Logging is an essential part of any web framework, and Django is no exception. It helps track events, debug issues, and monitor your application's performance.
However, Django's default logging setup might not work for every project right out of the box. In this guide, we'll explore how to set up and configure Django logging, handle logs in various formats (like JSON), and improve your logging strategies to enhance monitoring.
What is Django Logging?
Django logging is built on Python’s robust logging module, which offers a flexible framework for emitting log messages.
These logs can be sent to various outputs such as the console, log files, or even remote servers for centralized logging.
A typical log record in Django includes several details, like the log level (INFO, DEBUG, etc.), timestamp, the actual log message, and more.
While Django’s logging system isn’t pre-configured for all use cases, Python's logging module allows for easy customization to fit your needs.
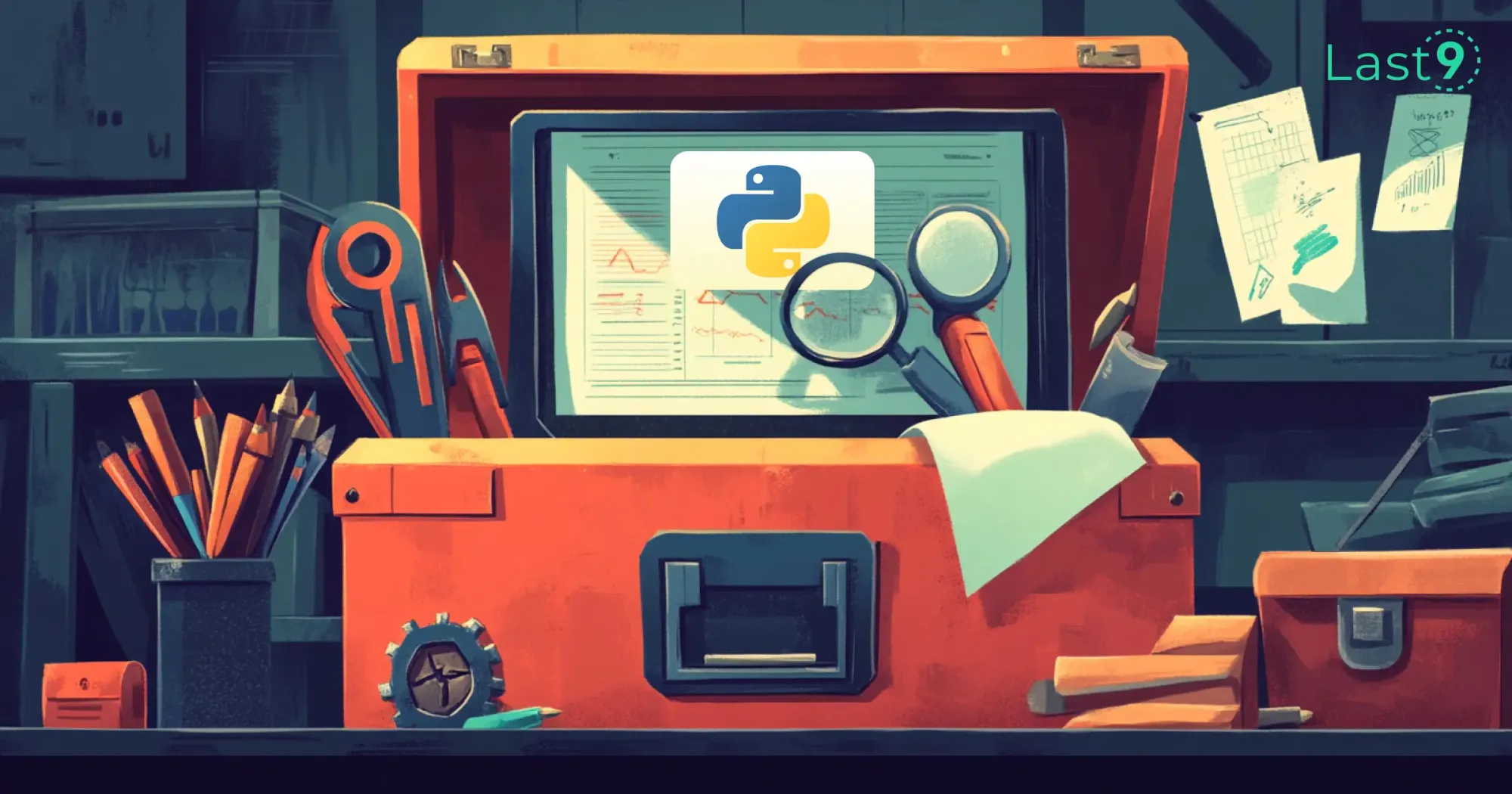
Setting Up Logging in Django
Configuring Django Logging in settings.py
In Django, logging is usually configured in the settings.py
file, where you define loggers, handlers, and formatters.
