Log parsing plays a critical role in modern observability, enabling engineers to analyze the vast streams of data generated by servers, applications, and services.
The Grok debugger is an essential tool for efficiently interpreting logs, yet its full capabilities often remain underutilized.
This guide provides a detailed exploration of Grok debugging, offering insights into its mechanics and practical applications to help you optimize your log analysis processes.
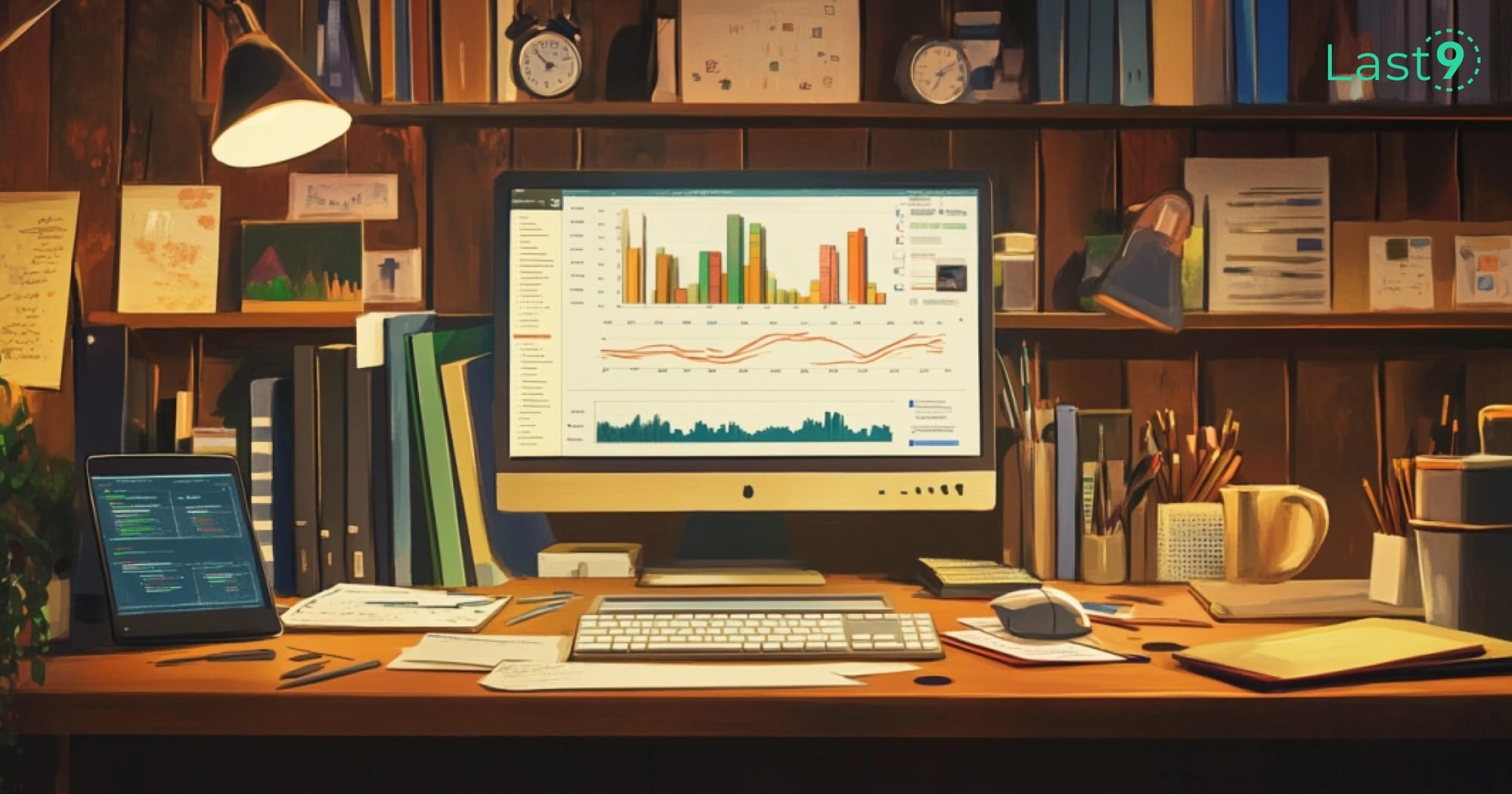
Understanding Pattern Matching Fundamentals
The Theory Behind Grok
At its foundation, the Grok debugger relies on pattern-matching principles derived from formal language theory. A solid understanding of these principles can elevate your debugging game:
- Regular Expressions (Regex): The building block for matching text patterns.
