When building modern .NET applications, logging is one of those things you don’t want to get wrong. Serilog steps in as a popular logging framework that has earned its spot as a go-to tool for developers. Why? Because it’s flexible, versatile, and does an awesome job of giving you clear insights into your app's behavior.
But what exactly is Serilog? It's a structured logging framework for .NET, meaning it helps you capture logs in a way that's easy to query and analyze, rather than just dumping lines of text into a log file. This makes troubleshooting and performance monitoring a whole lot easier.
In this blog, we’ll walk through how to set up and configure Serilog, how to handle errors like a pro, and why it’s the top choice for developers when it comes to logging. Let's get into it!
What is Serilog?
Serilog is a structured logging framework for .NET that helps you capture logs in a way that’s more useful than traditional text-based logs.
Instead of just outputting plain text, Serilog allows you to log data in a structured format, making it easier to query, analyze, and troubleshoot.
With Serilog, you can include rich context in your logs, like request IDs, user information, and performance metrics. This makes your logs more informative and valuable, giving you a better understanding of your application’s behavior.
In short, Serilog is all about making your logs more powerful and easier to work with—helping you to debug faster, monitor performance, and gain valuable insights into your application.
Why Use Serilog?
Now that we've covered the basics of configuring Serilog and handling errors, let's take a moment to talk about why Serilog is such a valuable tool in modern application development.
1. Structured Logging for Clarity
Traditional logging can often feel like reading a jumble of text that doesn’t tell you much. Serilog’s structured logging, however, gives you machine-readable log entries that are way easier to work with. This is super helpful for:
- Debugging: You can add specific details and context to your logs, making it much easier to spot what went wrong.
- Searchability: Forget manually scrolling through endless lines of text. With structured logs, you can search and find exactly what you need in no time.
- Analysis: You can send your log data to tools like Elasticsearch, where you can run queries and dig deeper into your app’s behavior.
2. Real-Time Logging
Serilog lets you log in in real-time, which is crucial for spotting issues as they pop up, especially in production. With real-time log streaming, you can keep an eye on your app’s performance, catch errors on the fly, and take action before problems escalate.
3. Integration with Multiple Outputs
One of the coolest things about Serilog is its ability to send logs to pretty much anywhere—whether that’s local files, databases, cloud services, or monitoring platforms. This flexibility makes it easy to integrate Serilog into whatever system you’ve got, no matter how complex.
4. Rich Contextual Information
Serilog doesn’t just log timestamps and messages. It logs context—like request IDs, user data, and performance metrics—that help you understand the why behind an issue. This makes it easier to figure out exactly what happened and how to fix it.
5. Scalable and Flexible
Whether you're running a small app or managing a large, distributed system, Serilog scales effortlessly. It can handle a huge volume of logs without bogging down your system, making it perfect for apps of all sizes.
6. Customizable and Extensible
If you need Serilog to do something a little more specific, no problem. It’s highly customizable. You can write your own sinks or extend its features to suit your needs, so it always works the way you want it to.
So, if you’re looking for a logging framework that’s easy to use, flexible, and powerful, Serilog has you covered.
How to Configure Serilog
Configuring Serilog in your .NET application is straightforward, but doing it right can make a difference. Here’s a simple guide to get you started:
1. Install Serilog Packages
The first step is to install the necessary Serilog packages. These are available via NuGet, and you’ll usually install the core Serilog package along with any "sinks" (outputs) for where you want your logs to go.
To install Serilog and the console and file sinks, run these commands:
dotnet add package Serilog
dotnet add package Serilog.Sinks.Console
dotnet add package Serilog.Sinks.File
You can also install additional sinks if you plan to send logs to other destinations, such as databases, cloud services, or monitoring platforms.
2. Set Up Serilog in Code
Once the packages are installed, you’ll need to set up Serilog in your Program.cs
or Startup.cs
file.
