Picture an e-commerce platform during a flash sale. Customers are placing orders, but some face delays or payment failures. With multiple microservices handling tasks like authentication, inventory, and payments, pinpointing the root cause can be challenging.
This is where distributed tracing becomes essential. It gives you a clear view of how requests move through your system, making it easier to identify bottlenecks or errors and ensure a smoother customer experience.
Understanding Spans and Trace Structure
Before we get into enhancing traces, let’s review the building blocks of distributed tracing.
The core components are traces and spans:
Trace
A trace represents the entire journey of a request as it travels across various services in your system.
Span
A span is a single unit of work in that journey, capturing the start and end of an operation (such as a request to a service or database).
Each span contains metadata about the operation it represents, such as its start time, end time, and relationships to other spans. These building blocks allow you to follow the path of a request as it moves through the system.
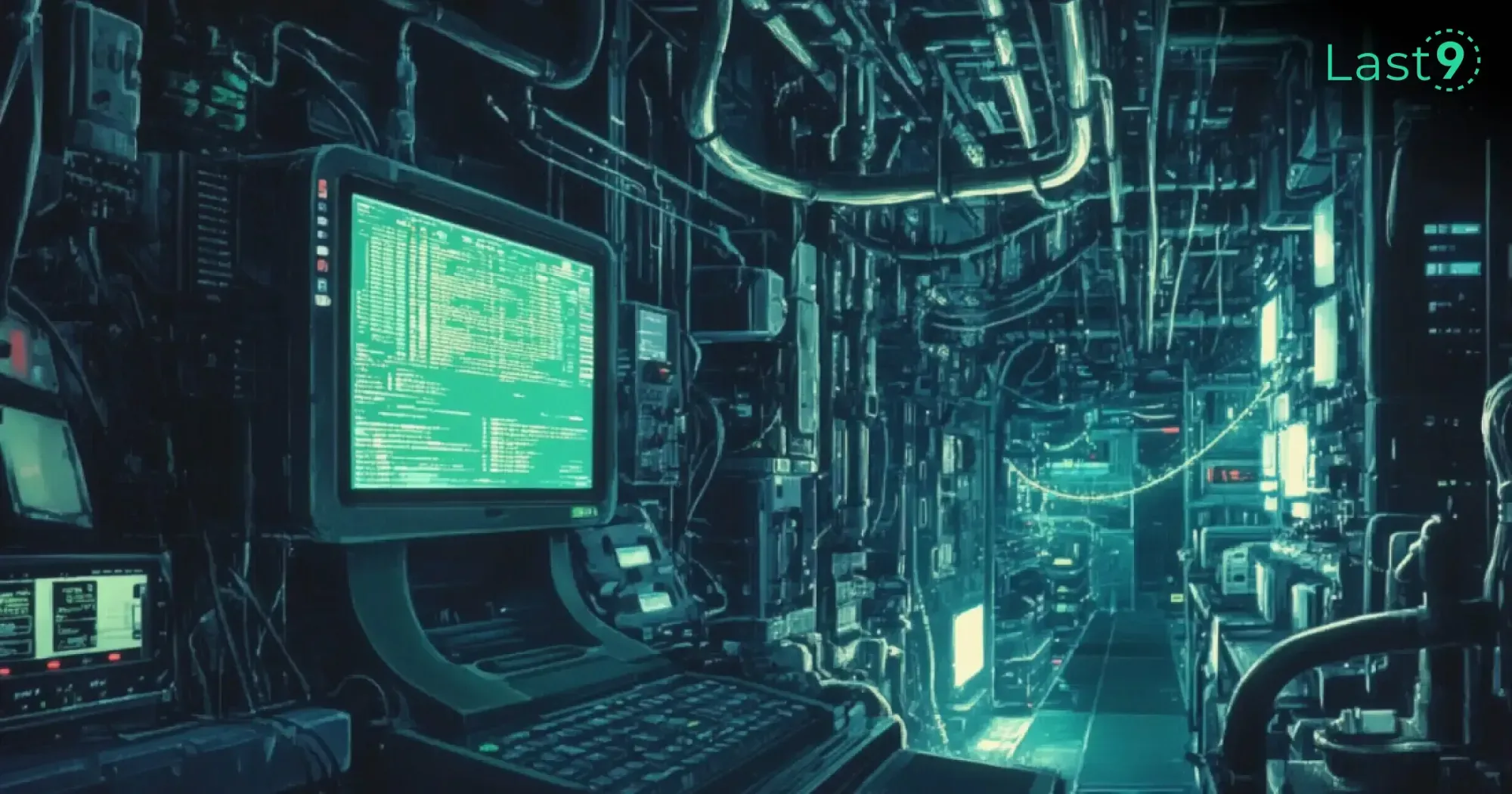
Integrating OpenTelemetry with Elixir: A Step-by-Step Guide
Span Links and Status
Now that you know spans capture detailed information about operations, but they often have complex relationships and outcomes. Span Links and Span Status are key concepts that help represent these intricacies effectively.
