Dec 13th, ‘24/7 min read
Docker Compose Logs: An In-Depth Guide for Developers
Master Docker Compose logs with our in-depth guide. Learn log commands, tips for effective management, and troubleshooting multi-container apps!
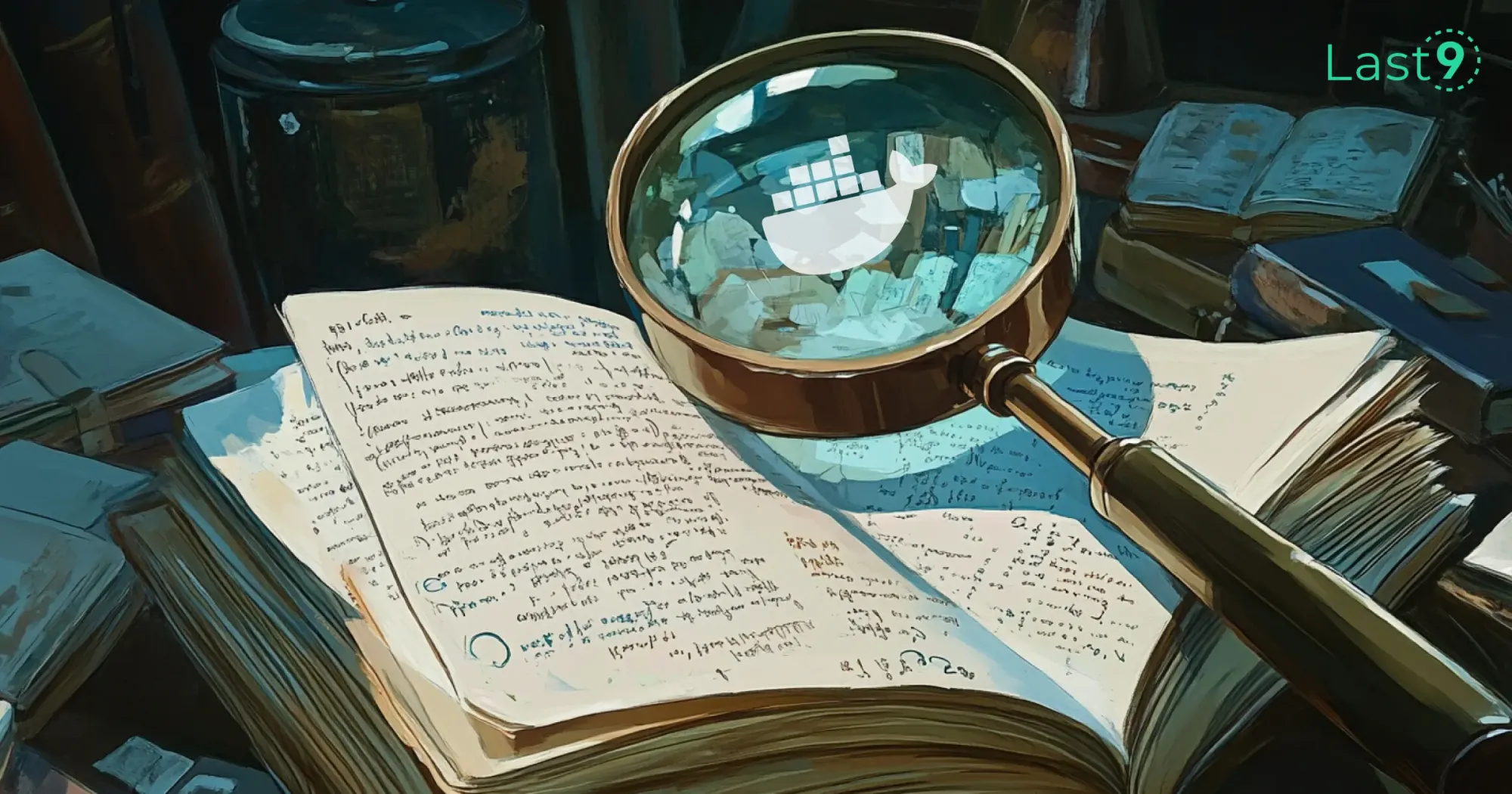
When working with containerized applications, troubleshooting often starts with logs. Docker Compose, a popular tool for managing multi-container Docker applications, makes it easy to gather and analyze logs from multiple services in your stack.
In this guide, we’ll explore everything you need to know about Docker Compose logs: how they work, tips for effective log management, and best practices for maintaining clear, actionable insights into your containers’ behavior.
What Are Docker Compose Logs?
Docker Compose logs are a unified way to view the logs of all services defined in your docker-compose.yml
file. Instead of inspecting logs service by service, docker-compose logs
aggregates them, making debugging and monitoring more efficient.
By default, these logs capture standard output (stdout
) and standard error (stderr
) streams from your running containers.
Depending on your configuration, you can route these logs to specific drivers for storage, analysis, or further processing. These logs are crucial when analyzing service logs across multi-container applications.
