logging features like log levels (TRACE, DEBUG, INFO, WARN, ERROR, FATAL), log filtering, and asynchronous logging.
- Highly Configurable: Log4j supports flexible configuration options for custom logging behavior, including filtering log messages based on severity or specific criteria.
- Output Options: Log4j supports logging to various appenders, such as files, console, databases, and even remote servers, making it adaptable to different logging needs.
- Performance: Log4j is designed for high-performance logging, especially in large-scale applications where efficiency matters.
SLF4J vs Log4j: Key Differences
Now that we've covered the basics of SLF4J and Log4j, let's dive into a comparison of their features to help you understand when to use each.
1. Abstraction vs. Implementation
- SLF4J: As an abstraction layer, SLF4J allows you to choose your logging framework (e.g., Log4j, Logback) at runtime without changing the code.
- Log4j: Log4j, on the other hand, is a full-fledged logging framework that provides both the interface and the implementation. You configure and use Log4j directly to handle logging within your application.
2. Flexibility and Adaptability
- SLF4J: The primary advantage of SLF4J is its flexibility. If your project requires switching between different logging frameworks, SLF4J offers the easiest way to do so. You can integrate various logging implementations without changing any logging statements in your code.
- Log4j: While Log4j offers robust functionality, it’s less flexible than SLF4J when it comes to switching frameworks. If you’re using Log4j, you’re tied to that particular framework unless you decide to refactor your code to use something else.
3. Logging Features
- SLF4J: SLF4J itself does not handle the actual logging functionality. It relies on the underlying logging framework (like Log4j) to provide features like log levels, file output, and custom formatting. Therefore, SLF4J’s capabilities are determined by the logging framework you choose.
- Log4j: Log4j provides a full suite of logging features out of the box. From log-level control to appender configuration, Log4j is a highly feature-rich solution that gives you complete control over your logging output.
4. Performance
- SLF4J: Since SLF4J is merely an abstraction, the performance depends on the logging framework it’s coupled with. SLF4J itself doesn’t add much overhead, but the actual performance depends on the underlying framework (such as Logback or Log4j).
- Log4j: Log4j is built with performance in mind, especially in high-load environments. It provides asynchronous logging capabilities and optimized logging for large-scale applications. If you need efficient and fast logging, Log4j is a solid choice.
5. Ease of Use
- SLF4J: SLF4J has a simple API that is easy to use for developers, and since it’s just an abstraction, the actual configuration and behavior depend on the logging implementation you select.
- Log4j: Log4j is also straightforward to use but comes with more configuration options, which can be both an advantage and a disadvantage. It’s great for users who want fine-grained control over their logging but may seem overly complex for simple use cases.
6. Configuration and Setup
- SLF4J: The configuration for SLF4J is done through the underlying logging implementation. For example, if you're using Logback or Log4j, you'll need to configure them independently.
- Log4j: Log4j has its own configuration format and is typically set up using XML or JSON configuration files. This gives you complete control over your logging behavior but can be more complex for beginners.
7. Integration and Compatibility
- SLF4J:
- Acts as a façade, allowing easy integration with multiple logging frameworks.
- Supports frameworks like Log4j, Logback, and Java Util Logging.
- Enables switching between logging systems without changing the application code.
- Ideal for projects that need flexibility in choosing or changing logging frameworks.
- Log4j:
- Works well within its own ecosystem, providing robust logging features.
- Doesn’t offer the same flexibility to switch frameworks easily.
- Typically requires refactoring to change to another logging system.
- Best for projects that don’t need frequent changes in the logging framework.
When to Use SLF4J and Log4j
When to Use SLF4J
- If you want a flexible, modular logging solution that can easily switch between different logging frameworks.
- If your project needs to support multiple logging implementations (e.g., Logback, Log4j, or even JUL) without modifying the codebase.
- If you’re building a library or framework and want to abstract the logging mechanism for users to choose their preferred framework.
When to Use Log4j
- If you need a full-featured logging framework with advanced capabilities such as log filtering, asynchronous logging, or custom log output configurations.
- If you don’t foresee the need to switch between logging frameworks and prefer a single logging implementation throughout the application.
- If performance is a top priority, Log4j’s optimizations and support for asynchronous logging make it well-suited for large-scale applications.
Use Cases and Examples
SLF4J and Log4j are widely used in real-world projects to handle logging needs across various types of applications.