1. Span Links: Representing Relationships Between Spans
A span link creates an explicit relationship between two spans that aren’t in a direct parent-child hierarchy. Links are helpful when operations span multiple traces or when you need to correlate spans across systems.
When to Use Span Links
- Fan-out operations: When a single request triggers multiple downstream services.
- Batch processing: When processing a batch of items, each with its own trace.
- Async messaging: When messages are received from a message queue and need to relate the span to the trace that enqueued the message.
Example: Using Span Links
In Python, you can create a span link using Link
objects and include them while starting a new span:
from opentelemetry import trace
from opentelemetry.trace import Link, SpanContext
from opentelemetry.sdk.trace import Resource, TracerProvider
tracer = trace.get_tracer(__name__)
# Simulate a source trace and span context
source_span_context = SpanContext(trace_id=0x1, span_id=0x2, is_remote=True)
# Start a span with a link to the source span
with tracer.start_as_current_span(
"process_batch_item", links=[Link(context=source_span_context)]
) as span:
span.set_attribute("item_id", "12345")
print("Processing batch item")
This example shows how a new span references the source trace through a link, even if the source and current spans are in separate traces.
2. Span Status: Indicating the Outcome of an Operation
The span status reflects whether the operation it represents succeeded or failed. This is crucial for quickly identifying issues within traces.
Status Codes in OpenTelemetry
- UNSET: Default status if no explicit status is set.
- OK: The operation was successful.
- ERROR: The operation failed.
How to Set Span Status
You can set the span status based on the outcome of the operation:
from opentelemetry.trace.status import Status, StatusCode
with tracer.start_as_current_span("http_request") as span:
try:
# Simulate an HTTP request
response_code = 404 # Example response
if response_code == 200:
span.set_status(Status(StatusCode.OK))
else:
span.set_status(Status(StatusCode.ERROR, description="HTTP 404 Not Found"))
except Exception as e:
span.set_status(Status(StatusCode.ERROR, description=str(e)))
When to Use Span Status
- OK: For successful operations (e.g., HTTP 200 responses).
- ERROR: For failures, exceptions, or timeouts (include a description for context).