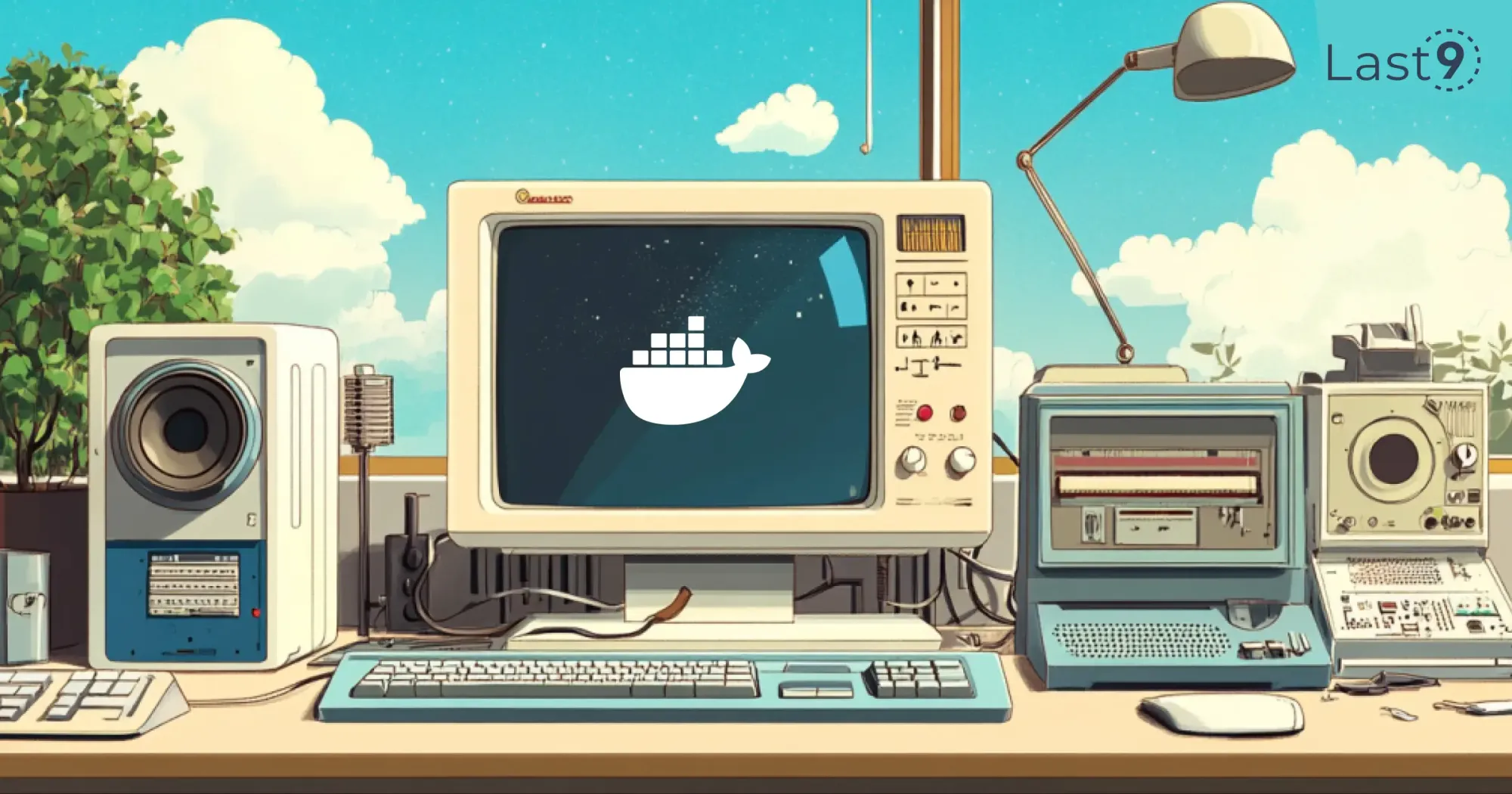
How to View Docker Compose Logs
The command to access logs is straightforward:
docker-compose logs
Key Options:
--follow
: Streams logs in real-time, similar to tail -f
.
docker-compose logs --follow
<service-name>
: View logs from a specific service.
docker-compose logs <service-name>
--tail
: Limits the number of log lines displayed.
docker-compose logs --tail=100
--timestamps
: Adds timestamps to each log entry for better context.
docker-compose logs --timestamps
--follow
: Streams logs in real-time, similar to tail -f
.
docker-compose logs --follow
The docker-compose logs
command is especially useful for troubleshooting container logs in real-time during development.
