When it comes to Rails development, logging isn’t just about tracking what’s happening in your app. It’s a lifeline for developers, helping you catch bugs, monitor performance, and keep your code running smoothly in production.
In this guide, we’ll cover everything from the basics to some cool tips that are often overlooked. Whether you’re just starting with Rails or you’ve been around the block a few times, getting the hang of Rails logger will save you a ton of headaches.
What is a Rails Logger?
Rails Logger is the go-to tool for tracking what's going on inside your Ruby on Rails app. It logs messages to help developers debug issues and keep an eye on how things are running.
Whether it's capturing tiny details or catching critical errors, Rails Logger covers it all. By default, Rails comes with a straightforward yet powerful logger that can log messages at different levels—like debug
, info
, warn
, error
, and fatal
—depending on the severity of the issue.
You’ll find the logger available in every Rails app Rails.logger
, making it easy to log messages from anywhere in your app—whether it's in your controllers, models, or other parts of your code.
How to Set Up Rails Logger: Step-by-Step
Setting up the Rails Logger is pretty simple, but there are a few tweaks you can make to customize how it behaves. Here’s a step-by-step guide:
1. Default Logger Setup
By default, Rails logs messages in two main environments:
log/development.log
during developmentlog/production.log
for production
You don’t need to do anything special to get started—Rails takes care of this for you. However, if you want to fine-tune things, you can easily adjust settings in your config/application.rb
or specific environment configuration files.
2. Log Level Customization
Rails Logger lets you filter messages by their severity level. For instance, if you want to only log warnings, errors, or fatal messages (and not all the debug messages cluttering your logs), you can set the log level. Here’s how to do it:
Open your config/environments/development.rb
(or production.rb
if you’re working in production) and add the following line to set the log level:
config.log_level = :warn
This means only warn
, error
, and fatal
level messages will show up in your logs. It helps keep your log files clean and relevant, especially when you're troubleshooting or monitoring performance.
3. Adding Log Tags for More Context
Sometimes, it’s useful to add extra context to your logs, such as the user ID, request ID, or any other custom data. Rails allows you to add "tags" to your log messages so that each log line comes with more identifying information.
To add log tags, you’ll also modify the config/environments/development.rb
(or production) file. Here’s an example of how to add a request UUID and subdomain as tags:
config.log_tags = [:subdomain, :uuid]
With this, every log line will include the subdomain
and uuid
, making it much easier to trace specific requests and correlate log entries across requests.
4. Testing Your Configuration
Once you’ve set up the log level and tags, it’s time to test it. You can do so by triggering different log messages in your application. For example, in your controller or model, you can log a message like this:
Rails.logger.debug "This is a debug message"
Rails.logger.info "This is an info message"
Rails.logger.warn "This is a warning message"
Rails.logger.error "This is an error message"
Check your development.log
or production.log
to see how your messages are logged. You should see that based on your log level setting, only messages at or above the :warn
level is captured.
What are Rails Logger Levels?
Rails Logger provides different log levels, each designed for a specific purpose. Knowing when to use each level can help you avoid drowning in log noise while still capturing the right info. Here's a breakdown of the available levels:
1. DEBUG
This is the most detailed log level. It captures everything—useful when you're debugging or tracking down issues during development. However, it can generate a lot of log noise, so it’s best to use it sparingly in production.
2. INFO
This level provides general information about the app's behavior. For example, it might log when a request is received or when a process starts. It helps track routine operations without overloading you with excessive detail.
3. WARN
A warning indicates something that’s not critical but may need attention soon. It’s great for flagging potential issues, like deprecated methods or slow database queries, that don’t immediately break the app but could cause problems down the road.
4. ERROR
When something goes wrong, this level captures it. Errors are often used for catching exceptions and logging things like failed database queries or invalid user inputs. If the app is still running, an error level log will give you a heads-up.
5. FATAL
This is the most severe log level. It usually marks a critical error that causes the application to crash. These messages require immediate attention, as they often indicate something’s gone very wrong and your app may not recover.
Production vs. Development
- In production: You typically set the log level to
:info
or:warn
to avoid filling the logs with too much detail while still catching important events. - In development: The
:debug
level is often used, as it gives more granular insight into app behavior, which is useful when you're building or debugging.
