In web development, logging is a vital part of tracking your application’s behavior, especially when it comes to debugging and monitoring.
If you’re working with Express.js, one of the most common frameworks for building Node.js applications, you’ll want to streamline your logging. That’s where Morgan npm helps you.
In this guide, we’ll go over how to install Morgan, how it fits into your Express.js setup and its many powerful features. By the end, you’ll understand how Morgan enhances your Node.js applications with robust logging solutions.
What is Morgan?
Morgan is a middleware for Express.js that provides HTTP request logging. It’s built specifically to capture useful details about each request sent to your Express app, including the request method (GET, POST, PUT, etc.), status code, and response time ms.
This helps you track how your application is performing, troubleshoot issues, and monitor traffic.
But, what makes Morgan so special?
It’s all about its simplicity and flexibility. From logging to a log file to outputting response-time ms or even changing the format based on environments, Morgan logging ensures you have the information you need at your fingertips.
Here’s a simple example of how you can use Morgan in an Express app:
const express = require('express');
const morgan = require('morgan');
const app = express();
// Use Morgan middleware to log HTTP requests
app.use(morgan('combined')); // 'combined' is one of the predefined logging formats
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
In this example, we’ve set up Morgan middleware to log incoming HTTP requests using the 'combined' format.
This format includes important information like the status code, response time, and HTTP version while tracking the user-agent and content length. You can configure the log output to suit different environments.
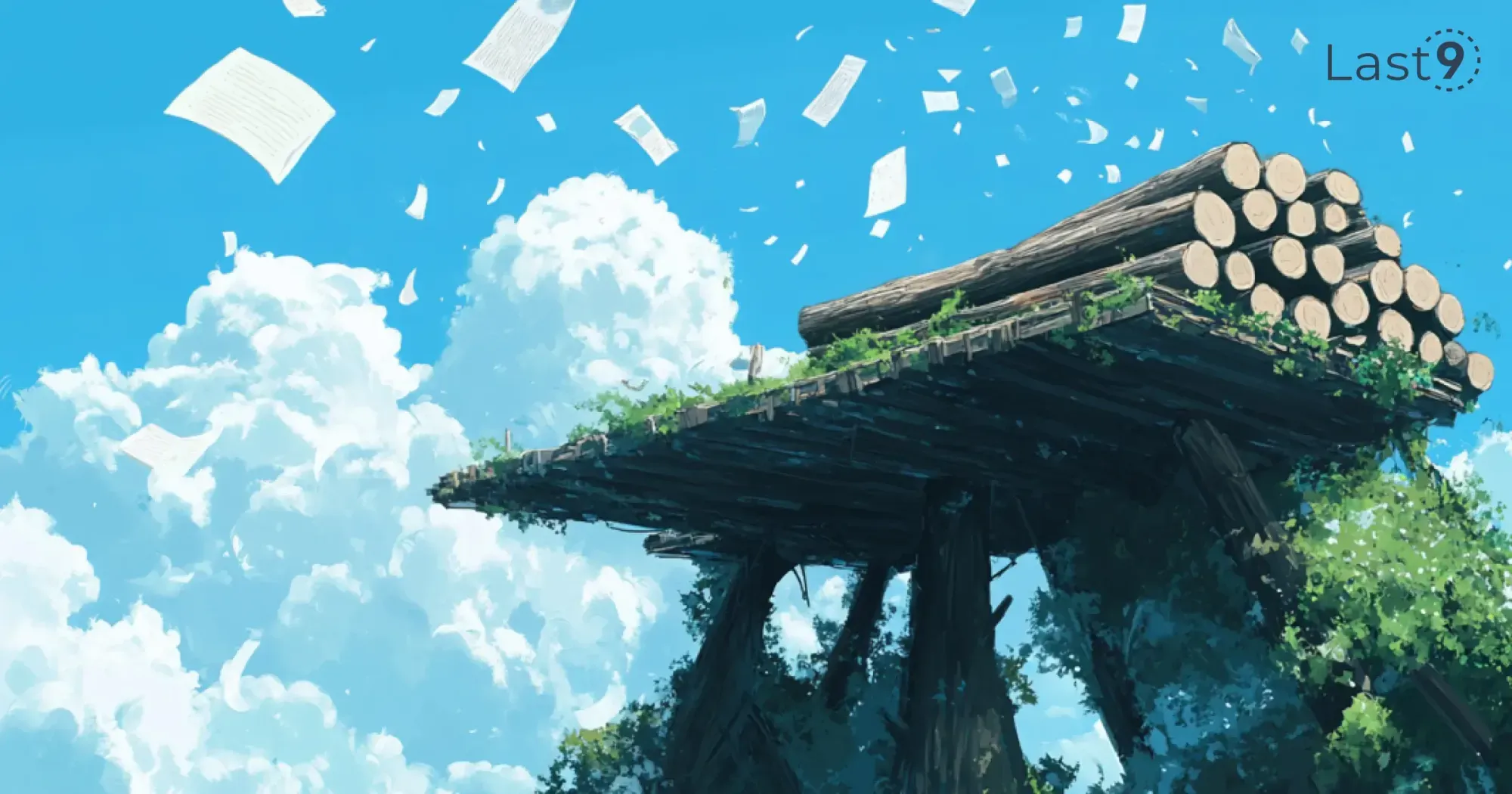
Why Should You Use Morgan?