By default, Django logs errors to the console and sends them to the development server logs (via the django.server
logger).
Here’s an example of a logging configuration:
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'formatters': {
'verbose': {
'format': '{levelname} {asctime} {module} {message}',
'style': '{',
},
'simple': {
'format': '{levelname} {message}',
'style': '{',
},
},
'handlers': {
'console': {
'level': 'DEBUG',
'class': 'logging.StreamHandler',
'formatter': 'simple',
},
'file': {
'level': 'ERROR',
'class': 'logging.FileHandler',
'filename': 'error.log',
'formatter': 'verbose',
},
},
'loggers': {
'django': {
'handlers': ['console'],
'level': 'INFO',
'propagate': True,
},
'django.server': {
'handlers': ['console'],
'level': 'ERROR',
'propagate': False,
},
'myapp': {
'handlers': ['file'],
'level': 'ERROR',
'propagate': False,
},
},
}
This setup defines both console and file handlers:
- The
django.server
logger outputs error-level messages to the console. - Custom logs from
myapp
are written to a file (error.log
), making it easier to separate logs for better management in production.
You can further tailor this configuration by specifying additional loggers, adjusting log levels, or defining custom file names for logs based on your project’s requirements.
The Role of Handlers and Formatters
In Django’s logging configuration, handlers and formatters are key components:
- Handlers decide where the log messages go (e.g., console, files, or remote services).
- Formatters define the structure of the log messages, letting you include details like timestamps, log levels, or the logger name.
For example, adding a timestamp to your logs can help you track when events occurred. If you want your logs in JSON format (useful for tools like Logstash or Kibana), you can configure a JSON formatter like this:
'json': {
'format': '{"timestamp": "%(asctime)s", "level": "%(levelname)s", "message": "%(message)s"}',
'style': '%',
},
