Docker containers generate a massive amount of logs, and if left unchecked, they can quickly fill up your disk space. Whether you're new to containerization or managing large-scale deployments, keeping logs under control is essential for performance and stability.
Here’s a practical guide on how to manage and clear Docker logs effectively—no unnecessary jargon, just straightforward steps you can use right away.
Understanding Docker Logs and Storage Issues
Docker captures stdout and stderr output from your containers and stores them as JSON files. These logs accumulate over time, and here's the kicker – Docker has no built-in log rotation mechanism by default.
Think of it like your kitchen trash – if you never take it out, eventually you'll be eating dinner on top of a garbage mountain. Docker logs work the same way.
Common culprits behind rapidly expanding logs:
- Verbose applications that log every minor event
- Debug-level logging in production
- High-traffic applications
- Applications with chatty dependencies
- Improperly configured logging drivers
How to Monitor Docker Log Space Consumption
Before clearing logs, it's important to check how much space they are consuming. Large log files can quickly lead to storage issues, affecting performance and stability.
Here’s how to monitor Docker log space usage:
Checking Log File Sizes
To see the size of all container logs, run:
sudo du -h /var/lib/docker/containers/*/*-json.log | sort -h
What This Does:
du -h
(disk usage) displays file sizes in a human-readable format.- The
sort -h
command sorts the output by size. - This helps identify which containers have oversized logs that may require cleanup.
If you notice logs in the hundreds of MBs or even GBs, it's time to take action.
Identifying Containers with Large Log Usage
For a quick overview of which containers are consuming the most space, use:
docker ps --size
Understanding the Output:
- The
SIZE
column in the output shows each container's writable layer size. - This includes log files, temporary files, and other changes made since the container was started.
- A container with an unusually high size might have excessive log accumulation.
Why Monitoring Log Size Matters
- Prevents disk space exhaustion, which can cause system instability.
- Helps identify misconfigured logging settings that generate excessive data.
- Enables proactive log rotation and cleanup to maintain efficient operations.
By regularly checking Docker log sizes, you can ensure that your system remains performant and avoid unexpected storage issues.
Practical Methods for Clearing Docker Logs
Let's get to the meat of it – how to clear those Docker logs. I've got several approaches for you, ranging from quick fixes to proper long-term solutions.
Quick Truncate Approach for Instant Space Recovery
When you need to clear logs fast without disrupting services, this one-liner is your new best friend:
sudo sh -c 'truncate -s 0 /var/lib/docker/containers/*/*-json.log'
This command zeroes out all container log files without deleting them, keeping your containers happy while reclaiming that precious disk space.
Targeted Clearing for Specific Container Logs
Need a more surgical approach? You can target specific containers:
docker inspect --format='{{.LogPath}}' CONTAINER_ID | xargs truncate -s 0
Just replace CONTAINER_ID
with your container's ID or name. Perfect for when you have that one verbose container that's causing all the trouble.
Docker Compose Solution for Complete Log Removal
If you're using Docker Compose and want a fresh start:
docker-compose down
docker-compose rm -v
This stops your services and removes containers, which eliminates their logs. Just remember to back up any data you need before running this!
Log Driver Configuration for Preventing Storage Issues
Clearing logs is great, but preventing buildup is even better. Let's set up proper log management to avoid midnight disk space emergencies.
Docker Daemon Configuration for Automatic Log Rotation
Edit your Docker daemon configuration file (usually at /etc/docker/daemon.json
):
{
"log-driver": "json-file",
"log-opts": {
"max-size": "10m",
"max-file": "3"
}
}
This configuration:
- Limits each log file to 10MB
- Keeps a maximum of 3 rotated files per container
- Uses the default json-file driver
After saving, restart Docker with:
sudo systemctl restart docker
Container-Specific Log Management Settings
For more granular control, you can configure logging per container:
docker run --log-driver json-file --log-opt max-size=10m --log-opt max-file=3 your-image
Or in docker-compose.yml:
services:
your-service:
image: your-image
logging:
driver: "json-file"
options:
max-size: "10m"
max-file: "3"
3 Common Docker Log Problems and Solutions
Even with the best setups, log issues happen. Here's how to handle common problems:
Resolving Missing Container Log Access
If docker logs
suddenly returns nothing after clearing logs, don't panic. This usually happens because the log file was deleted instead of truncated.