When building applications in Node.js and Express, request logging is essential. Here’s why Morgan is a great choice for your Express app:
- Easy Setup: It integrates seamlessly into your Express application. With just a few lines of code, you can start logging requests to the console or even a log file.
- Customizable Formats: Choose from predefined formats like ‘combined’ or ‘dev’, or create your own custom format strings. This flexibility allows you to tailor logs for debugging, monitoring, and more.
- Monitor Performance: Track response-time ms to monitor how long each HTTP request takes. This helps you identify slow requests and optimize performance.
- Environment-Specific Configurations: Whether you’re in development or production, you can configure Morgan to log in different formats, ensuring the right level of detail for each environment.
- Log to a File: Instead of logging to the console, Morgan can write logs to a log file for persistent storage or integration with tools like Winston or MongoDB, making it easier to analyze logs later.
Morgan makes request logging simple, flexible, and powerful for Node.js and Express applications!
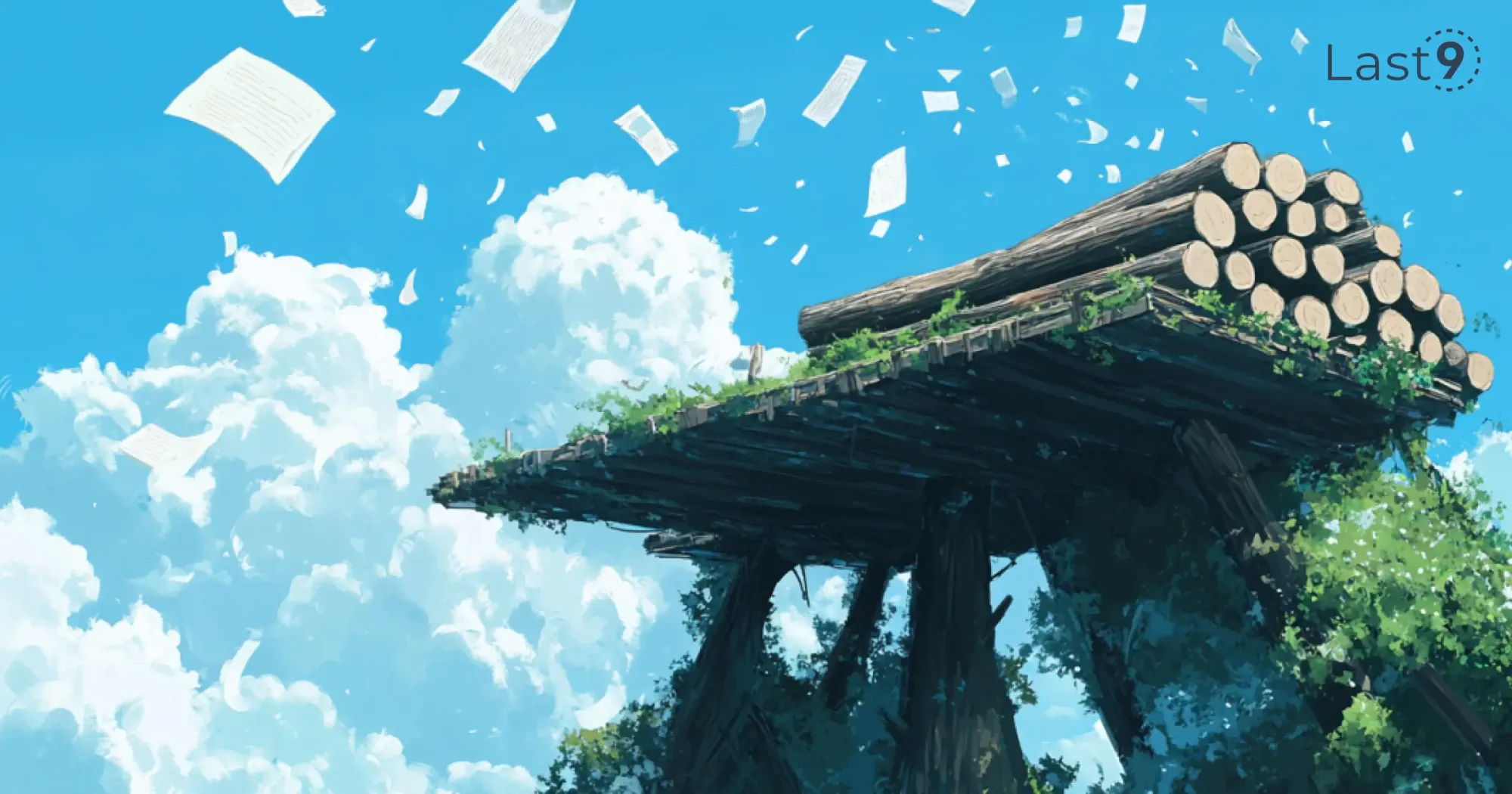
Let’s take a look at how Morgan helps with HTTP request logging by breaking down its output:
- Status Code: Indicates whether the request was successful or if there was an error (like 404 for "Not Found" or 500 for "Internal Server Error").
- Response Time: The time it took for your server to process the request and send a response.
- HTTP Version: Logs the version of HTTP (like HTTP/1.1 or HTTP/2) used for the request.