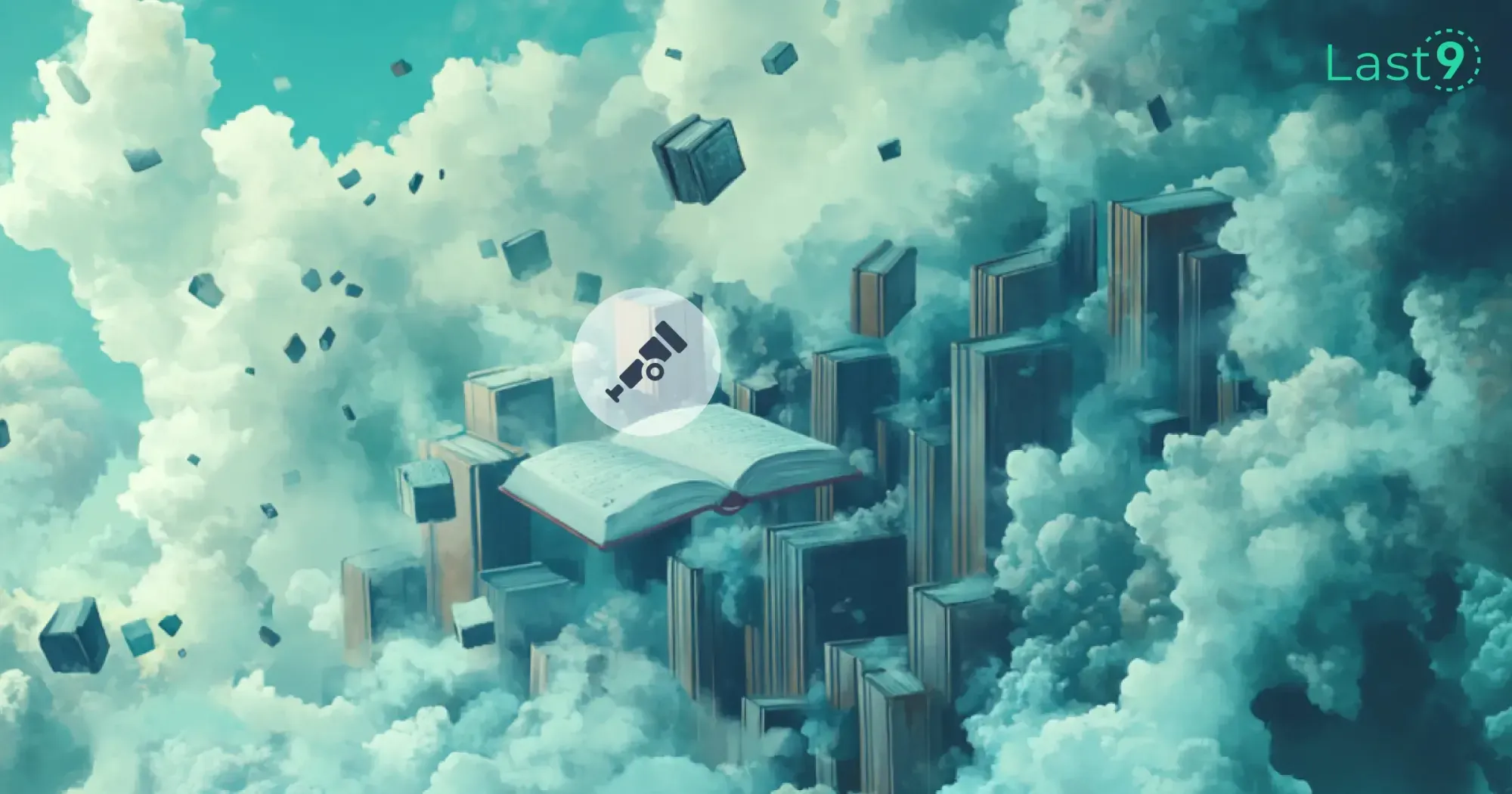
The Role of OpenTelemetry Events in Improving Observability
Span Links vs. Parent-Child Relationships
Feature | Parent-Child | Span Links |
---|---|---|
Definition | Direct hierarchical relationship. | Non-hierarchical relationship. |
Use Case | Request flows and dependencies. | Correlations across traces or systems. |
Example | A function calling another. | A batch item tied to the original batch. |
Best Practices
- Use Links Sparingly: Avoid overusing span links, as they can clutter traces. Use them only for significant relationships.
- Add Context with Attributes: Complement links with attributes to make relationships clearer.