How to Integrate Logger in Rails Applications
With Rails Logger, you can capture critical events, exceptions, and informational messages that provide insight into your app’s behavior.
In this section, we'll guide you through the process of setting up and integrating the Rails Logger to ensure smooth logging functionality.
1. Rails Logger Basics
Rails comes with a built-in logger that is preconfigured to log messages across different environments such as development, production, and test. The Rails Logger automatically handles different log levels like debug
, info
, warn
, error
, and fatal
, ensuring relevant logs are captured for monitoring.
Default Log Locations:
log/development.log
for the development environment.log/production.log
for the production environment.log/test.log
for the test environment.
These logs are automatically generated, and no extra configuration is needed to get started.
2. Configuring the Logger
While Rails comes with a default logger, you can modify its configuration to meet your application's specific needs. This includes adjusting the log level, setting custom log locations, or tweaking the format of logs.
Setting the Log Level
The log level determines the minimum severity of messages to be captured by the logger. By default, Rails uses :debug
in development and :info
in production. To change the log level in production, for example, you would adjust config/environments/production.rb
:
config.log_level = :warn
This ensures that only warning, error, and fatal messages are logged in production.
Custom Log File Location
To change where logs are saved, modify config.logger
in your environment file:
config.logger = Logger.new('/path/to/production.log')
To log both to a file and the console:
config.logger = Logger.new(STDOUT)
config.logger = Logger.new('/path/to/production.log', 'daily')
3. Using Logger in Code
Once configured, you can use the Rails Logger throughout your application to capture messages of different severity levels. For example:
Rails.logger.debug "This is a debug message"
Rails.logger.info "This is an info message"
Rails.logger.warn "This is a warning message"
Rails.logger.error "This is an error message"
Rails.logger.fatal "This is a fatal message"
These messages will be logged to the appropriate log file (e.g., development.log
or production.log
) based on the environment.
Logging Exceptions
To log exceptions, use the begin-rescue
block along with Rails.logger
:
begin
risky_method
rescue => e
Rails.logger.error "Error occurred: #{e.message}"
end
4. Customizing Log Format and Tags
If you want to personalize the log output, Rails allows you to modify the log format and add tags to include contextual information.
Custom Log Formatter
You can create a custom log format by subclassing Logger::Formatter
and overriding the call
method. For example, to include the timestamp and log level:
class CustomLogFormatter < Logger::Formatter
def call(severity, time, progname, msg)
"#{time.utc.iso8601} [#{severity}] #{msg}\n"
end
end
In config/application.rb
, set the custom formatted:
config.logger.formatter = CustomLogFormatter.new
Adding Tags to Logs
You can add useful context, like the request ID or user ID, to every log message. For example:
config.log_tags = [:request_id]
This automatically includes the request ID in each log entry. You can also add tags dynamically using Rails.logger.tagged
:
Rails.logger.tagged("User ID: #{user.id}") do
Rails.logger.info "User successfully logged in"
end
5. Log Rotation and Archiving
To prevent logs from becoming too large, you can set up log rotation, which will automatically rotate log files and keep only the most recent ones.
Log Rotation
Configure log rotation based on file size in your environment file:
config.logger = Logger.new('/path/to/logs/production.log', 10, 1024 * 1024 * 5)
This configuration rotates the log file once it reaches 5 MB and keeps the 10 most recent log files.
Archiving Old Logs
You can also archive old logs to a separate folder or compress them to save space. Tools like logrotate
(on Linux systems) can automate this process.
6. Integrating External Loggers
Sometimes, logging into a file may not be enough, especially in distributed systems. In these cases, you might want to send logs to an external service like Last9, , Datadog, or Splunk for centralized logging and analysis.
To integrate an external logger, replace the default Rails logger with one that connects to the service. For example, to use Last9:
config.logger = RemoteSyslogLogger.new('logs.last9.io', 12345)
This sends all log messages directly to Last9 for centralized analysis.
Advanced Rails Logging Techniques
Rails Logger basics are simple, but advanced techniques can enhance your logging. From integrating external services to adding context to messages, these tips help you make the most of your logs.
Let's explore some of these powerful features:
1. Logging to External Services
When your application grows, relying only on local log files can feel limiting. You may need a more scalable solution, especially if you want to aggregate logs from multiple environments.