This setup outputs log messages as JSON strings, making them easier to process in modern log analysis systems.
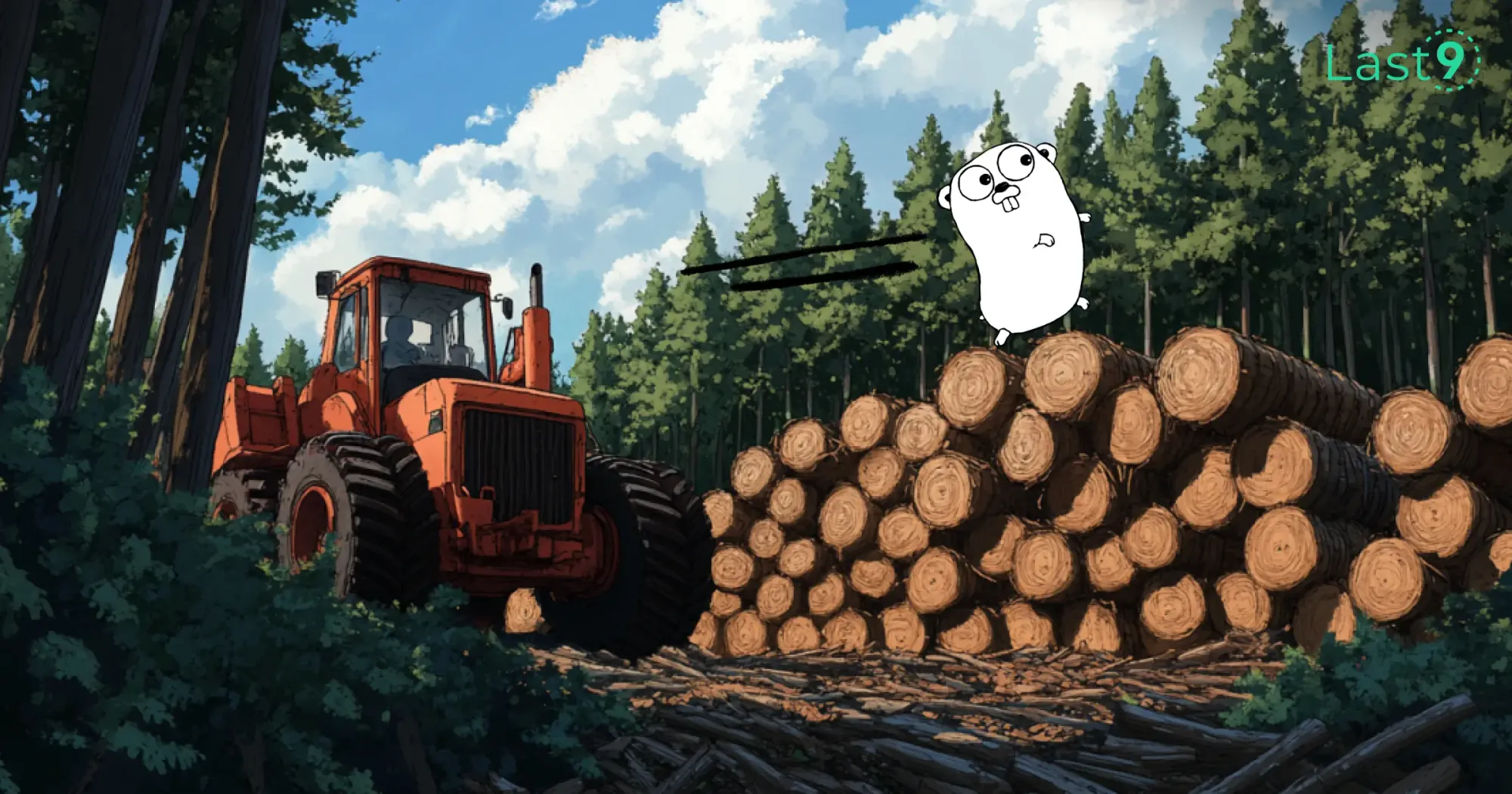
Log Levels
Log levels determine the severity of log messages. Django supports several levels, including:
- DEBUG: Detailed information, typically useful during development.
- INFO: General operational messages.
- WARNING: Indications of potential problems.
- ERROR: Errors that have occurred.
- CRITICAL: Severe issues requiring immediate attention.
For example, in development, you might use the DEBUG level to capture everything for troubleshooting. In contrast, production environments often use ERROR or CRITICAL levels to focus on significant issues without cluttering the logs.
Advanced Django Logging Configuration
Using dictConfig
for More Complex Logging Configurations
For more advanced logging setups, you can use Python’s logging.config.dictConfig
, which allows you to configure logging using Python dictionaries. This is especially useful when you need to programmatically adjust logging settings in larger projects.
Here’s an example of how to use dictConfig
:
import logging.config
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'formatters': {
'detailed': {
'format': '%(asctime)s %(name)s %(levelname)s %(message)s',
},
},
'handlers': {
'file': {
'level': 'ERROR',
'class': 'logging.FileHandler',
'filename': 'django_error.log',
'formatter': 'detailed',
},
},
'loggers': {
'django': {
'handlers': ['file'],
'level': 'ERROR',
'propagate': False,
},
},
}
logging.config.dictConfig(LOGGING)
This approach allows you to easily programmatically configure logging, making it a great choice for larger projects or when you want to centralize logging configurations in a specific module.
Handling Server Logs
Django provides a dedicated logger for server-related logs: django.server
. This logger captures requests and their associated errors, which is critical for monitoring your application’s health.