- Set Span Status Early: Set status codes as soon as the operation's outcome is determined.
How Can Attributes and Events Enrich Your Traces?
Once your tracing is up and running, how do you make it truly insightful? By adding attributes and events to your spans!
These elements bring extra context to your traces, making them easier to analyze and more helpful for debugging.
Once you’ve set up tracing, you can add extra context to spans using attributes and events. These elements enrich your traces, making them more insightful and easier to analyze.
Attributes: Adding Context to Spans
Attributes are key-value pairs that provide additional metadata about what the span is doing. They’re like sticky notes of context, helping you debug and analyze traces more effectively.
Common Use Cases for Attributes:
- User or request-specific details (e.g.,
user_id
,http.status_code
). - Environment information (e.g.,
host.name
,cloud.region
).
Example: Adding Attributes to a Span
from opentelemetry import trace
tracer = trace.get_tracer(__name__)
with tracer.start_as_current_span("process_payment") as span:
span.set_attribute("order_id", "12345")
span.set_attribute("payment_method", "credit_card")
span.set_attribute("user_id", "user_67890")
print("Processing payment...")
When to Use Attributes
- Use attributes for static or relatively unchanging metadata directly tied to the operation the span represents.
- They’re particularly helpful for filtering, searching, and understanding the trace context in your visualization tools.
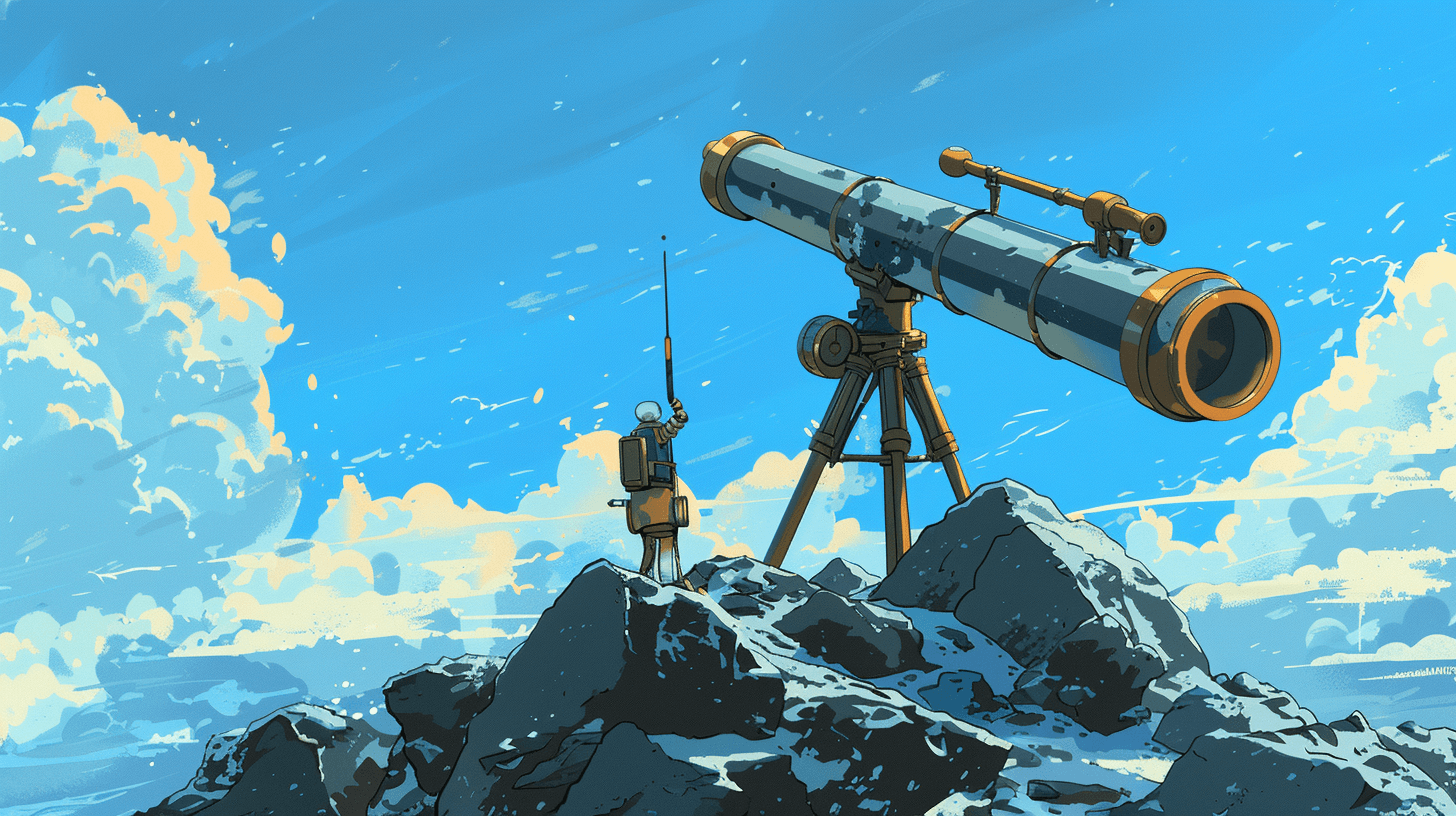
OpenTelemetry Context Propagation for Better Tracing
Events: Capturing Significant Occurrences