Here are a few practical scenarios where these tools come in handy:
- Web Applications: In web applications, logging helps track user interactions, errors, and performance metrics. Using SLF4J with Log4j allows developers to create detailed logs that can be easily adjusted in terms of verbosity.
For instance, a Java-based Spring Boot application might use SLF4J as the API to log messages, with Log4j as the underlying implementation to handle file output and console logging.
Example: A Spring Boot application with Log4j might log user login attempts and errors like so:
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void login(String username) {
if (isValidUser(username)) {
logger.info("User {} successfully logged in.", username);
} else {
logger.error("Invalid login attempt for user: {}", username);
}
}
- Microservices Architecture: In microservices environments, where different services communicate via APIs, logging is crucial for tracing requests and diagnosing issues. SLF4J and Log4j can be used to implement logging across multiple services, ensuring consistent logging formats and centralized log management.
Example: In a microservices architecture using Spring Cloud, each service can log messages indicating the progress of requests between services:
logger.info("Sending request to payment service for order ID {}", orderId);
- Performance Monitoring: Logging isn’t just for errors—it’s also valuable for monitoring performance and operational insights. With Log4j, performance metrics such as response times, resource utilization, and API call frequencies can be logged to track system health.
Example: A service that processes heavy computation might log timing information:
long startTime = System.currentTimeMillis();
// Process something
long endTime = System.currentTimeMillis();
logger.info("Task completed in {} ms", (endTime - startTime));
- Distributed Systems: In a distributed system, tracing the flow of a request across different services is essential for debugging and monitoring. SLF4J and Log4j can be used to inject context (like request IDs) into log messages to make tracing easier.
Example: A system logging a request's journey through various services might look like:
MDC.put("requestId", requestId);
logger.info("Processing request {} in service {}", requestId, serviceName);
MDC.clear();
These are just a few examples of how SLF4J and Log4j are applied in real-world projects. The flexibility of these tools allows developers to tailor the logging system to meet the needs of the project, providing valuable insights and aiding in troubleshooting.
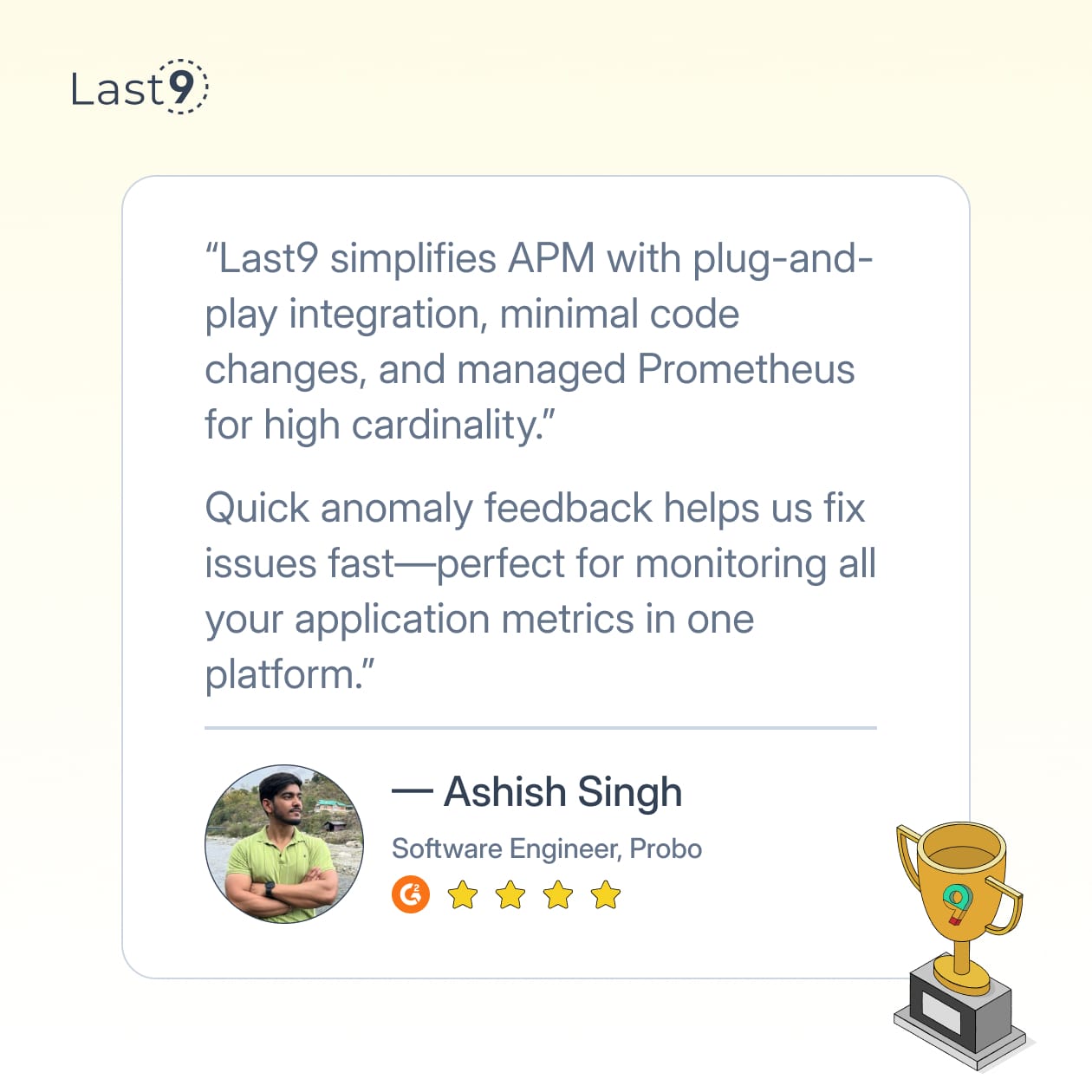
Conclusion:
Both SLF4J and Log4j have their place in Java application development. SLF4J offers a more flexible, modular approach, while Log4j provides rich features and high performance. Your decision will ultimately depend on your project's specific needs:
- Choose SLF4J if you need flexibility and the ability to switch between logging frameworks without major changes to your code.