To capture detailed server logs, you can configure this logger with a handler, such as a console or a file handler, to save logs for later analysis. This helps you keep track of critical errors during development while using the runserver
command.
Here’s how you can configure the django.server
logger for development:
'handlers': {
'console': {
'level': 'DEBUG',
'class': 'logging.StreamHandler',
},
},
'loggers': {
'django.server': {
'handlers': ['console'],
'level': 'ERROR',
'propagate': False,
},
},
This configuration ensures that only necessary error messages are logged during development, keeping the console output clean and manageable.
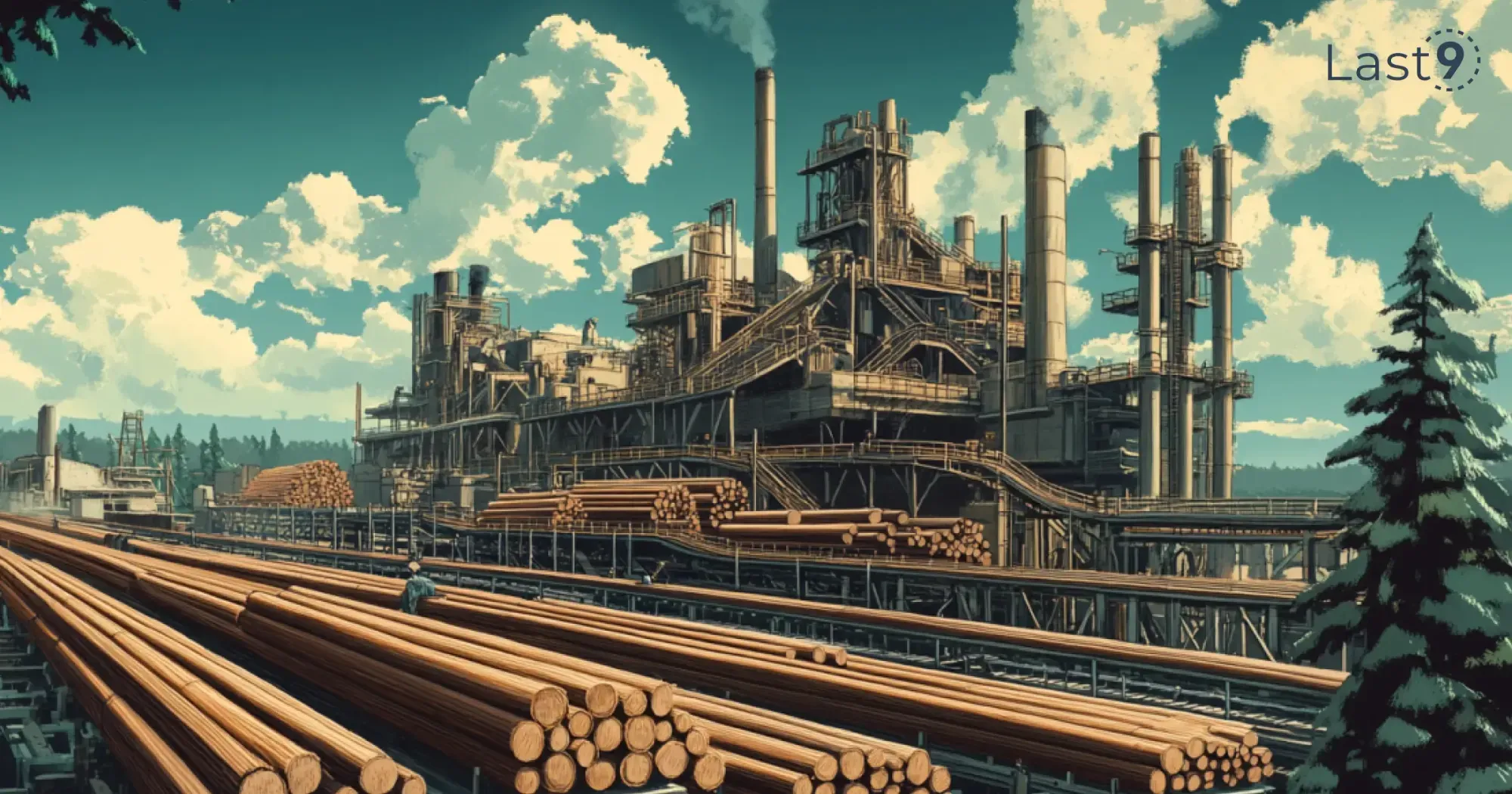
Logging API Calls
If you're working with APIs, especially those built with Django Rest Framework (DRF), logging all incoming requests can be valuable for monitoring and troubleshooting. Django logging can be integrated into DRF by overriding views or middleware to log requests.