Events are discrete, timestamped moments that occur during a span’s lifetime. Think of them as lightweight logs for significant milestones or exceptions within an operation.
Common Use Cases for Events:
- Logging retries for failed requests.
- Capturing state changes (e.g.,
order_dispatched
). - Recording exceptions or errors.
Example: Adding Events to a Span
from opentelemetry import trace
tracer = trace.get_tracer(__name__)
with tracer.start_as_current_span("process_payment") as span:
span.add_event("payment_initiated", attributes={"timestamp": "2024-12-26T12:00:00Z"})
try:
# Simulate a payment failure
raise ValueError("Payment declined")
except ValueError as e:
span.add_event("payment_failed", attributes={"error": str(e)})
print("Payment process completed.")
When to Use Events
- Use events for dynamic, timestamped occurrences during a span's lifetime.
- They’re ideal for tracking progress, milestones, or exceptions within a span.
Attributes vs. Events: What’s the Difference?
Feature | Attributes | Events |
---|---|---|
Purpose | Static metadata about the span. | Dynamic, timestamped occurrences. |
Frequency | Set once or updated sparingly. | Multiple occurrences in a span. |
Use Case | Context and filtering. | Logging significant moments. |
What is Context Propagation in Distributed Tracing?
In distributed tracing, context refers to the metadata that identifies a trace and its current span. This typically includes:
- Trace ID: A unique identifier for the entire trace.
- Span ID: A unique identifier for the current span.
- Sampling Decision: Indicates whether this trace should be sampled or not.
Context propagation is the process of passing this information along with requests so that downstream services can link their spans to the same trace.