- Finite Automata: The concept that underpins how Grok interprets patterns.
- Pattern Composition: Rules for combining smaller patterns into larger, reusable ones.
- Capture Groups: Assigning meaning to matched values for better log interpretation.
Grok Pattern Architecture
Every Grok pattern follows a simple yet flexible syntax:
%{SYNTAX:SEMANTIC}
- SYNTAX: The specific pattern to match, like numbers or words.
- SEMANTIC: The identifier assigned to the matched value.
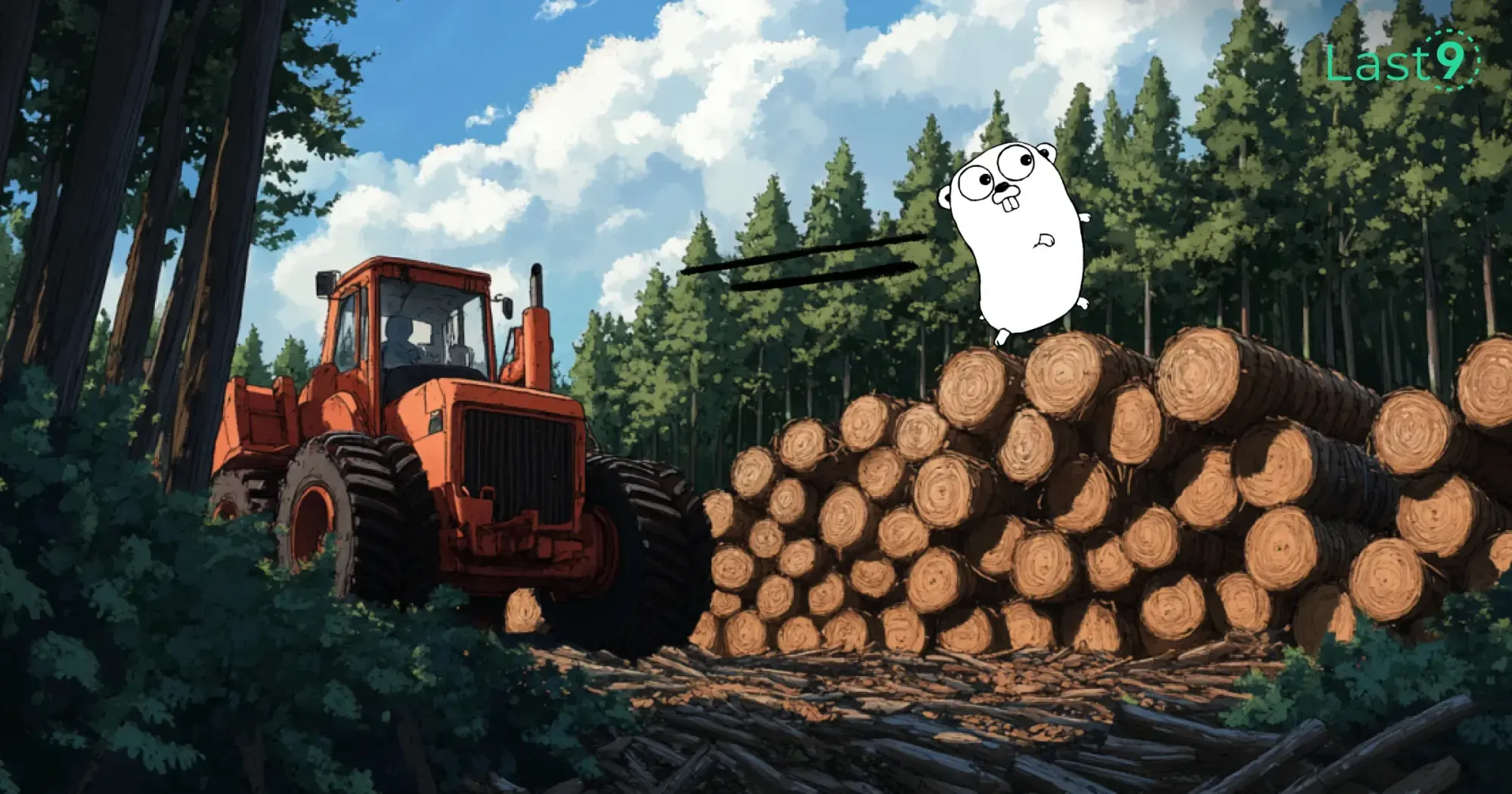
Common Pattern Types in Grok
Here are a few Grok patterns you’ll encounter frequently:
%{NUMBER} # Matches numeric values
%{WORD} # Matches alphanumeric words
%{GREEDYDATA} # Matches everything (be cautious!)