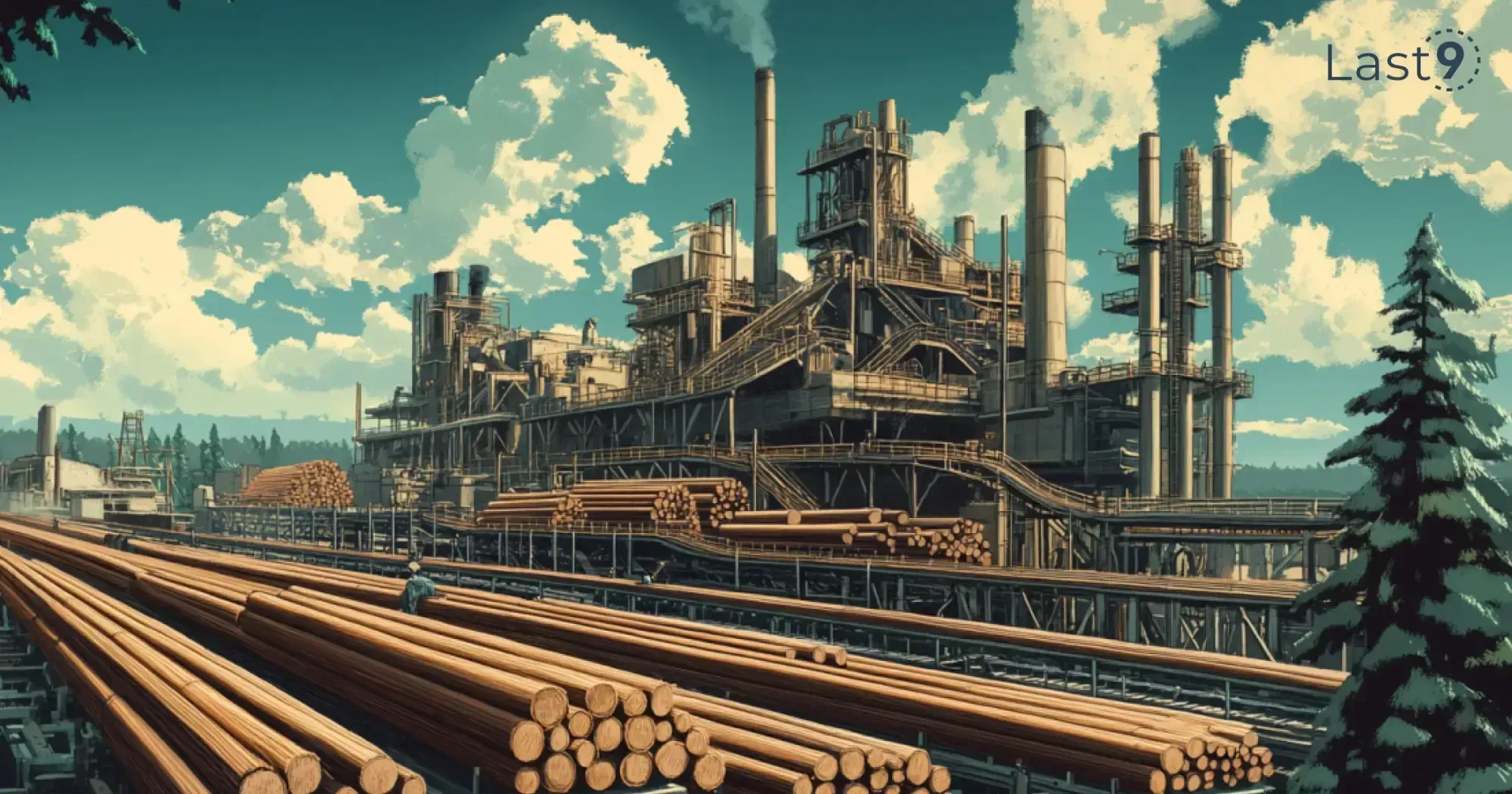
Configuring Logging in Docker Compose
Docker Compose uses the Docker logging drivers to control how logs are handled. You can specify logging options in your docker-compose.yml
file under the logging
key.
Example Configuration:
services:
web:
image: nginx
logging:
driver: "json-file"
options:
max-size: "10m"
max-file: "3"
Supported Logging Drivers:
json-file
: Default driver, stores logs locally as JSON.syslog
: Sends logs to a syslog server.journald
: Integrates with systemd.fluentd
: Streams logs to Fluentd.
Each driver offers unique advantages and trade-offs. For instance, fluentd
is useful for centralized logging in kubernetes
or large-scale environments. Choose one based on your environment and requirements.
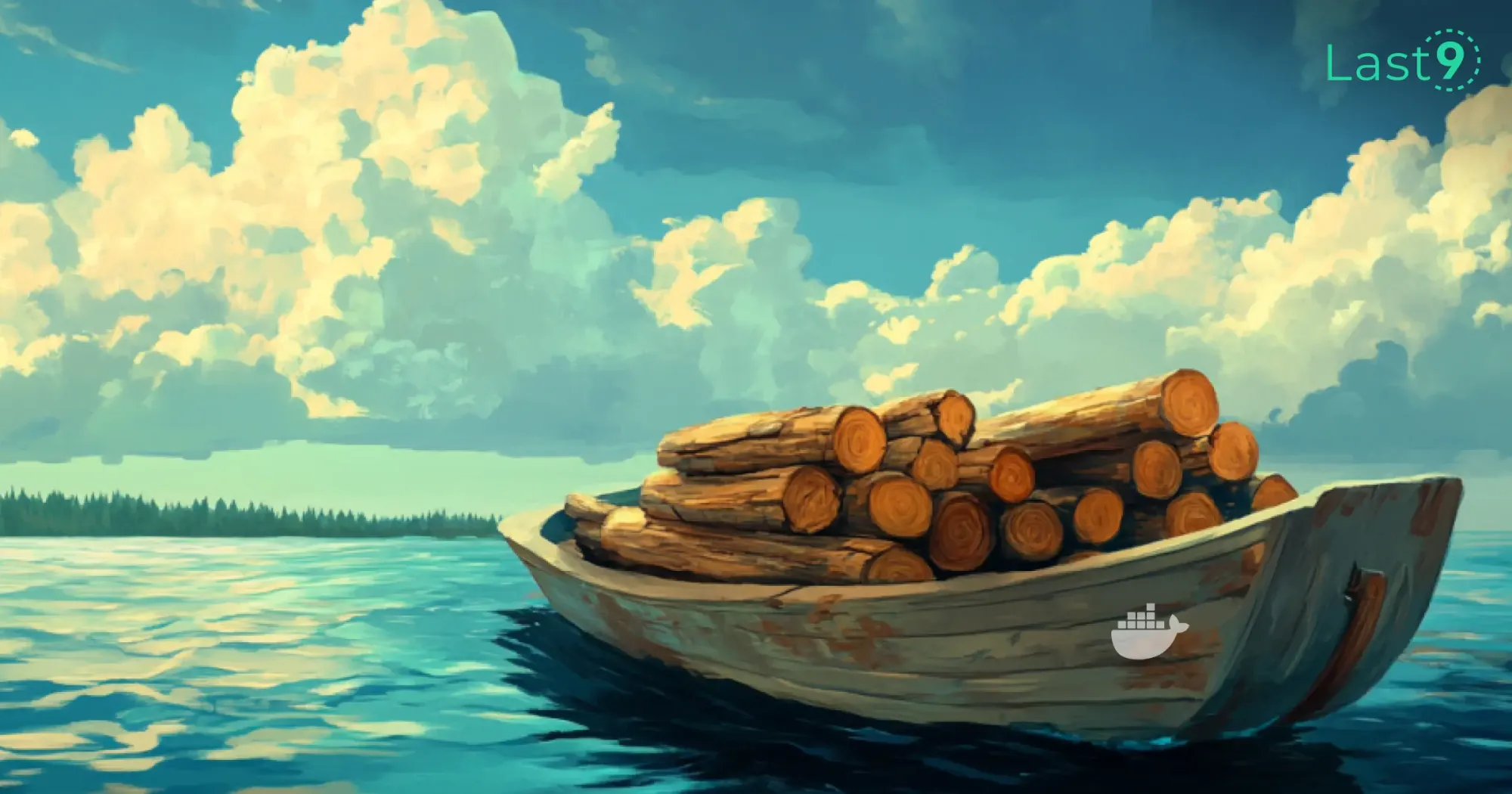
Managing Container Logs Effectively
Implement Log Rotation
Prevent disk space issues by configuring log rotation. For example:
logging:
driver: "json-file"
options:
max-size: "10m"
max-file: "3"
Use Centralized Logging