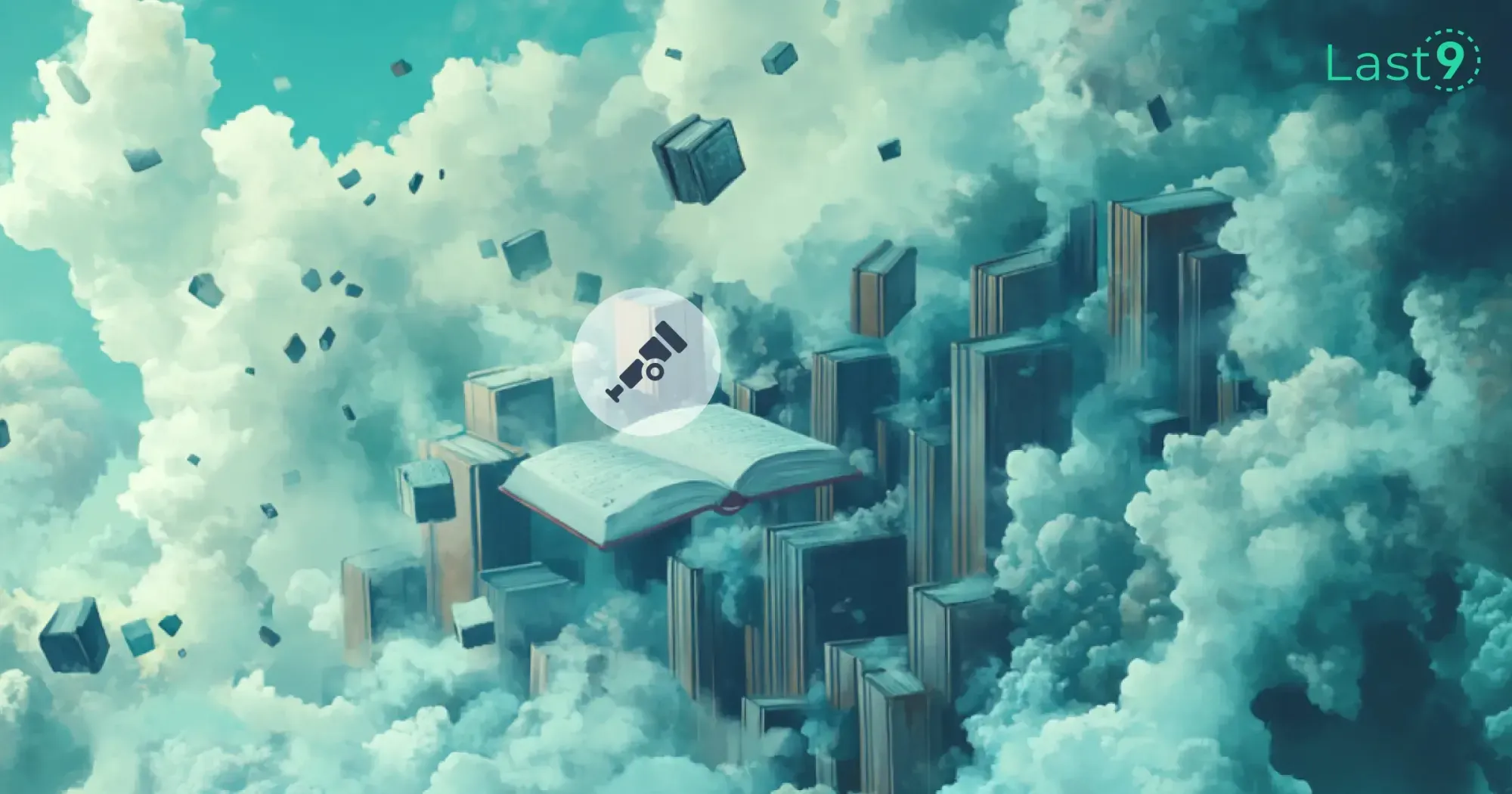
gRPC with OpenTelemetry: Observability Guide for Microservices
How Context Propagation Works
When a request moves from one service to another, the trace context is carried along, ensuring continuity.
- Injecting Context:
Before sending a request, the upstream service (e.g., Service A) injects the trace context into the request headers. - Extracting Context:
Upon receiving the request, the downstream service (e.g., Service B) extracts the trace context from the headers and starts a new span as a child of the received context. - Propagating Context:
The process repeats as requests flow through additional services, databases, or APIs.