Here’s a simple example that configures Serilog to log into both the console and a file:
using Serilog;
class Program
{
static void Main(string[] args)
{
// Configure Serilog to log to both the console and a file
Log.Logger = new LoggerConfiguration()
.WriteTo.Console()
.WriteTo.File("logs/myapp.txt", rollingInterval: RollingInterval.Day)
.CreateLogger();
// Use Serilog for logging
Log.Information("Application has started");
// Always close the logger at the end of the application
Log.CloseAndFlush();
}
}
In this example, Serilog is set up to log messages to the console, and a file called myapp.txt
that rolls over every day. The WriteTo.Console()
method ensures that logs are printed to the console, while WriteTo.File()
writes logs to the file.
3. Customize Log Output
You can also customize the format of your logs. For example, if you want your logs to be in JSON format for better machine readability, you can adjust the configuration like this:
Log.Logger = new LoggerConfiguration()
.WriteTo.Console(outputTemplate: "{Timestamp:HH:mm:ss} [{Level}] {Message}{NewLine}{Exception}")
.WriteTo.File("logs/myapp.json", outputTemplate: "{Timestamp:yyyy-MM-dd HH:mm:ss} [{Level}] {Message}{NewLine}{Exception}")
.CreateLogger();
This configuration customizes the time format and the log layout for both the console and the file. You can tailor the format to fit your needs, whether it's more human-readable or optimized for machine processing.
That’s it! With these steps, you’ll have Serilog up and running, logging both to the console and a file, with the option to customize the format however you like.
Handling Errors in Serilog
Serilog provides a fantastic way to handle and log errors in a meaningful way. Proper error handling is key to debugging and maintaining your app, especially in production, and Serilog ensures you won’t miss any crucial details.
Here’s how you can set up Serilog to handle errors effectively:
1. Logging Exceptions
Serilog makes it easy to capture detailed information about exceptions, including stack traces. To log exceptions, simply use the Log.Error
method:
try
{
// Some code that might throw an exception
throw new Exception("Something went wrong!");
}
catch (Exception ex)
{
Log.Error(ex, "An error occurred while processing the request.");
}
In this example, Serilog logs the exception along with a custom message. The exception details, including the message and stack trace, are automatically captured, making it much easier to trace errors when reviewing your logs.
2. Structured Error Logging
One of Serilog’s strengths is its ability to provide structured logging. Instead of just outputting plain text, you can log additional context to give you more insight into the circumstances of an error.
For example, if an exception occurs during user authentication, you might want to log the user’s ID or IP address:
try
{
// Some code that might throw an exception
}
catch (Exception ex)
{
Log.Error(ex, "Error processing user authentication for UserId: {UserId}", userId);
}
Here, {UserId}
is a template that gets replaced with the actual user ID, and because it’s structured, you can easily query and filter your logs to find issues related to specific users or other contextual data.
3. Handling Unhandled Exceptions
For critical errors like unhandled exceptions that might crash your application, Serilog can be set up to catch them globally.
In an ASP.NET Core app, you can configure Serilog in the Program.cs
file to log unhandled exceptions:
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureAppConfiguration((context, config) =>
{
// Other configuration
})
.ConfigureLogging((context, logging) =>
{
logging.ClearProviders(); // Clear default logging providers
logging.AddSerilog(); // Add Serilog as the logging provider
})
.ConfigureServices((hostContext, services) =>
{
services.AddHostedService<Worker>();
});
This configuration ensures that any unhandled exceptions in your application are caught and logged by Serilog, offering a robust error-handling solution to help you stay on top of any critical issues.
Serilog Community Support
Fortunately, the Serilog community is active and supportive, providing multiple ways to get help, share experiences, and find solutions.
Here’s where you can turn for support:
1. Serilog Documentation:
Before diving into forums or community discussions, the Serilog documentation should be your first stop. It’s comprehensive, regularly updated, and provides detailed information on configuration, sinks, and advanced usage.
Key Features of the Documentation:
- Getting Started Guides: Step-by-step instructions to integrate Serilog into your project.
- Configuration Examples: Learn how to set up Serilog for different log outputs (console, files, databases, etc.).
- Error Handling and Troubleshooting: Solutions to common issues.
- API Reference: In-depth details about Serilog’s methods, properties, and classes.
You can access the official Serilog documentation here.
2. GitHub Issues:
As an open-source project, Serilog’s community interacts heavily with GitHub. If you’ve encountered a bug or unexpected behavior, the GitHub repository is where you can report it.