# Named captures: Assign meaning to values
%{NUMBER:duration} # Captures a number as 'duration'
%{WORD:action} # Captures a word as 'action'
Practical Implementation with the Grok Debugger
Basic Pattern Structure
Consider this example log:
2024-03-27 10:15:30 ERROR [ServiceName] Failed to process request #12345
To match this log, use the following Grok pattern:
%{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:level} \[%{WORD:service}\] %{GREEDYDATA:message}
Here’s how the pattern works:
- %{TIMESTAMP_ISO8601:timestamp}: Matches and labels the timestamp.
- %{LOGLEVEL:level}: Captures the log level (e.g., ERROR).
- %{WORD:service}: Identifies the service name within square brackets.
- %{GREEDYDATA:message}: Grabs the remaining log message.
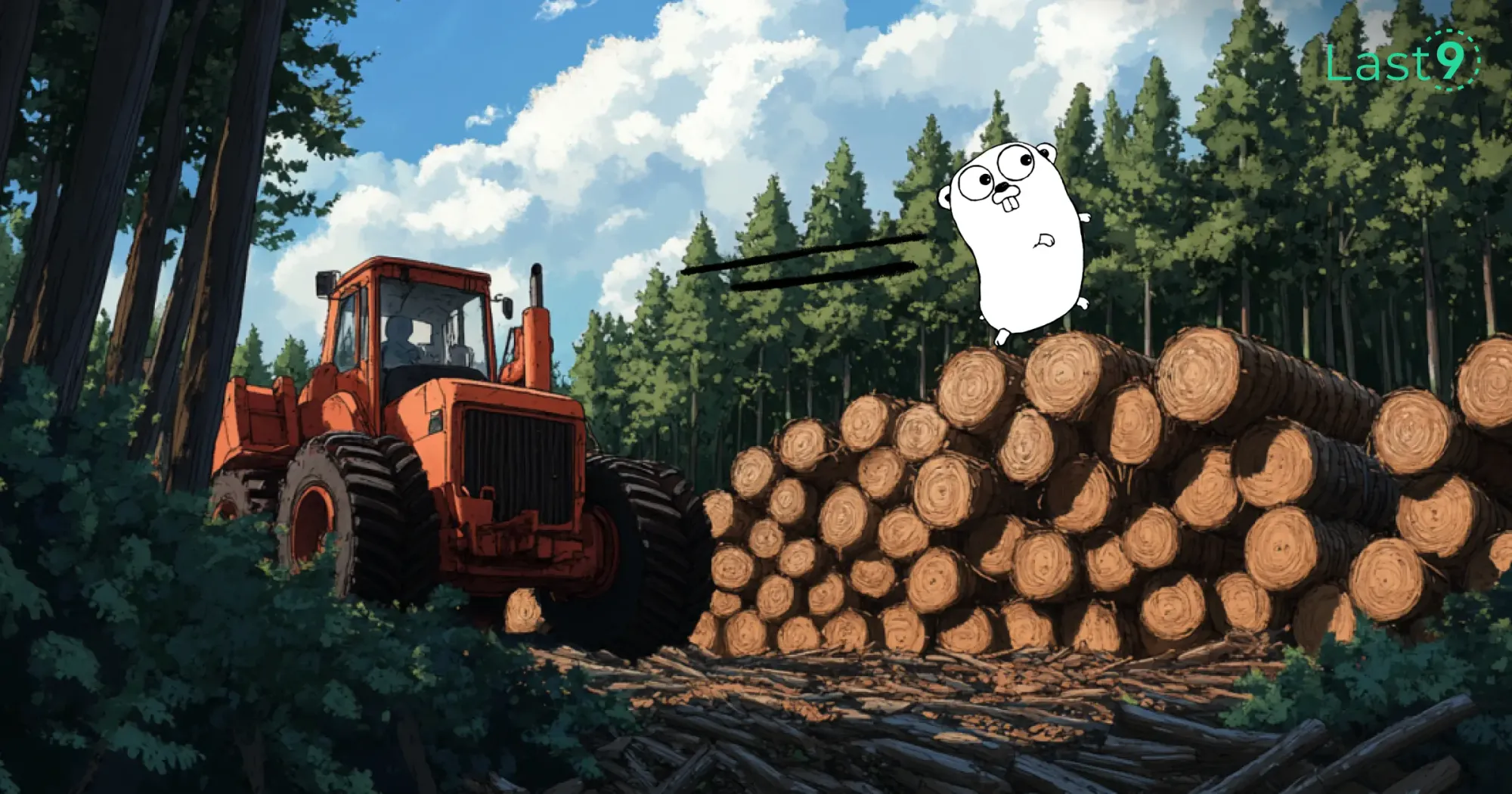
Pattern Development Workflow
Break Down the Log:
Identify each log component. For example:
- TIMESTAMP: 2024-03-27 10:15:30
- LEVEL: ERROR
- SERVICE: [ServiceName]
- MESSAGE: Failed to process request #12345
Build Incrementally:
Start simple and add components step by step:
# Step 1: Match the timestamp
%{TIMESTAMP_ISO8601:timestamp}
# Step 2: Add the log level
%{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:level}
# Step 3: Include the service name
%{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:level} \[%{WORD:service}\]
# Final: Capture the full message
%{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:level} \[%{WORD:service}\] %{GREEDYDATA:message}
Debugging Techniques in the Grok Debugger
Common Issues and Solutions
- Pattern Not Matching:
Symptoms: Your pattern doesn’t match the expected log.- Debug Tips:
- Check for invisible characters like tabs or extra spaces.
- Validate the pattern with a smaller portion of the log.
- Debug Tips:
- Partial Matches:
Symptoms: The pattern works for part of the log but fails elsewhere.
- Example:
# Problematic log:
2024-03-27 10:15:30.123 ERROR Service error
# Initial pattern:
%{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:level}
# Solution (include milliseconds):
%{TIMESTAMP_ISO8601:timestamp}.%{INT:ms} %{LOGLEVEL:level}
Using the Grok Debugger Effectively
- Test Patterns: Validate against multiple log samples to ensure accuracy.
# Test case
%{NUMBER:response_time}ms %{WORD:status}
# Logs:
100ms SUCCESS
50ms FAILURE
- Edge Cases: Include logs with special characters, empty fields, or unusual formats.