Tools like Elasticsearch, Fluentd, Kibana (EFK), or Grafana Loki can aggregate and visualize logs from multiple containers for a more comprehensive analysis. Centralized logging is critical for DevOps teams monitoring distributed systems.
Monitor Log Volume
High log volumes can affect application performance. Use delivery modes like non-blocking to mitigate this risk:
logging:
options:
mode: "non-blocking"
Additionally, monitor directories like /var/lib/docker
to ensure your system doesn’t run out of space.
Debugging and Troubleshooting
Common Scenarios:
- Application Errors: Look for error logs in the
stderr
stream to identify issues with your app. - Service Failures: Check for logs during container initialization to pinpoint configuration issues.
- Networking Problems: Logs can reveal if services fail to connect due to network misconfiguration.
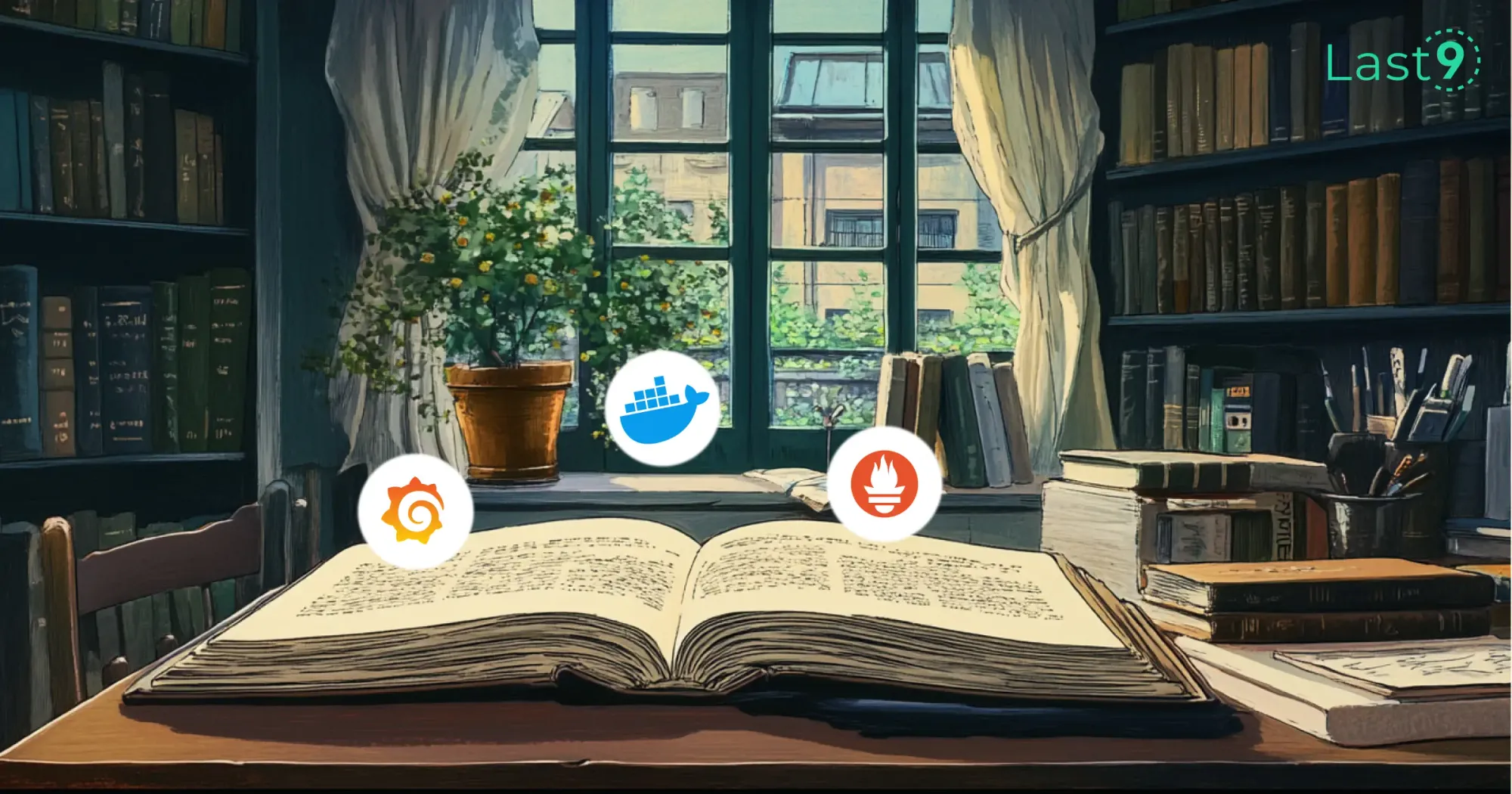
Using Filters
If logs become overwhelming, use the grep
command to filter entries:
docker-compose logs | grep "ERROR"
Or specify a particular service to narrow the scope:
docker-compose logs <service-name> | grep "connection"
Filtering log output is especially useful in large multi-container applications where individual service logs can quickly grow unwieldy.