Integrating Rails Logger with external log management services like Loggly, Last9, or Datadog is a great way to monitor your app more effectively.
For example, to send logs to Last9, you can configure it like this:
config.logger = RemoteSyslogLogger.new('logs.last9.io', 12345)
With external services, you get real-time insights into your app's health, making it easier to track issues and monitor performance across different environments.
2. Tagged Logging for More Context
Sometimes you need more context in your logs to make sense of what's going on—especially in high-traffic apps.
Tagged logging allows you to add custom tags (like user IDs or request IDs) to each log message, which makes it easier to track the flow of requests and pinpoint problems.
Here’s how to use tagged logging in Rails:
Rails.logger.tagged("User: #{user.id}") do
Rails.logger.info "User logged in"
end
This adds a "User: #{user.id}" tag to your log entry, making it easier to associate log messages with specific users or sessions. It’s especially helpful when debugging issues that involve different users or complex workflows.
3. Conditional Logging in Production
In production, you often don’t want to log everything, but you still need to capture certain actions that could impact user experience or system performance.
You can conditionally log events based on specific criteria, such as user roles or traffic conditions.
For example, you can log a critical action only for admin users in production:
if Rails.env.production? && user.admin?
Rails.logger.error "Admin user triggered a critical action"
end
This ensures that only important logs are captured, preventing unnecessary log bloat in your production environment while still keeping track of vital actions.
4. Custom Log Formatter
Sometimes the default log format just doesn’t cut it. Fortunately, Rails allows you to create custom log formatters so that you can output logs in a format that suits your needs, whether it’s for better readability or integration with other systems.
Here’s an example of a custom log formatter:
class CustomLogFormatter < Logger::Formatter
def call(severity, time, progname, msg)
"#{time.utc.iso8601} #{severity} #{msg}\n"
end
end
Rails.logger.formatter = CustomLogFormatter.new
This custom formatter will output logs in the format ISO8601 time
, severity level
, and the actual message. It makes the logs cleaner and potentially easier to parse for analysis or external monitoring tools.
How to Customize Log Output in Rails Logger
Customizing Rails logs helps improve readability and control, especially for specific application needs. From changing formats to directing logs to external services, Rails Logger is flexible enough to adapt to various logging preferences.
Here’s how you can customize your log output.
1. Customizing the Log Format
The default log format in Rails might not fit your needs. You can define a custom log format by creating a custom formatter.
How to Customize: Create a custom formatter by subclassing Logger::Formatter
and overriding its call
method.
Example:
class CustomLogFormatter < Logger::Formatter
def call(severity, time, progname, msg)
"#{time.utc.iso8601} [#{severity}] #{msg}\n"
end
end
Apply the formatter in config/application.rb
:
config.logger.formatter = CustomLogFormatter.new
This will change the log format across your Rails application to include ISO 8601 timestamps, severity, and the message.
2. Changing the Log Location
Rails logs by default to files like log/development.log
and log/production.log
. You can customize where your logs go, whether it's to different files or external services.
How to Customize: Modify the logger configuration in your environment files (e.g., config/environments/production.rb
).
Example: Logging to a custom file with daily rotation:
config.logger = Logger.new('/path/to/custom_log_file.log', 'daily')
To log to both a file and the terminal (useful for Docker or containerized apps):
config.logger = Logger.new(STDOUT)
config.logger = Logger.new('/path/to/custom_log_file.log', 'daily')
3. Adding Custom Tags to Logs
Tags can help contextualize your logs, making it easier to trace logs related to specific users, requests, or actions.
How to Customize: Use config.log_tags
to add tags to each log entry.
Example: Tagging logs with request ID and UUID:
config.log_tags = [:request_id, :uuid]
Add custom tags in specific parts of your app with Rails.logger.tagged
:
Rails.logger.tagged("User ID: #{user.id}") do
Rails.logger.info "User successfully logged in"
end
4. Using Log Rotation
Log files can grow large over time, which may slow down your app or consume too much disk space. Rails supports log rotation to manage large files.
How to Customize: Configure log rotation based on file size or time intervals in your environment files.
Example: Rotate logs after they exceed 5 MB and keep 10 recent log files:
config.logger = Logger.new('/path/to/logs/production.log', 10, 1024 * 1024 * 5)
5. Logging to External Services
In distributed systems or microservices, local log files might not be enough. You can log in to external services like Last9, Papertrail, or Datadog for better aggregation and analysis.