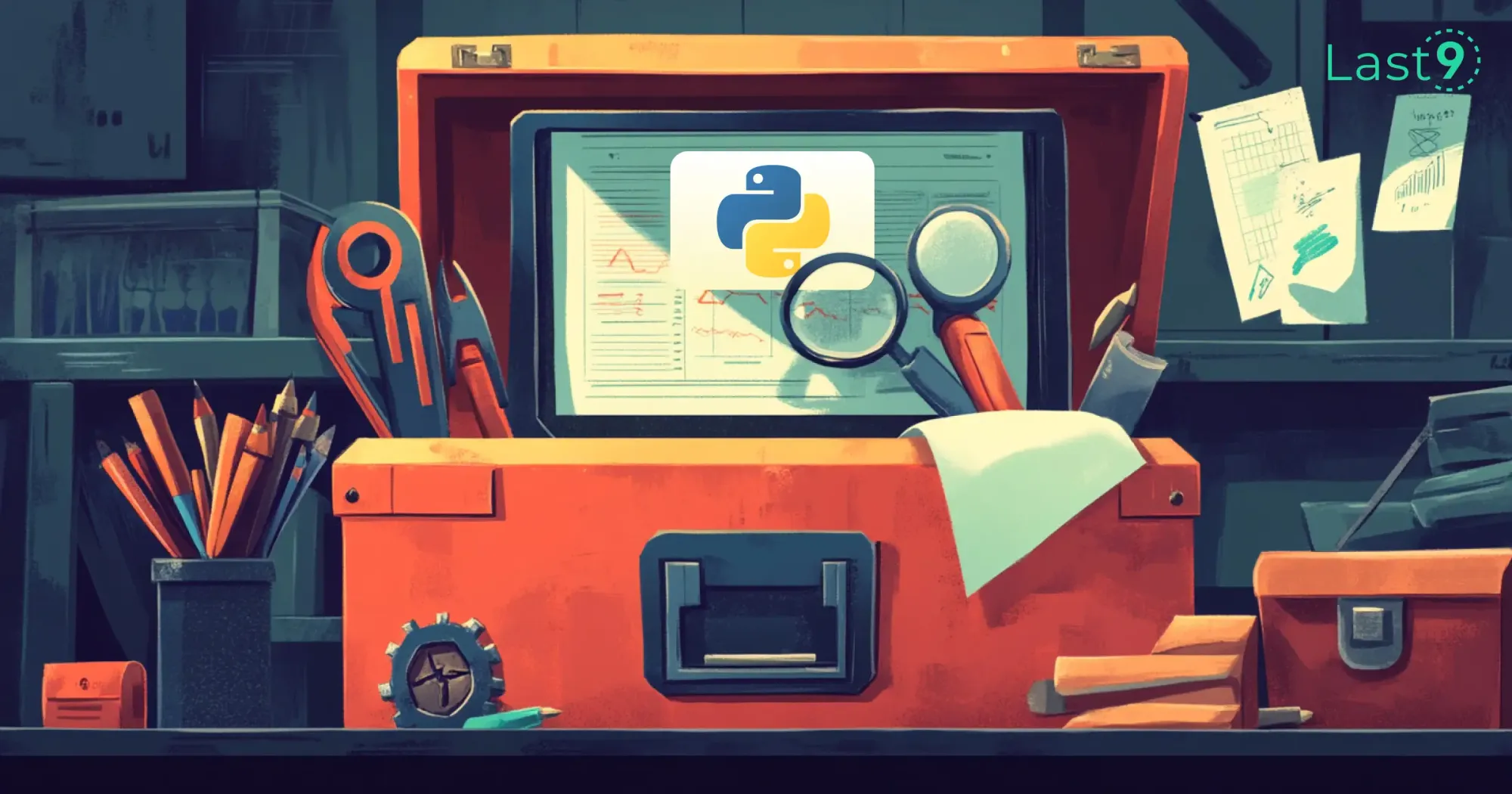
Advanced Patterns and Optimization
Custom Pattern Development
When standard patterns fall short, create custom ones:
# Custom patterns
RESPONSE_CODE [1-5][0-9][0-9]
SERVICE_NAME [A-Za-z]+[-_]?[A-Za-z0-9]+
# Use in main Grok pattern
%{TIMESTAMP_ISO8601:timestamp} %{SERVICE_NAME:service} %{RESPONSE_CODE:status}
Performance Optimization
Optimizing patterns can save resources, especially with large datasets:
- Efficient Pattern Design:
# Inefficient pattern
.*%{WORD:service}.*%{GREEDYDATA:message}
# Optimized pattern
%{WORD:service}[^}]*%{GREEDYDATA:message}
- Memory Management: Avoid redundant capture groups:
# High memory usage
(%{GREEDYDATA:field1}|%{GREEDYDATA:field2})
# Optimized
%{GREEDYDATA:field}
Common Pitfalls and Best Practices
Pitfalls to Avoid
- Over-Complicated Patterns:
Avoid unnecessary complexity:
# Over-complicated
%{TIMESTAMP_ISO8601:timestamp}(?:%{SPACE})?%{LOGLEVEL:level}(?:%{SPACE})?%{GREEDYDATA:message}