Here's an example of how you can log API calls and their responses using middleware:
import logging
logger = logging.getLogger(__name__)
class APILoggingMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
logger.info(f"Request: {request.method} {request.path}")
response = self.get_response(request)
logger.info(f"Response: {response.status_code}")
return response
This middleware logs the HTTP method and path of incoming requests, as well as the status code of the response.
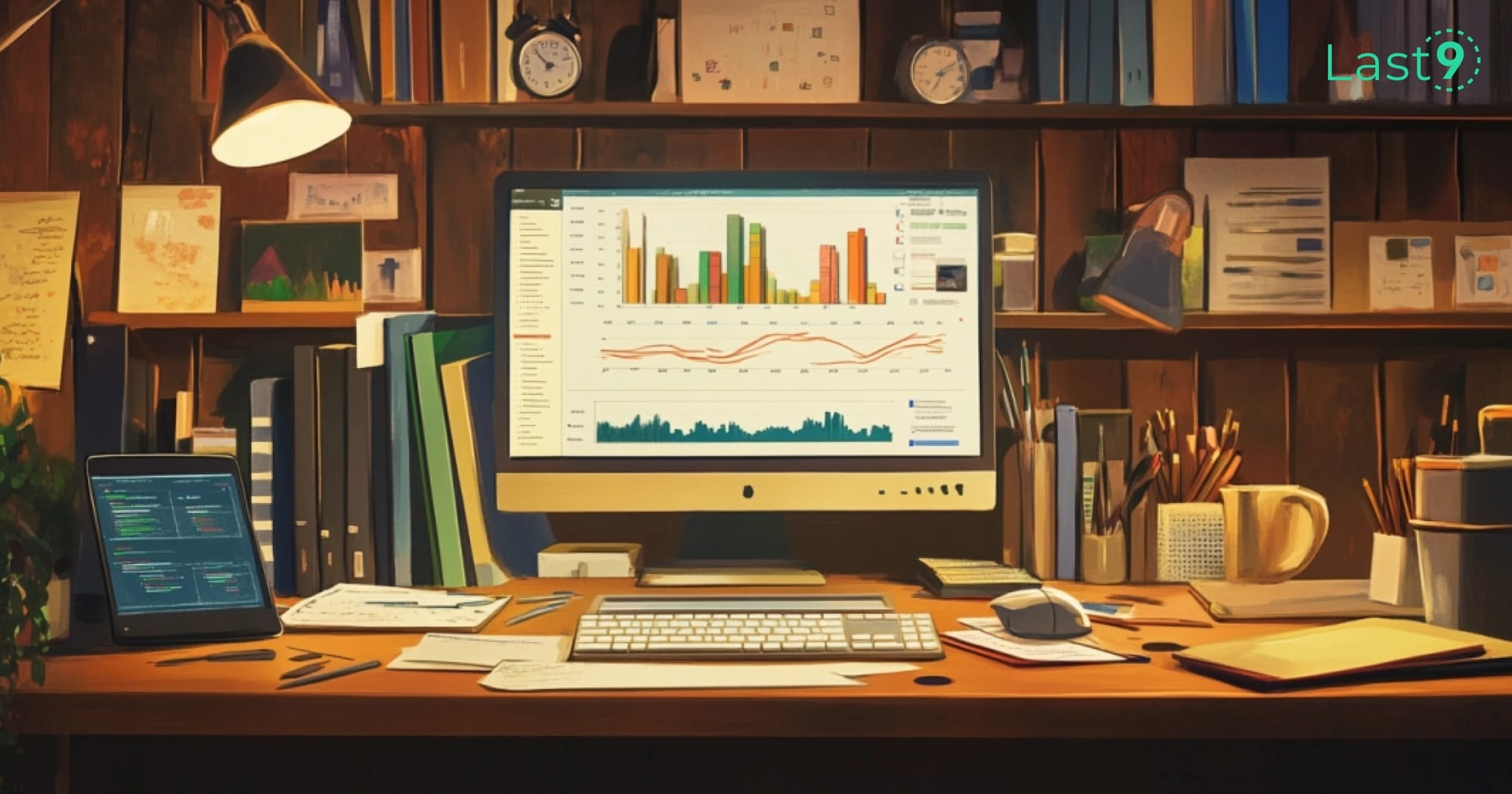
Conclusion:
Django’s flexible logging configuration provides all the tools you need to control how logs are captured, formatted, and stored.