Advanced Techniques
Collecting Logs via API
Docker API provides endpoints for fine-grained control over log collection. For example, to retrieve logs in real-time:
curl --unix-socket /var/run/docker.sock \
http://v1.40/containers/<container_id>/logs?stdout=true&stderr=true&follow=true
Sidecar Containers for Logging
A sidecar container dedicated to logging can centralize log management. For example, when working with redis
or postgresql
, sidecars can route log files to centralized systems:
logging:
driver: "fluentd"
options:
fluentd-address: "localhost:24224"
Sidecar configurations also streamline log outputs for tools like Prometheus or open-source log visualization platforms.
Integrating Logs with GitHub Actions
To enhance CI/CD pipelines, logs can be integrated with GitHub Actions. Use the following configuration file to capture logs during build or deployment:
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v3
- name: Build and run containers
run: |
docker-compose up -d
docker-compose logs --tail=50 > logs.txt
- name: Upload Logs
uses: actions/upload-artifact@v2
with:
name: container-logs
path: logs.txt
This approach makes log files available for post-build analysis.
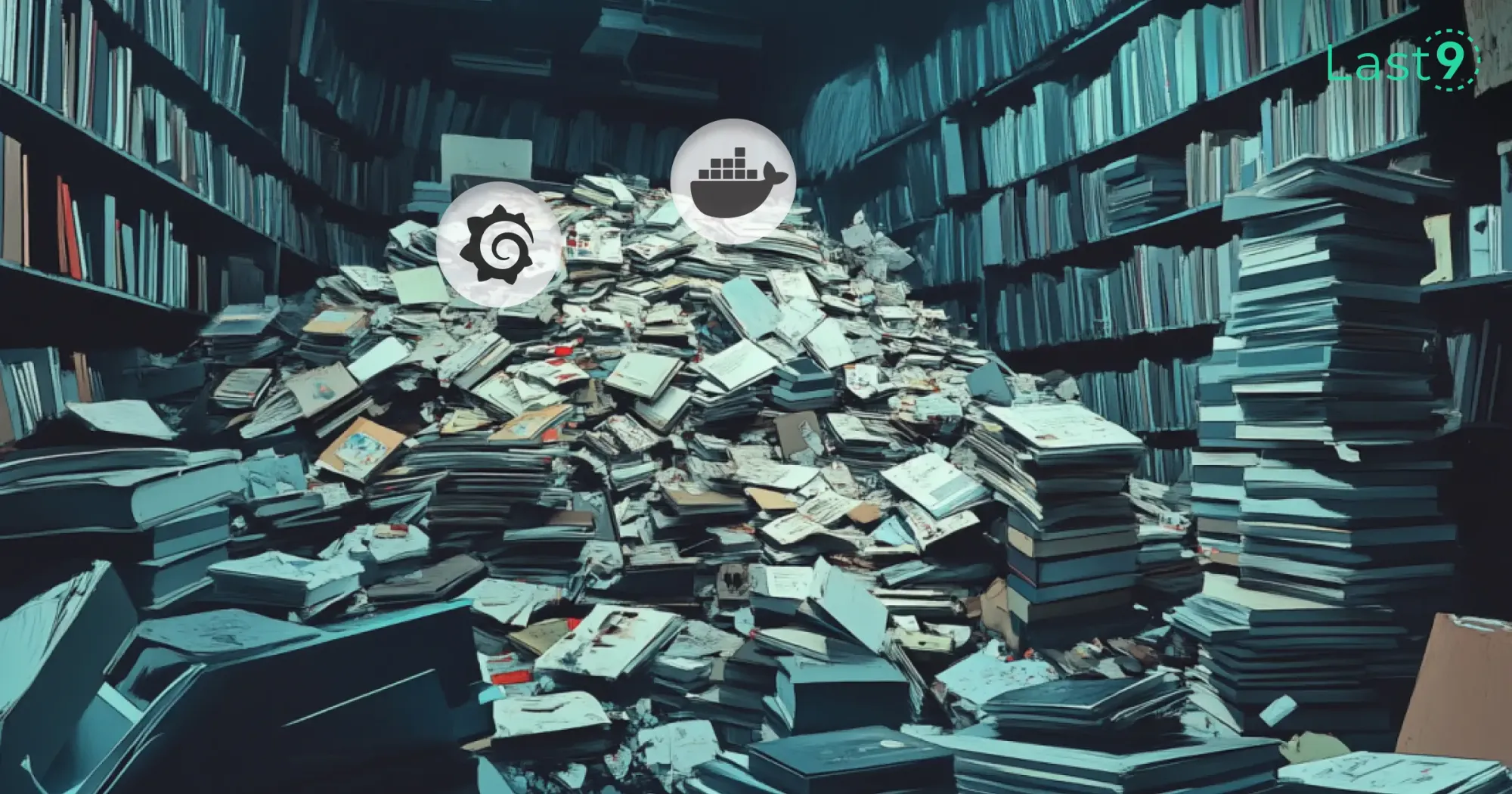
Example Dockerfile for Enhanced Logging
Here’s an example of a Dockerfile
for a Python web server with enhanced logging:
FROM python:3.9
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
ENV FLASK_APP=app.py
ENV FLASK_ENV=development
CMD ["flask", "run", "--host=0.0.0.0"]
Logs from this web server can be routed via docker-compose.yml
to a centralized system using Fluentd or similar plugins.