# Simplified
%{TIMESTAMP_ISO8601:timestamp}\s+%{LOGLEVEL:level}\s+%{GREEDYDATA:message}
- Insufficient Testing: Always test with:
- Valid logs
- Invalid logs
- Edge cases
- Special characters
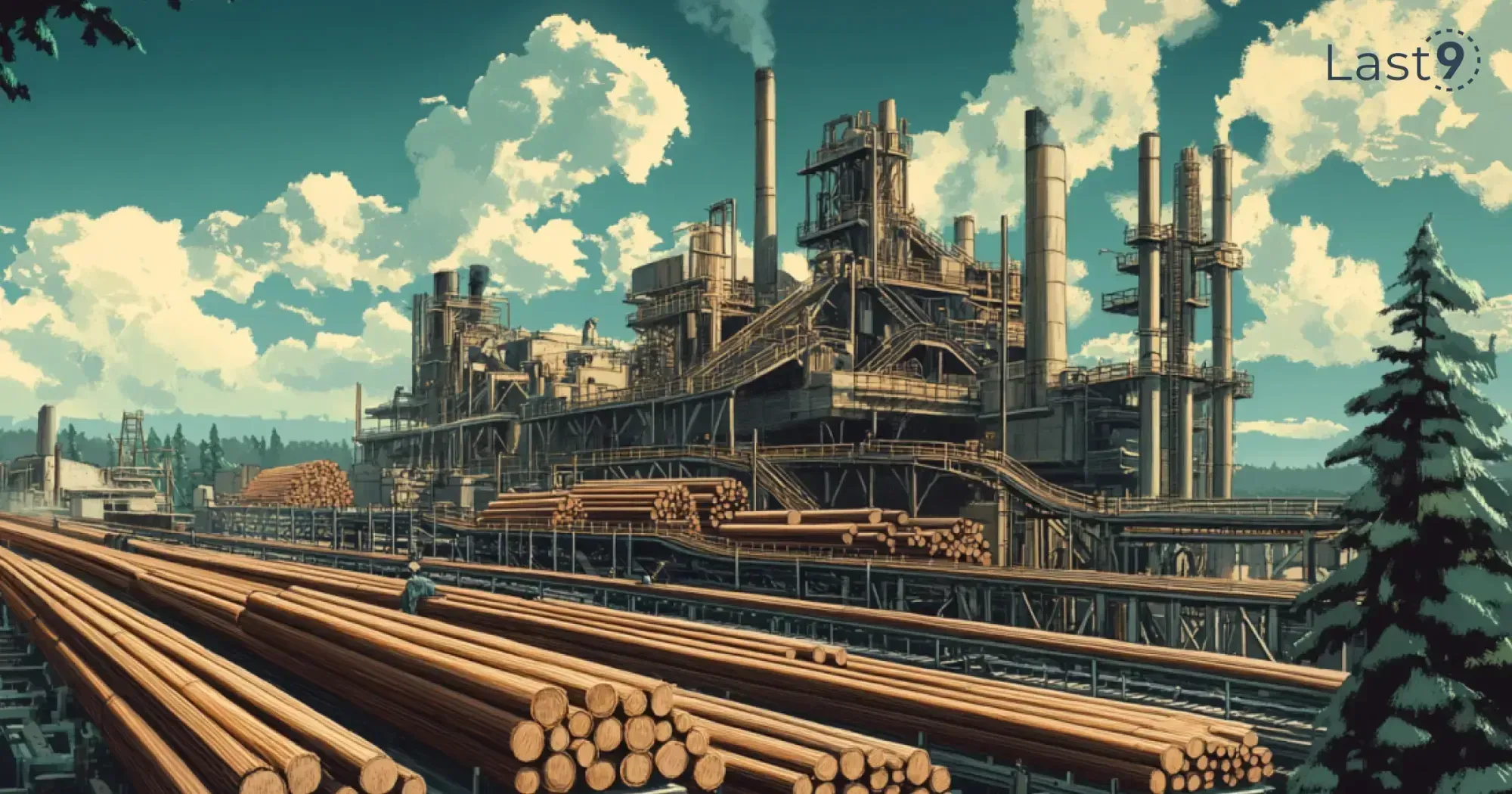
Best Practices
- Pattern Organization: Keep patterns modular and reusable:
# Modular patterns
TIMESTAMP_PATTERNS %{TIMESTAMP_ISO8601}|%{TIMESTAMP_UNIX}
LOG_LEVEL (DEBUG|INFO|WARN|ERROR|FATAL)
# Main pattern
%{TIMESTAMP_PATTERNS:timestamp} %{LOG_LEVEL:level}
- Document Your Patterns:
# Pattern documentation
# PATTERN: APP_LOG
# DESCRIPTION: Matches application log format
# EXAMPLE: 2024-03-27 10:15:30 ERROR [Service] Message
APP_LOG %{TIMESTAMP_ISO8601:timestamp} %{LOGLEVEL:level} \[%{WORD:service}\] %{GREEDYDATA:message}
Conclusion
The Grok debugger is more than a tool—it's a superpower for log parsing and debugging. Remember, success with Grok comes from an iterative approach:
- Start simple.