The fix:
- Restart the affected container
- Always use
truncate -s 0
instead ofrm
on the log files
Troubleshooting Continuous Log Growth Issues
If your logs continue expanding despite your max-size settings, check that:
- You've restarted Docker after changing daemon.json
- Your containers were created after the configuration change (existing containers won't adopt new log settings)
- You're not using a different logging driver that ignores these settings
Recovering Disk Space When Log Clearing Fails
Sometimes clearing logs doesn't seem to free up space. This often happens because:
- The files are still in use by processes
- You're looking at cached disk usage information
Try running:
sudo sync && sudo sh -c 'echo 3 > /proc/sys/vm/drop_caches'
Then check disk usage again.
5 Enterprise-Grade External Logging Platforms
For production environments, relying on external logging solutions is a smarter way to manage logs at scale. Storing logs externally keeps your Docker hosts from filling up and provides better tools for searching, analyzing, and alerting on critical events.
Solution | Best For | Features |
---|---|---|
Last9 | High-cardinality observability unifying logs, metrics and traces | Connects logs with metrics and traces, embedded Grafana dashboards, time-series analysis, cost-efficient storage, alerting |
ELK Stack (Elasticsearch, Logstash, Kibana) | Large deployments with heavy log volumes | Full-text search, visualization, alerting, customizable pipelines |
Fluentd | Kubernetes and cloud-native environments | Unified logging, flexible routing, support for multiple outputs |
Graylog | Security-focused teams | SIEM capabilities, compliance reporting, efficient log storage |
Loki | Grafana users needing lightweight log storage | Label-based search, cost-effective, integrates seamlessly with Grafana dashboards |
Why Use External Logging?
- Prevents disk space issues: Keeps your Docker hosts from getting clogged with logs.
- Advanced search and filtering: Find what matters without sifting through endless raw logs.
- Better observability: Correlate logs with system performance and user behavior.
- Alerting and automation: Get notified when patterns or anomalies emerge.
Among these options, Last9 stands out for its ability to correlate logs, metrics, and traces in high-cardinality environments, along with embedded Grafana dashboards and built-in alerting for a more simplified monitoring experience.
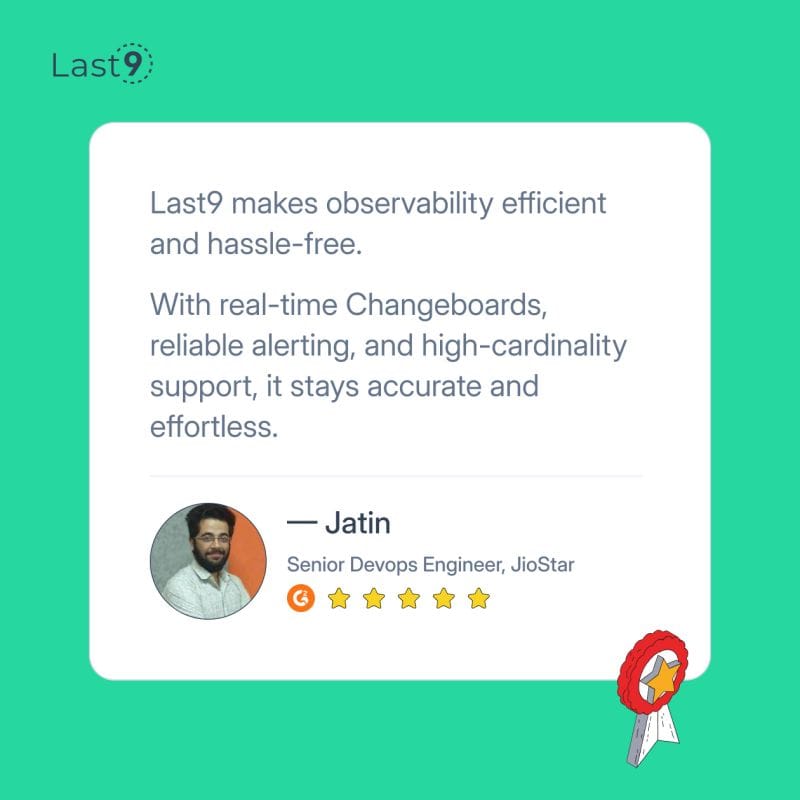
Choosing the right platform depends on your use case—whether you need full-text search (ELK), lightweight log management (Loki), or compliance-focused monitoring (Graylog). Whatever you pick, moving logs to an external system will save resources and give you better visibility into your infrastructure.