Example: Context Propagation in OpenTelemetry
1. Service A: Sending a Request
from opentelemetry import trace
from opentelemetry.propagate import inject
import requests
tracer = trace.get_tracer(__name__)
with tracer.start_as_current_span("service_a_span") as span:
headers = {}
inject(headers) # Inject trace context into headers
response = requests.get("http://service-b.example.com/api", headers=headers)
2. Service B: Receiving a Request
from opentelemetry import trace
from opentelemetry.propagate import extract
from flask import Flask, request
app = Flask(__name__)
tracer = trace.get_tracer(__name__)
@app.route("/api")
def service_b_endpoint():
context = extract(request.headers) # Extract trace context from headers
with tracer.start_as_current_span("service_b_span", context=context) as span:
span.set_attribute("received_from", "service_a")
return "Processed by Service B"
Mechanisms for Context Propagation
OpenTelemetry supports several propagation formats and standards to ensure compatibility across tools and languages:
- W3C Trace Context (default):
A standardized format for trace context propagation. It uses headers liketraceparent
andtracestate
.Exampletraceparent
header:
00-4bf92f3577b34da6a3ce929d0e0e4736-00f067aa0ba902b7-01
- B3 Propagation:
Popular in systems using Zipkin, with headers likeX-B3-TraceId
andX-B3-SpanId
. - Custom Formats:
Some systems may use proprietary propagation mechanisms, which can be integrated using OpenTelemetry's flexible APIs.
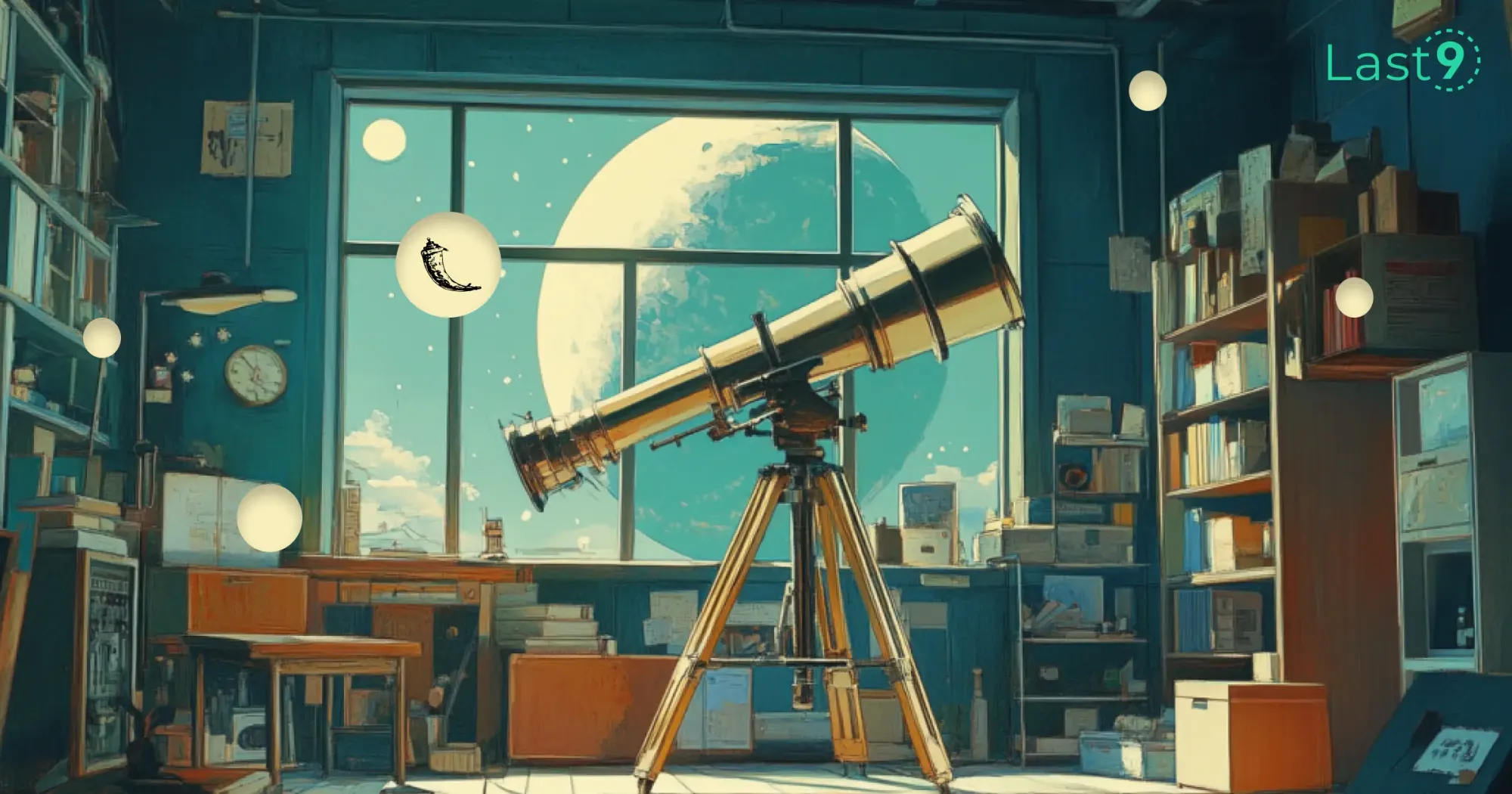
OpenTelemetry with Flask: A Comprehensive Guide for Web Apps
Challenges in Context Propagation
Context propagation, while essential for tracing in distributed systems, comes with its own set of challenges.
Here are some of the most common hurdles teams face:
1. Cross-Language Compatibility
In polyglot environments, where different services are written in different languages, maintaining consistent context propagation can be tricky.
OpenTelemetry’s SDKs help address this by supporting multiple languages out of the box, ensuring that traces remain coherent across services, regardless of language.
2. Third-Party Services