- Test extensively.
- Refine constantly.
Logs don’t have to be confusing. With the Grok debugger, they can reveal the story behind your system.
At Last9, we bring together traces, metrics, and logs to help you troubleshoot faster and understand your infrastructure better.
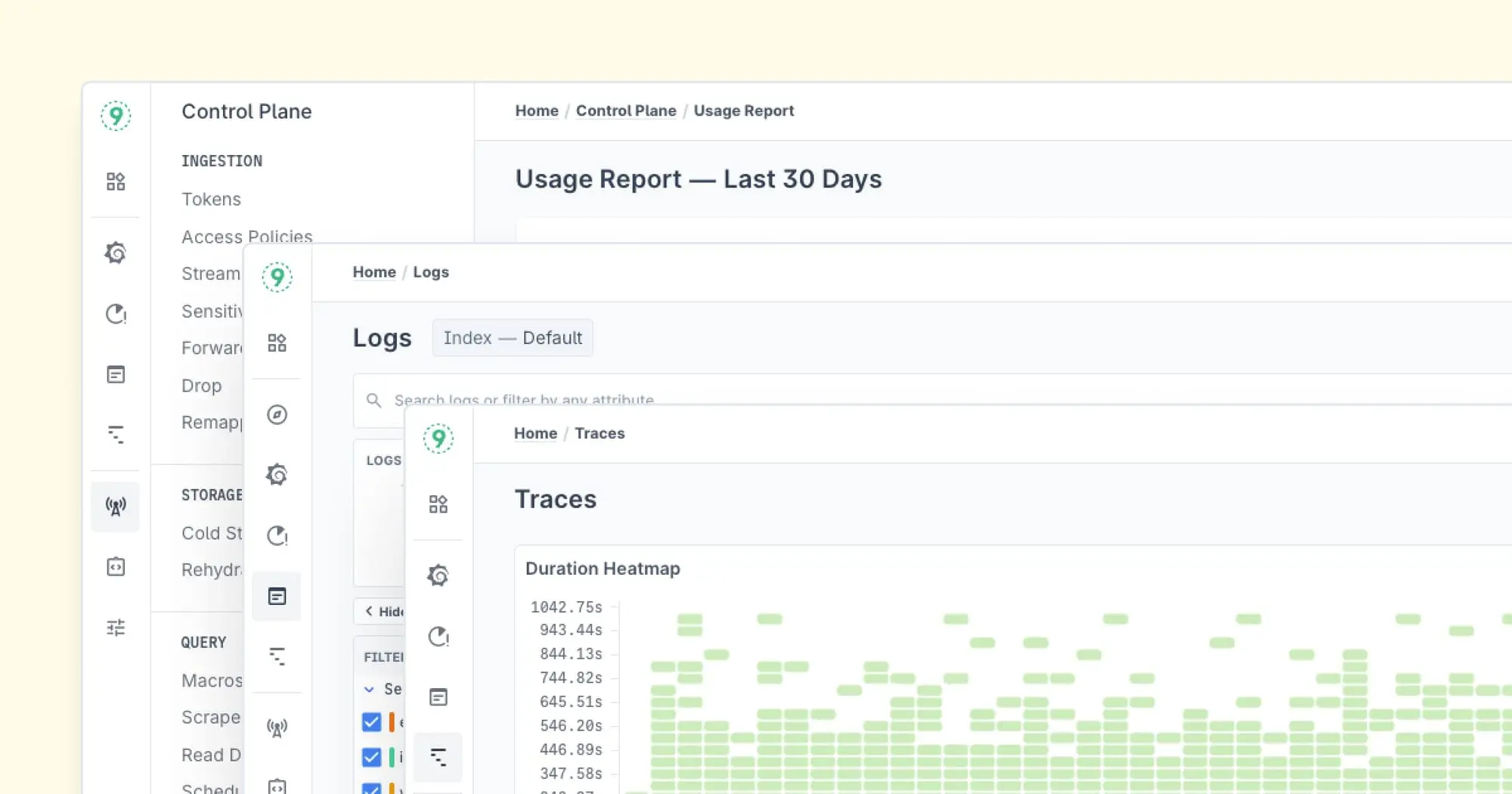
Schedule a demo with us or try it for free to learn more!