- Opt for Log4j if you require a comprehensive, feature-rich logging framework with robust configuration options and high performance.
Whichever you choose, both frameworks can help you achieve effective logging, ensuring your application remains easy to maintain and monitor over time.
FAQs
What is the main difference between SLF4J and Log4j?
SLF4J is a logging abstraction layer that provides a unified API to integrate with different logging frameworks like Log4j, Logback, or Java Util Logging. Log4j, on the other hand, is a full-fledged logging framework that handles the actual logging functionality.
Which should I use, SLF4J or Log4j?
If you need flexibility and want to switch between logging frameworks without modifying your code, SLF4J is a better choice. If you need a powerful, feature-rich logging framework with detailed configuration options, Log4j is more suitable.
Can SLF4J work without Log4j?
Yes, SLF4J can work with other logging frameworks besides Log4j, like Logback or Java Util Logging. SLF4J simply acts as an abstraction layer, allowing you to choose your logging implementation at runtime.
Is Log4j better for performance?
Log4j is built with performance in mind, especially for high-load applications. It provides features like asynchronous logging to optimize performance. The performance of SLF4J depends on the logging framework it uses (e.g., Logback, Log4j), but SLF4J itself doesn't introduce significant overhead.
Can I switch logging frameworks easily with SLF4J?
Yes, one of SLF4J’s key advantages is its ability to switch between different logging frameworks without modifying the logging code. This makes it very adaptable to changes in the project.
Do I need to configure Log4j if I use SLF4J?
Yes, if you’re using Log4j as your logging implementation with SLF4J, you will need to configure Log4j separately. SLF4J itself doesn’t handle the logging configuration, so the actual logging behavior is determined by the underlying framework.
Which has more logging features, SLF4J or Log4j?
Log4j offers more advanced logging features out of the box, such as log filtering, multiple output appenders, and support for asynchronous logging. SLF4J only provides the API for logging; the features depend on the framework you choose to use with it.
Is it hard to set up Log4j?
Log4j can be more complex to set up compared to SLF4J because it has its own configuration files (typically XML or JSON). However, once configured, it provides comprehensive control over your logging setup.
Can SLF4J and Log4j be used together?
Yes, SLF4J and Log4j can be used together. SLF4J can act as the logging facade, while Log4j provides the actual implementation. This combination allows you to use Log4j’s advanced features while keeping your application decoupled from the logging framework.
Which one is easier to use, SLF4J or Log4j?
SLF4J has a simpler API and is easier to use, especially for developers who want an abstraction layer without dealing with complex configurations. Log4j, while straightforward for experienced users, offers more customization and control, which might make it seem more complex for simpler use cases.