How to Automate Log Management in CI/CD Workflows
Integrating log management into your CI/CD pipeline helps prevent performance issues before they reach production. By automating log cleanup, you can keep disk usage under control and ensure that logs don't pile up unnecessarily, slowing down builds and deployments.
Here’s how to integrate log management in two popular CI/CD systems:
Automating Log Cleanup in Jenkins
If you're using Jenkins, you can add a dedicated stage to your pipeline that clears old logs and removes unused Docker resources.
Jenkins Pipeline Example:
pipeline {
agent any
stages {
stage('Clean Logs') {
steps {
sh 'docker system prune -f'
sh 'truncate -s 0 /var/lib/docker/containers/*/*-json.log'
}
}
}
}
What This Does:
docker system prune -f
removes unused containers, networks, and images, keeping your CI environment clean.truncate -s 0 /var/lib/docker/containers/*/*-json.log
clears existing container logs without deleting the files, preventing disk bloat.
Automating Log Cleanup in GitHub Actions
For GitHub Actions, you can include a step in your workflow to automatically clear logs and remove unnecessary Docker artifacts after each build.
GitHub Actions Workflow Example:
name: CI Cleanup
on:
push:
branches:
- main
jobs:
cleanup:
runs-on: ubuntu-latest
steps:
- name: Clean Docker logs
run: |
sudo truncate -s 0 /var/lib/docker/containers/*/*-json.log
docker system prune -f
What This Does:
- Runs automatically when changes are pushed to the
main
branch. - Clears all container logs to free up disk space.
- Prunes unnecessary Docker resources, keeping the CI environment lightweight.
Why Automate Log Management in CI/CD?
- Prevents disk space issues: Logs can quickly fill up a CI/CD runner or build server, causing failures.
- Keeps builds efficient: Clearing unnecessary logs reduces overhead and improves build times.
- Reduces manual maintenance: No need to manually SSH into servers to clean up logs.
- Improves reliability: Ensures log files don’t consume excessive storage and slow down deployments.
Best Practices for Ongoing Docker Log Management
Managing Docker logs doesn't have to be complicated. With the right configuration and occasional maintenance, you can keep your containers running smoothly and your disk space under control.
Remember these key takeaways:
- Check log sizes regularly
- Set up log rotation from the start
- Use
truncate -s 0
for immediate relief - Consider external logging for production
FAQs
How often should I clear Docker logs?
There's no one-size-fits-all answer, but monitoring log sizes weekly is a good starting point. For high-traffic production environments, consider setting up automated log rotation and clearing. If your disk usage jumps above 80%, that's a good indicator it's time to clear logs.
Will clearing logs affect my running containers?
When using the truncate method (truncate -s 0
), your containers will continue running without interruption. The container's logging process simply starts writing to the now-empty file. However, if you delete log files entirely, containers may lose their logging ability until restarted.
Can I recover Docker logs after clearing them?
Once logs are truncated or deleted, that data is gone. This is why proper log forwarding to external systems like ELK Stack or Loki should be implemented before clearing logs in production environments.
Why does Docker store logs as JSON files?
Docker uses JSON file format for logs because it provides a structured way to store both the log message and metadata like timestamps. This makes logs more easily parsable by automated tools and compatible with many logging systems.
Is there a way to disable logging for certain containers?
Yes, you can set the logging driver to "none" when running a container:
docker run --log-driver none your-image
This completely disables logging for that container, which is useful for containers that generate excessive, unnecessary logs.
Does Docker have built-in log compression?
Docker itself doesn't compress logs by default. However, you can use logging drivers like "local" which support compression:
{
"log-driver": "local",
"log-opts": {
"compress": "true",
"max-size": "10m"
}
}
What permissions do I need to clear Docker logs?
On most systems, you'll need root or sudo privileges to access and modify files in the Docker directory. This is why many of the commands in this guide use sudo
.