Conclusion
Docker Compose logs are a cornerstone of effective containerized application management.Combine the Docker Compose logs command with centralized tools and CI/CD integrations to make container logs a strategic asset.
FAQs
How to see logs in Docker Compose?
To view logs in Docker Compose, use the docker-compose logs
command. Add options like --follow
to stream logs in real-time or specify a service name to view logs for a particular container.
Where does Docker Compose store log files?
Docker Compose logs are stored in the same location as Docker container logs, usually under /var/lib/docker/containers/<container_id>/
. The format depends on the logging driver specified in the configuration file.
How can I check Docker logs?
To check logs for a specific Docker container, use the docker logs <container_id>
command. You can also use docker-compose logs
for multi-container setups.
How do you save Docker Compose logs?
To save Docker Compose logs to a file, redirect the log output using the following command:
docker-compose logs > compose-logs.txt
How to tail Docker Compose log?
To tail Docker Compose logs in real-time, use the --follow
option:
docker-compose logs --follow
What is TTY in Docker Compose?
TTY
(teletypewriter) in Docker Compose refers to enabling or disabling the pseudo-TTY allocated to the container. This is configured in the docker-compose.yml
file under the tty
option.
How do I view logs for a specific Docker container?
Use the docker-compose logs <service-name>
command to view logs for a specific container managed by Docker Compose.
How do I see the console of a Docker container?
To access the console or shell of a running container, use the docker exec -it <container_id> /bin/bash
command.
Why are Docker Compose logs important?
Logs help diagnose issues, monitor system behavior, and ensure smooth operation of containerized applications. They are essential for debugging and maintaining application performance.
How can I change the logging driver?
Modify the logging driver in the docker-compose.yml
file under the logging
key. For example:
logging:
driver: "fluentd"
How to Remove a Docker Image?
To remove a Docker image, use the docker rmi <image_id>
command. Ensure no containers are using the image before removing it.
How do I filter Docker Compose logs by service name?
Use the docker-compose logs <service-name>
command to filter logs by service name.
How do I filter logs by service in Docker Compose?
Specify the service name when running the docker-compose logs
command:
docker-compose logs <service-name>
Can I customize log output formats?
Yes, the log format can be customized using logging drivers and their associated options in the docker-compose.yml
file.
How to handle large log files?
Implement log rotation in the docker-compose.yml
file using options like max-size
and max-file
under the logging
key.
What are the common logging drivers?
The most common drivers include json-file
, syslog
, journald
, and fluentd
. Each driver serves specific use cases, like local storage or centralized logging.
How to inspect logs for a failed container?
For failed containers, use the docker logs <container_id>
or docker-compose logs <service-name>
commands to inspect logs and diagnose the issue.
How do I set environment variables for logging?
Environment variables can be configured in the docker-compose.yml
file under the environment
key. For example:
environment:
LOG_LEVEL: "debug"
Contents
Newsletter
Stay updated on the latest from Last9.
Helping to make the tech a little less intimidating. I love breaking down complex concepts into easy-to-understand terms.