How to Customize: Replace the default logger with one that integrates with an external service.
For example, to log directly to Last9, you can set it up like this:
config.logger = RemoteSyslogLogger.new('logs.last9.io', 12345)
6. Using Structured Logging with JSON
For advanced logging needs, structured logging (e.g., in JSON format) is useful for easier parsing and integration with log aggregation systems like ELK (Elasticsearch, Logstash, Kibana) or Splunk.
How to Customize: Create a custom formatter that outputs logs as JSON.
Example:
class JsonLogFormatter < Logger::Formatter
def call(severity, time, progname, msg)
{ time: time.utc.iso8601, severity: severity, message: msg }.to_json + "\n"
end
end
Apply this formatter in config/application.rb
:
config.logger.formatter = JsonLogFormatter.new
This outputs structured logs as JSON, making it easier to parse and analyze.
Debugging with Rails Logger: Best Practices
Effective logging is key to debugging, but it’s important to avoid overwhelming your logs. Here are some best practices:
1. Use Debug Sparingly in Production
In production, stick to info
, warn
, error
, and fatal
logs. Excessive debug
logs can clutter production logs. Reserve detailed logs for troubleshooting when necessary.
2. Capture Exceptions Effectively
Rails automatically logs uncaught exceptions, but you can explicitly log errors in your code like this:
begin
# Code that might raise an exception
rescue => e
Rails.logger.error "An error occurred: #{e.message}"
end
3. Log User Actions
For user-focused apps, log user actions (like logins or purchases) to track behavior and improve troubleshooting.
4. Avoid Logging Sensitive Data
Never log sensitive info like passwords or credit card numbers. Use Rails' built-in filtering to block sensitive parameters:
config.filter_parameters += [:password, :credit_card_number]
Performance Considerations: Keeping Logs Efficient
Excessive logging can impact performance. Here’s how to keep your logs efficient:
1. Log Only What’s Necessary
Focus on logging errors, warnings, and significant events—don’t log every action.
2. Rotate Log Files
Use log rotation (e.g., logrotate
on Unix systems) to prevent log files from growing too large.
3. Asynchronous Logging
Consider using background jobs to handle non-critical logs, freeing up resources for more important tasks.
What’s the Impact of Logging on Performance?
While logging is crucial for debugging and monitoring, it can negatively impact your app's performance, especially in high-traffic environments. Let’s break down the key performance issues caused by logging and strategies to mitigate them.
1. Increased I/O Operations
Writing logs to disk involves I/O operations, which can slow down your app, particularly with frequent logging or large datasets.
How to Mitigate:
- Asynchronous Logging: Use background jobs (e.g., ActiveJob) to process log entries without blocking the app's main operations.
- Log Rotation: Implement log rotation to prevent log files from becoming too large, ensuring smooth log management.
2. CPU Overhead from Log Processing
Low-level log levels like debug
can result in excessive CPU usage from formatting and processing each log entry.
How to Mitigate:
- Adjust Log Levels: In production, use
:info
or:warn
instead of:debug
to limit log entries. - Log Filtering: Use
config.filter_parameters
to filter out sensitive data, reducing unnecessary log processing.
3. Excessive Memory Usage
Real-time logging, especially with large payloads, can consume significant memory as logs are processed and stored.
How to Mitigate:
- Log Level Optimization: In production, set log levels to
:error
or:fatal
to capture only critical events. - Garbage Collection: Optimize Ruby’s garbage collector and manage object allocations to reduce memory pressure.
4. Disk Space Consumption
Excessive logging over time can consume large amounts of disk space, leading to potential performance issues.
How to Mitigate:
- Log Rotation and Archiving: Set up log rotation to archive old logs and prevent unnecessary disk usage. Use tools like
logrotate
or Rails’ built-in rotation mechanisms.
5. Slow Database Queries in Logs
Logging database queries, especially complex ones, can add overhead to query performance.
How to Mitigate:
- Selective Query Logging: Only log slow queries. Set a threshold for query execution time to minimize the performance impact.
config.active_record.logger = Logger.new(STDOUT)
config.active_record.auto_explain_threshold_in_seconds = 0.5
This configuration logs only queries that take longer than 0.5 seconds, reducing unnecessary overhead.