Benefits of GitHub Issues:
- Bug Reporting: Report bugs or unexpected behaviors, and provide as much detail as possible.
- Feature Requests: Suggest improvements or new features.
- Solutions from the Community: Often, community members offer workarounds or fixes that can help resolve your issue.
Visit the Serilog GitHub repository to report issues or browse existing discussions.
3. Serilog Community Forums:
The Serilog community forums are another great resource for help and discussion. Here, you can ask questions, share experiences, and learn from others who have faced similar challenges.
Why Use the Serilog Forums:
- Active Discussions: Engage with other developers and find solutions to common questions.
- Problem-Solving: If you're facing a unique issue, chances are someone else has encountered it.
- Sharing Best Practices: Learn tips on how to solve performance issues or customize your logging setup.
4. Stack Overflow:
For more general questions, Stack Overflow is an excellent platform to tap into a broader pool of knowledge.
How to Use Stack Overflow:
- Ask Questions: Post clear, detailed questions and tag them with
serilog
. - Find Existing Solutions: Search for answers to similar problems before posting new ones.
- Community Expertise: Stack Overflow has a large community of .NET and Serilog experts who regularly contribute answers.
Search for Serilog-related questions on Stack Overflow.
5. Serilog Slack or Gitter:
If you prefer real-time support or want to engage with others while you're working, joining the Serilog Slack or Gitter room is a great option.
Why Join Slack or Gitter:
- Instant Help: Get immediate answers from the community.
- Talk with Developers: Discuss everything from basic setup to advanced configurations.
- Community Events: Stay updated on live sessions and events.
You can join Serilog’s Slack channel or Gitter room through links provided on the Serilog website or GitHub.
6. Blog Posts and Tutorials:
In addition to the official documentation, there’s a wealth of blog posts, tutorials, and videos created by the community. These resources go beyond the basics and dive into advanced topics like configuration for different environments, performance optimization, and best practices.
Great places to explore include:
- Medium: Developers share Serilog configuration tips, performance insights, and more.
- Dev.to: A platform for developers to share posts about Serilog.
- YouTube: Video tutorials offer hands-on guidance and visual demonstrations.
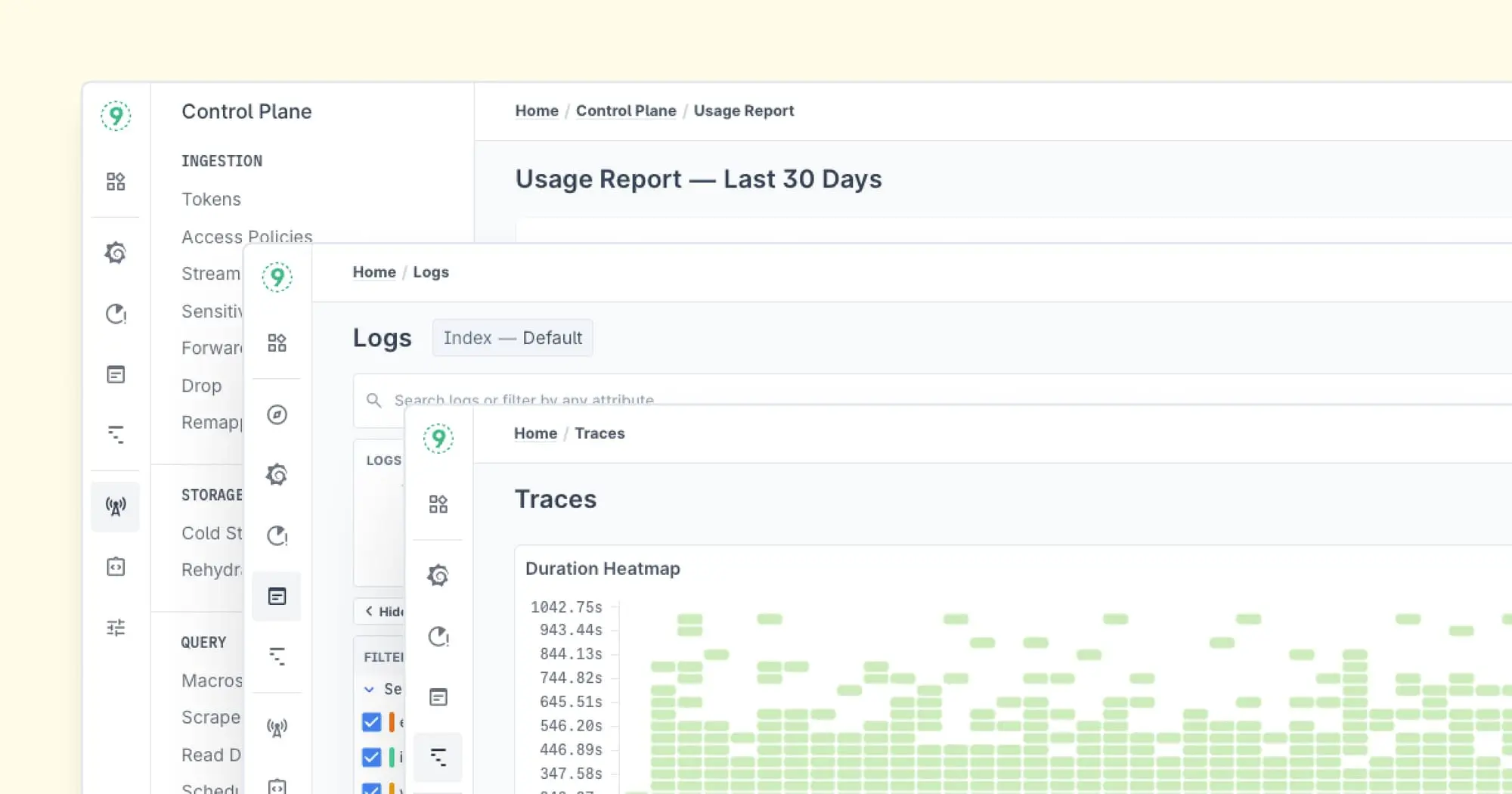
7. Serilog Community Events and Meetups
The Serilog team and community frequently host meetups, webinars, and events to dive deeper into Serilog's features, best practices, and new releases. These events are excellent for networking with other developers, learning from experts, and staying up to date with Serilog developments.