Configuring logging in settings.py, using various log levels, and using advanced configurations like dictConfig ensures that your logs are detailed, readable, and organized. Don't forget to set up handlers for real-time monitoring, file-based logs, and even error emails to admins if something goes wrong in production.
When combined with tools like Sentry and OpenTelemetry, Django’s logging system can provide robust monitoring and offer valuable insights into your web application’s performance.
Happy logging and debugging with Django!
FAQs
What is logging in Django?
Logging in Django helps developers track and manage log records, capture exceptions, and monitor their application’s performance.
How do I configure Django to write logs to a file?
You can configure Django logging by specifying a FileHandler
in the LOGGING
dictionary in settings.py
. This will direct logs to a specific log file.
How can I configure Django to send error emails to admins?
Django has an AdminEmailHandler
that can be configured to send error logs via email to administrators when errors occur in production.
What is the logging.config
module used for?
The logging.config
module is used to configure logging from dictionaries or configuration files, offering more flexibility and customization for complex logging setups.
How do I configure Django's logging to output to a file?
To configure Django to log output to a file, you can set up theFileHandler
logging configuration in settings.py
. This handler will log messages to a specific file, such as django.log
, and you can specify the log level and formatting for the messages.
What are the different logging levels in Django?
Django supports several logging levels, including DEBUG
, INFO
, WARNING
, ERROR
, and CRITICAL
. These levels help control the verbosity of the logs. For instance, DEBUG
logs detailed information, while ERROR
logs only critical issues.
How do I set up Django logging to capture server errors?
Django logs server errors using the django.server
logger. You can configure it in settings.py
to capture server logs at the ERROR
level and direct them to the console or a file for easier monitoring.
How to log all API calls in a Django application?
To log all API calls in a Django application, you can implement custom logging in views or use middleware. By logging the request and response data, you can easily track all incoming API requests.
What is OpenTelemetry, and how does it relate to Django logging?
OpenTelemetry is an open-source observability framework that provides instrumentation for monitoring applications. Integrating OpenTelemetry with Django allows you to collect traces and metrics, alongside traditional logging, to get a more complete picture of your application’s performance and behavior.
How do I configure Django to send error logs via email?
Django can be configured to send error logs via email by using AdminEmailHandler
in the logging settings. This handler will email the log messages to the site’s admins when critical errors occur.
How do I set up JSON logs in Django?
To configure Django to output logs in JSON format, you can define a custom formatter in the logging settings. This is especially useful if you plan to aggregate logs for analysis in tools like Elasticsearch or Kibana.
What is the dictConfig
method in Django logging?dictConfig
is a method provided by the logging module that allows for logging configuration using dictionaries. It offers greater flexibility and is useful for more complex logging setups compared to the standard LOGGING
dictionary configuration.
How to log exceptions in Django?
Django’s logger allows you to log exceptions logger.exception()
when an exception is caught. This captures the stack trace along with the log message, which is useful for troubleshooting errors.
How do I configure Django logging for real-time analysis?
For real-time log analysis, you can configure logging to output logs to external services like Sentry or Loggly. Additionally, using tools like OpenTelemetry can help capture performance metrics and traces alongside logs for comprehensive monitoring.