- User-Agent: Tracks what browser or tool made the request, useful for debugging user-facing issues.
Installing Morgan for Your Node.js App
To get started with Morgan logging, you’ll need to install the Morgan npm package. You can easily do this via npm (Node Package Manager):
npm install morgan
Once Morgan is installed, you can require it in your index.js or app.js file and set it up as middleware.
Here’s a quick example of how to use Morgan for logging requests in your Node.js application:
const express = require('express');
const morgan = require('morgan');
const app = express();
// Use Morgan middleware to log HTTP requests
app.use(morgan('dev')); // 'dev' is a lightweight logging format
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
In the example above, we use morgan('dev')
, which outputs the logs in a concise format suitable for development environments. The logs will include the status code, response time, HTTP version, and more.
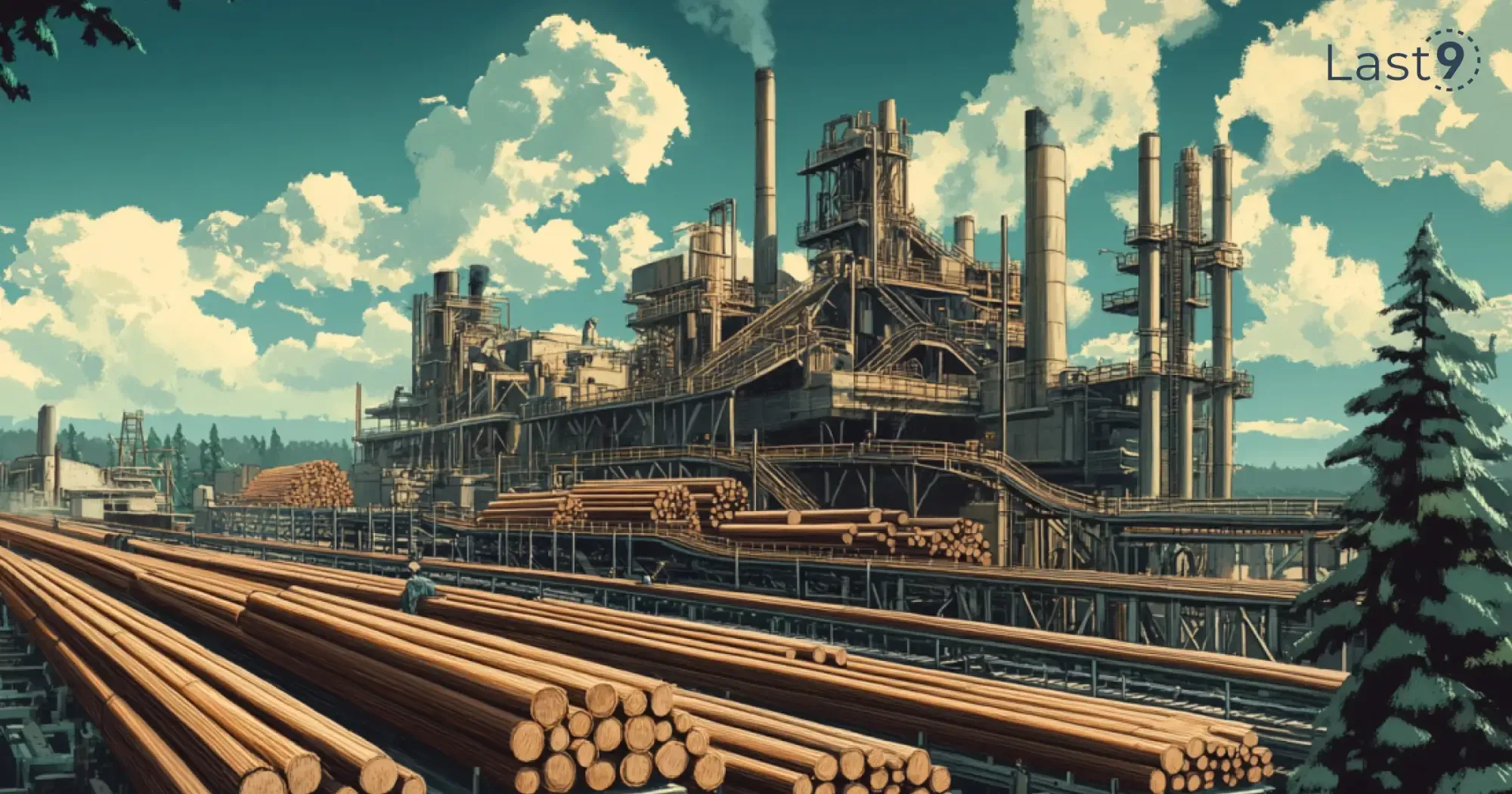
What is Middleware?
Middleware in Express refers to functions that have access to the request (req) and response (res) objects and can modify them before the final response is sent.
Morgan is a middleware that runs before your route handlers, capturing details about the HTTP request (such as the method, URL, and user-agent) and logging them according to the format you specify.
In an Express.js app, middleware functions like Morgan are used to handle tasks such as logging, authentication, error handling, and more. Here’s a simple breakdown of how middleware fits into the Express app lifecycle:
app.use(morgan('combined')); // Logging middleware
app.use(express.json()); // Parses JSON bodies
app.get('/api', (req, res) => {
res.send('Hello, World!');
});
Middleware sits in between the request and the final route handler, making it an essential part of the Express request handling process.
Different Logging Formats in Morgan
One of the coolest features of Morgan is the ability to configure predefined formats for logging. These formats provide various levels of detail for the logs:
- 'combined': This format is great for production environments and includes details like the remote address, status code, content length, and more.
- 'dev': The ‘dev’ format is more compact and colored, making it ideal for development environments where you need quick access to basic logging details.
- 'short': A condensed version of the logs, displaying basic details about the request.
- 'tiny': The simplest format that logs just the status code and response time.
If these formats don’t meet your needs, you can also define a custom format using Morgan tokens, like logging the user-agent or response time in a specific structure.
What is HTTP Request Logging?
HTTP request logging is the process of tracking the requests made to your server, including information such as the method, status code, user-agent, and response time.
It’s essential for debugging, monitoring performance, and ensuring that your application is functioning as expected.
In Express.js, you can use Morgan to log HTTP requests efficiently. These logs provide critical information that helps developers understand how users interact with their applications, what errors might have occurred, and how long requests are taking to process.