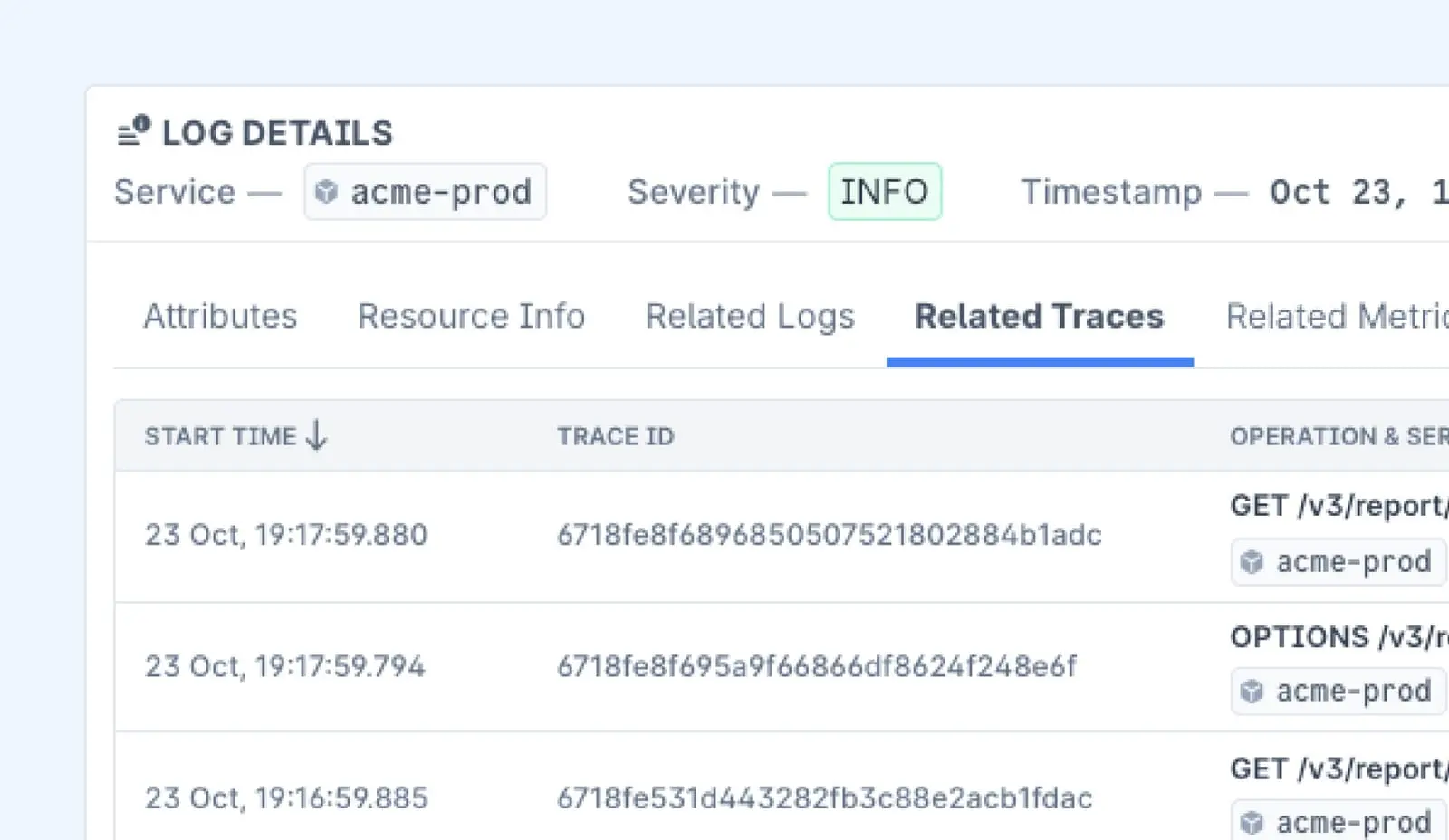
Conclusion
The Rails Logger is a versatile tool for monitoring and debugging, offering flexibility in customizing formats, setting up log rotation, and integrating with external services.
Tailoring it to your needs ensures your logs remain clear, manageable, and easy to analyze.