Handcrafted Related Posts
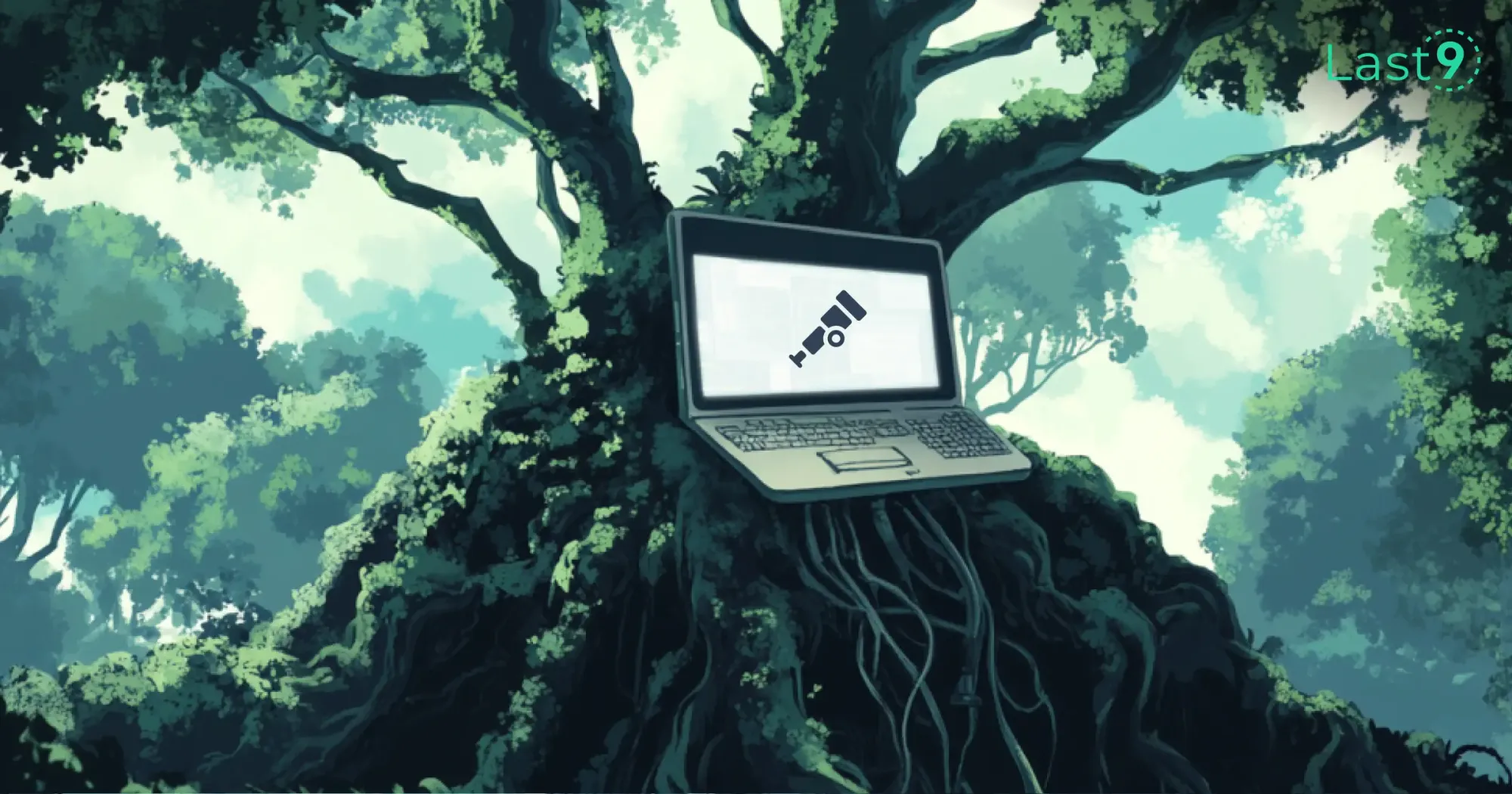
Identify Root Spans in Otel Collector
How to identify root spans in OpenTelemetry Collector using filter and transform processors
Prathamesh Sonpatki
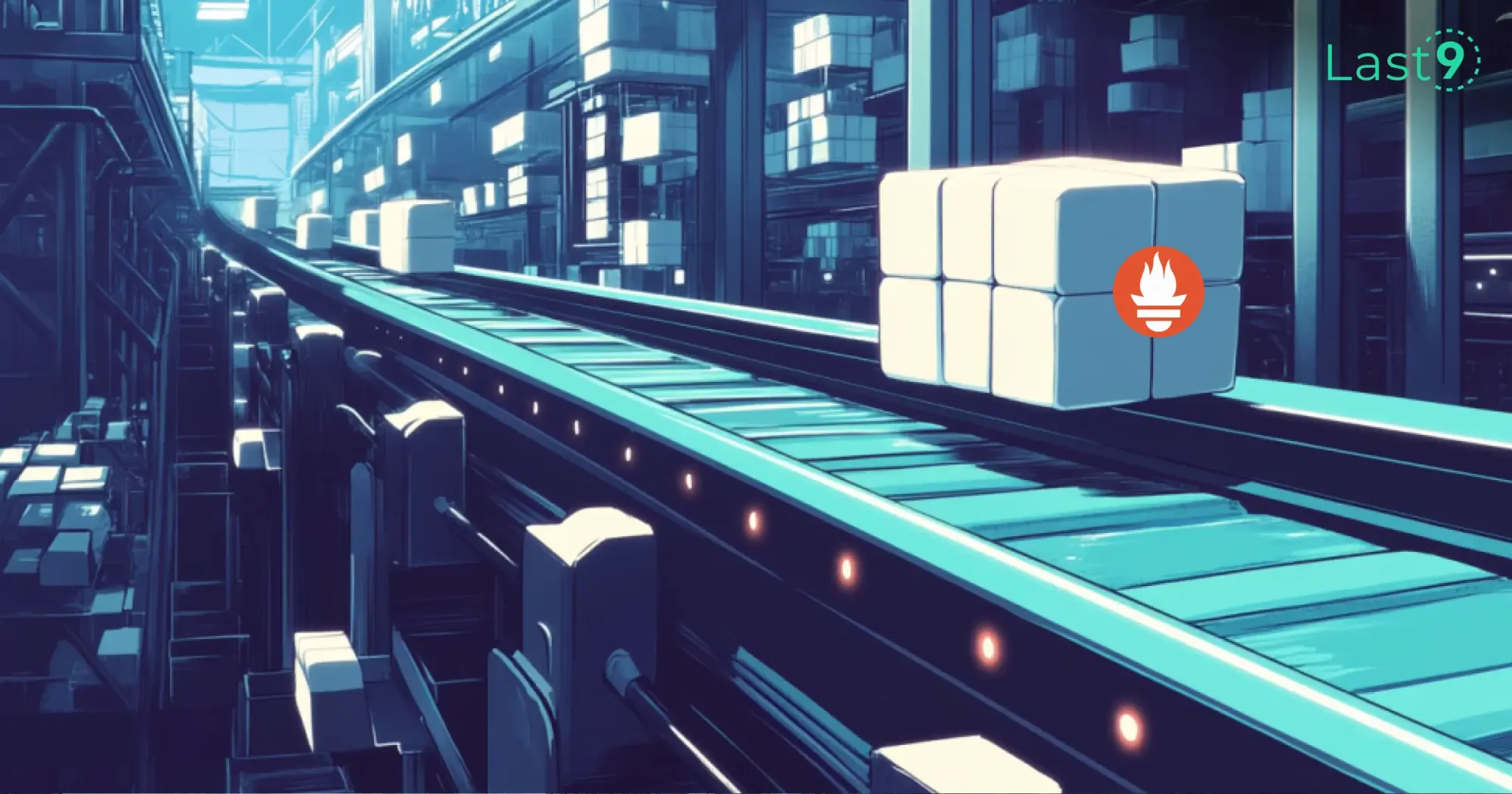
Optimizing Prometheus Remote Write Performance: Guide
Master Prometheus remote write optimization. Learn queue tuning, cardinality management, and relabeling strategies to scale your monitoring infrastructure efficiently.