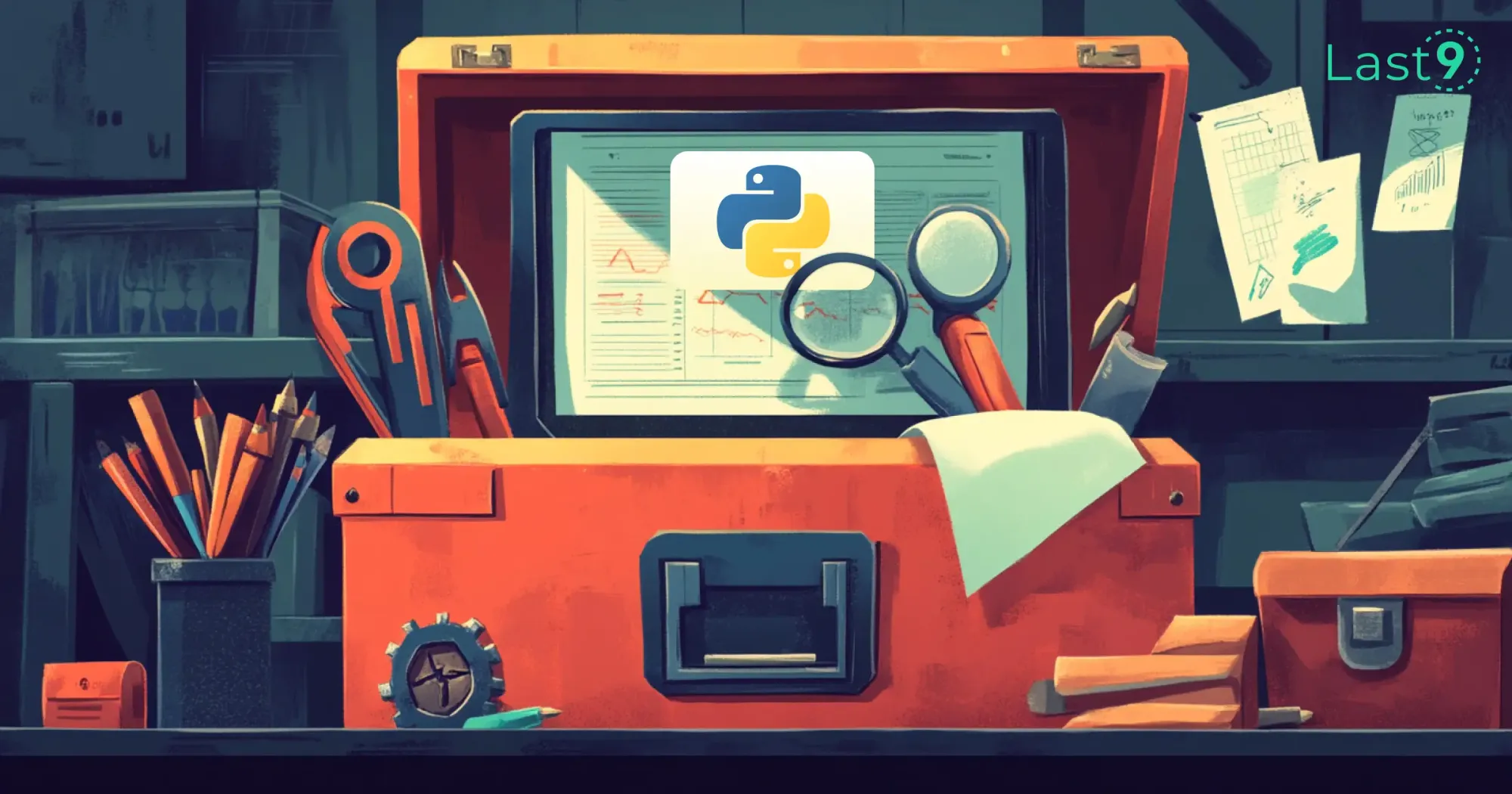
Advanced Configuration and FAQs
Configuring Morgan for Different Environments
Depending on whether you’re working in development, staging, or production, you may want different levels of logging detail. Morgan allows you to adjust the logging format dynamically based on the environment, giving you the flexibility to customize the logging output accordingly.
Here’s an example of how you might configure Morgan differently for development and production:
const express = require('express');
const morgan = require('morgan');
const app = express();
// Determine environment and set Morgan logging format
if (process.env.NODE_ENV === 'production') {
app.use(morgan('combined')); // Use 'combined' in production for more detailed logs
} else {
app.use(morgan('dev')); // Use 'dev' in development for quicker, color-coded logs
}
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
In this setup:
- Morgan’s 'combined' format is used for production environments, logging detailed request data like the user-agent, status code, and response time.