FAQs
1. What is a Grok debugger used for?
The Grok debugger is a tool designed to parse and test Grok patterns. It helps users validate their patterns against log formats, identify issues, and fine-tune them for better accuracy.
2. What is the syntax for Grok patterns?
The basic syntax of Grok patterns is %{SYNTAX:SEMANTIC}
, where:
- SYNTAX represents the predefined pattern (e.g.,
%{NUMBER}
,%{WORD}
). - SEMANTIC is the user-defined field name to capture the data (e.g.,
%{NUMBER:duration}
).
3. How do I debug a Grok pattern that doesn’t work?
To debug:
- Simplify the pattern to isolate the issue.
- Test each section incrementally.
- Look for special characters, hidden spaces, or incorrect syntax.
- Use the Grok debugger tool to test the pattern with sample logs.
4. Can I create custom Grok patterns?
Yes! You can create custom patterns using regex and assign them meaningful names. For example:
RESPONSE_CODE [1-5][0-9][0-9]
You can then use %{RESPONSE_CODE:status}
in your patterns.
5. Why is my pattern partially matching the log?
Partial matches occur due to:
- Missing parts of the log in the pattern.
- Incorrect assumptions about separators (e.g., spaces vs. tabs).
- Misalignment in data types.
Verify the full log format and adjust your pattern accordingly.
6. How do I optimize Grok patterns for better performance?
- Avoid overly greedy patterns like
.*
or%{GREEDYDATA}
where not necessary. - Use specific patterns instead of generic ones.
- Combine related patterns into reusable components.
- Test with a variety of log samples to ensure efficiency.
7. What’s the difference between %{WORD}
and %{GREEDYDATA}
?
%{WORD}
matches only word characters (letters, numbers, or underscores).%{GREEDYDATA}
matches everything, including spaces and special characters. Use%{GREEDYDATA}
sparingly to avoid inefficiency.
8. Can I use the Grok debugger for JSON logs?
Yes, but JSON logs often require preprocessing to flatten their structure into a format Grok can parse effectively. Tools like jq
can help transform JSON logs before using Grok patterns.
9. Are there any tools to test Grok patterns online?
Yes! Several online Grok debuggers are available, such as:
- Grok Constructor
- Kibana’s built-in Grok debugger (if using the ELK stack).
10. How can I handle multiline logs with Grok?
Multiline logs need preprocessing to combine them into a single line. Tools like Logstash can be configured with multiline
filters to ensure the Grok debugger processes them correctly.
11. What are some common pitfalls in using Grok debugger?
- Relying too much on
%{GREEDYDATA}
. - Not testing with diverse log samples.
- Overcomplicating patterns with nested or redundant elements.
- Ignoring hidden characters or whitespace issues.
12. Where can I find predefined Grok patterns?
Predefined patterns are available in the Grok pattern library. You can also explore community-contributed patterns or create your own.
Feel free to reach out with more questions, and happy debugging!