Gabriel Diaz
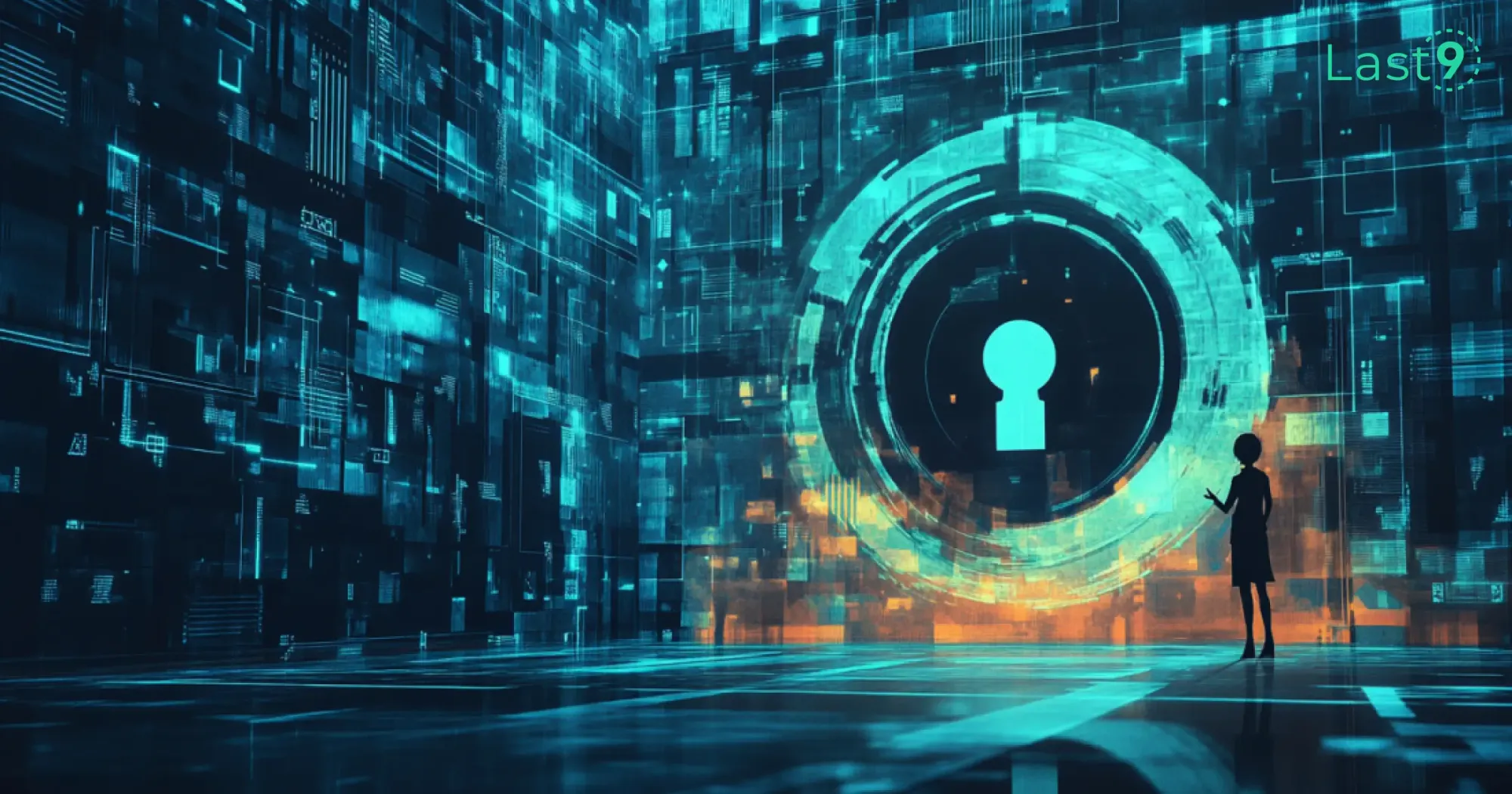
Redacting Sensitive Data in OpenTelemetry Collector
This guide covers types of data that can be redacted and step-by-step instructions for configuring the Attribute Processor.
Ujjwal Goyal