- The 'dev' format is used for development, providing a simpler and color-coded output to quickly track requests while developing.
Tailoring your logging format to the environment ensures you get the right level of detail without overwhelming yourself with unnecessary data.
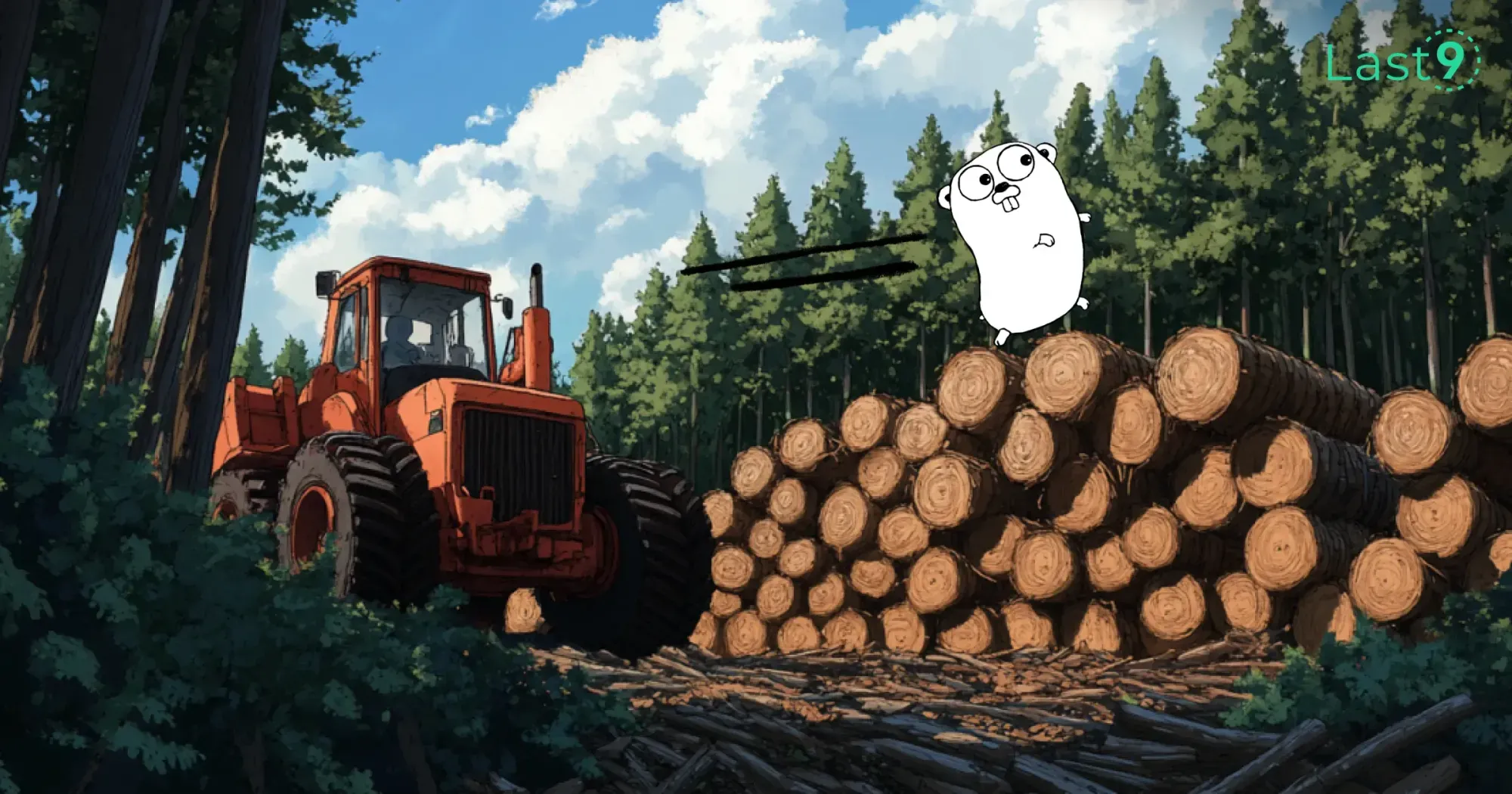
How to Output Logs in JSON Format
For advanced logging scenarios, you might prefer to output logs in JSON format for better integration with logging systems or log aggregation tools. To do this, you can customize the Morgan middleware by creating a write stream that formats logs as JSON.