Check out the Serilog events page or platforms like Meetup.com for local Serilog or . NET-related meetups.
Conclusion
Integrating Serilog into your application brings robust logging capabilities that are key to building reliable and maintainable software. It not only makes it easier to track and resolve issues, but it also helps you get the most out of your application's log data.
Start using Serilog today to unlock the full potential of your application's logging system!
FAQs
What is Serilog?
Serilog is a modern logging framework for .NET applications that supports structured logging. It helps developers capture meaningful log data, making it easier to debug, monitor, and analyze application behavior.
How do I set up Serilog in my application?
To set up Serilog, first, install the necessary NuGet packages like Serilog
and Serilog.Sinks.Console
. Then, configure Serilog in your Program.cs
or Startup.cs
by specifying the sinks (like console, file, etc.) where you want to output logs.
Can I customize the log format in Serilog?
Yes, Serilog allows you to customize the log format. You can change the output template for different sinks, and you can also output logs in structured formats like JSON for better machine readability.
How does Serilog handle errors?
Serilog handles errors by logging exceptions along with detailed information like stack traces. You can use Log.Error()
to log exceptions with custom messages and structured data to help you trace issues effectively.
Can I use Serilog in production environments?
Absolutely! Serilog is well-suited for production environments. With real-time logging, error tracking, and the ability to send logs to various destinations like cloud services or databases, Serilog helps you monitor your app's health in real time.
What are sinks in Serilog?
Sinks are the destinations where logs are sent. Serilog supports a variety of sinks, including console, file, database, and cloud services. You can configure multiple sinks based on your needs.
How does structured logging improve error tracing?
Structured logging helps by attaching context and additional data to log entries, like user IDs or request details. This makes it easier to query and filter logs, allowing you to pinpoint the root cause of issues faster.
Is Serilog suitable for large applications?
Yes, Serilog is scalable and performs well even with large applications. It’s designed to handle high-volume logging without affecting the performance of your application.
Where can I get support for Serilog?
If you need help, Serilog has a vibrant community and several support channels, including official documentation, GitHub Issues, community forums, and real-time discussions on platforms like Discord and Slack.