Here’s an example of how to configure Morgan to output logs in JSON format:
const fs = require('fs');
const morgan = require('morgan');
const express = require('express');
const app = express();
// Create a write stream in append mode
const logStream = fs.createWriteStream('./logs/access.log', { flags: 'a' });
// Define a custom JSON format for logging
morgan.token('json', (req, res) => {
return JSON.stringify({
method: req.method,
url: req.originalUrl,
status: res.statusCode,
responseTime: res.responseTime,
date: new Date().toISOString(),
});
});
// Use Morgan to log requests in JSON format
app.use(morgan(':json', { stream: logStream }));
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
In this example, we define a custom Morgan token to format each log entry as a JSON object. This output will be written to the log file access.log
, which can be further processed by log management tools like Winston, MongoDB, or any other log aggregation system.
Sending logs in JSON format makes them machine-readable, which is great for monitoring and analyzing large-scale applications.
How to Install Morgan with npm
Installing Morgan in your Node.js application is straightforward. Simply run the following command in your project directory:
npm install morgan
Once installed, you can require it in your index.js
or app.js
file and set it up as middleware in your Express.js app. It’s that simple to get started with Morgan logging.
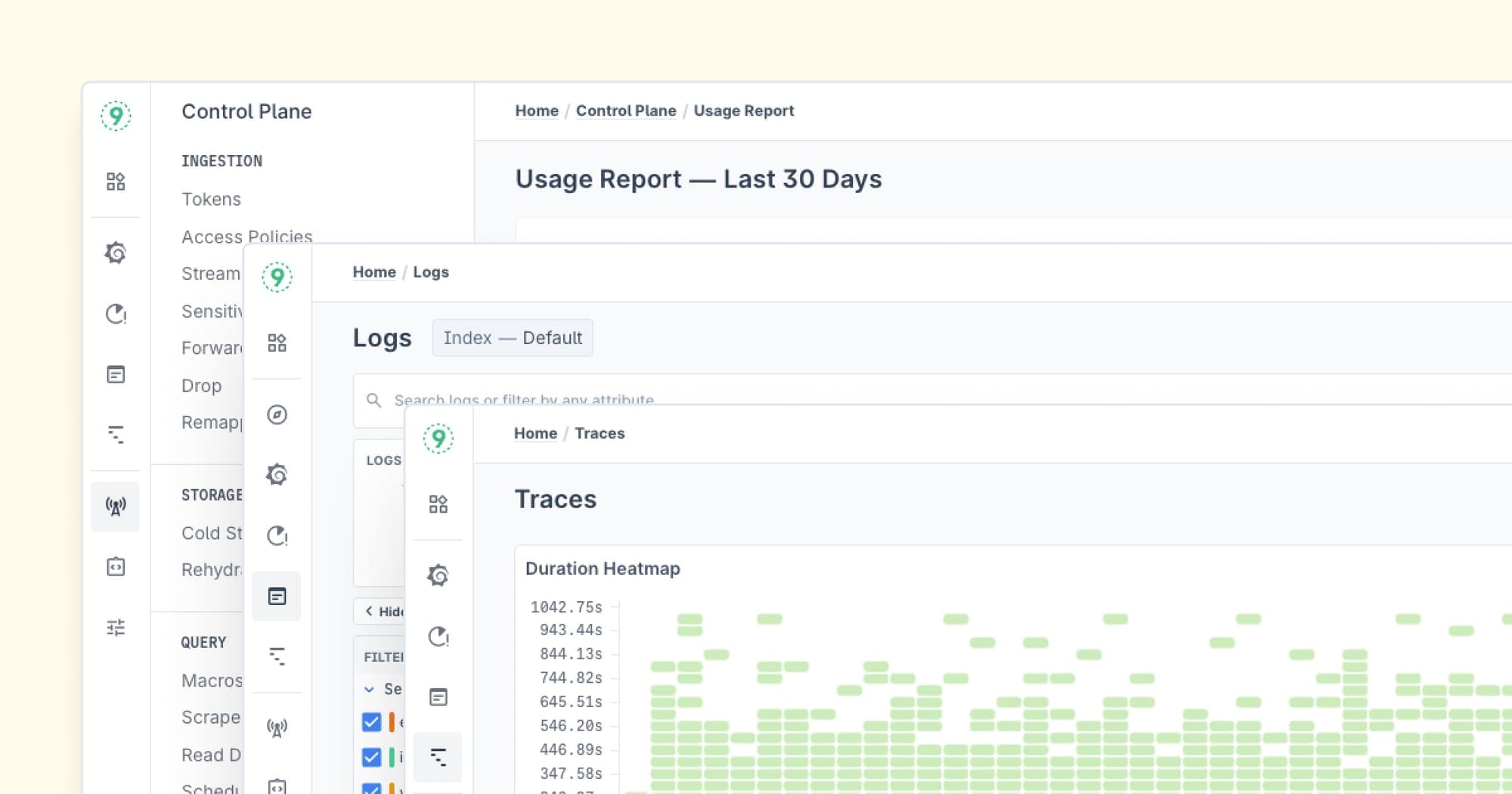
Conclusion
In conclusion, Morgan npm is an essential tool for Node.js and Express.js developers who want to manage and monitor their application's HTTP request logging.
Remember, Morgan isn’t just about logging — it’s about making your life easier by giving you the right information when you need it, in a format that fits your development environment. Whether you’re building simple Express applications or complex Node.js services, Morgan is a logging tool you can count on.
On the other hand, if you're looking for a managed observability solution, Last9 can help you with everything you need. Logs, Traces, and Metrics in one unified platform.
With Last9, we eliminated the toil. It just works.
— Matt Iselin, Head of SRE, Replit
Schedule a demo with us or try it for free to see how it makes your observability simpler and cost-effective.
FAQs
What is Morgan npm?
Morgan npm is a logging middleware for Express.js applications. It automatically logs incoming HTTP requests and their responses. Morgan can output logs in various formats like dev, combined, or even custom formats. It’s typically used for monitoring and debugging purposes in Node.js applications.
What is the use of Morgan middleware?
Morgan middleware helps you log HTTP requests in your Express.js application. It tracks details such as the method, status code, response time, and user-agent, making it easier to debug and monitor your app. You can also log these details to a log file or output them in JSON format.
Who is npm owned by?
npm (Node Package Manager) is owned by GitHub. It’s a tool that helps developers manage dependencies in their JavaScript applications and is integral to the Node.js ecosystem. npm allows you to install and update packages like Morgan, and it simplifies package management within your projects.
What is npm?
In simple terms, npm is a package manager for JavaScript. It allows developers to easily install, update, and manage libraries (like Morgan) and tools for Node.js applications. With npm, you can quickly add dependencies to your project, manage versions, and keep your app up to date with the latest packages.
What is MORGAN in Node.js?
Morgan is a middleware for logging HTTP requests in Node.js applications built with Express.js. It logs useful information about incoming requests such as the method, URL, status code, and response time. Morgan helps with debugging and monitoring your Express app.
Why Should You Use Morgan?
You should use Morgan because it simplifies logging HTTP requests in your Express.js app. It provides real-time logging, supports different logging formats, and can log to both the console and log files. Using Morgan makes it easier to debug and monitor your app’s performance.
What can Morgan do for an Express app?
Morgan provides logging capabilities for HTTP requests and responses in an Express.js application. It tracks details like status codes, response time, and HTTP methods, and can log this information to the console or a log file. Morgan makes it easier to debug and optimize your Express app by keeping track of all incoming requests.
What is Middleware?
Middleware in Express.js refers to functions that have access to the request and response objects and can modify or handle them before the final response is sent. It’s commonly used for logging, authentication, error handling, and more.
How does it fit in with other loggers?
Morgan works alongside other loggers (like Winston or Apache logs) by providing a simple, real-time logging solution for HTTP requests in Express.js. You can combine Morgan with Winston to log to files or send logs to a centralized logging system for further analysis.
What if you wanted to send it back to them in JSON format?
If you want to send your logs in JSON format, you can customize Morgan to log in JSON. This can be done by using Morgan tokens and creating a custom logging format that outputs logs as structured JSON, which is perfect for machine-readable logs and integration with logging systems.