When context needs to flow through external APIs or third-party services, things can get complicated. If the external service doesn't explicitly support context propagation, it can break trace continuity, making it harder to correlate requests across systems.
3. Asynchronous Flows
In asynchronous operations (e.g., message queues), maintaining context becomes more difficult. Without proper handling, the trace context can be lost between the time a request is initiated and when it’s processed by a different service.
Additional setup is often required to ensure that the trace context is carried forward through these operations.
Step-by-Step: Implementing Distributed Tracing with OpenTelemetry
1. Install OpenTelemetry Libraries
To get started, install the necessary OpenTelemetry libraries for your language. For example, in Python:
pip install opentelemetry-api opentelemetry-sdk opentelemetry-exporter-otlp opentelemetry-instrumentation-requests
2. Configure OpenTelemetry SDK
Set up basic tracing configuration. Here’s how you can do it in Python:
from opentelemetry import trace
from opentelemetry.sdk.trace import TracerProvider
from opentelemetry.sdk.trace.export import BatchSpanProcessor
from opentelemetry.exporter.otlp.proto.grpc.trace_exporter import OTLPSpanExporter
# Set up the tracer provider
trace.set_tracer_provider(TracerProvider())
# Configure the OTLP exporter
otlp_exporter = OTLPSpanExporter(endpoint="http://localhost:4317")
# Add a batch span processor to send spans to the exporter
span_processor = BatchSpanProcessor(otlp_exporter)
trace.get_tracer_provider().add_span_processor(span_processor)
# Get the tracer
tracer = trace.get_tracer(__name__)
This will initialize OpenTelemetry to export spans to an OTLP-compatible backend, such as Jaeger or Tempo.
3. Add Instrumentation
You can add automatic instrumentation for libraries like HTTP requests or database queries. For instance:
opentelemetry-instrument your_python_app.py
For manual instrumentation, create spans programmatically:
with tracer.start_as_current_span("fetch_user_data") as span:
# Simulate some work
span.set_attribute("user_id", 123)
print("Fetching user data...")
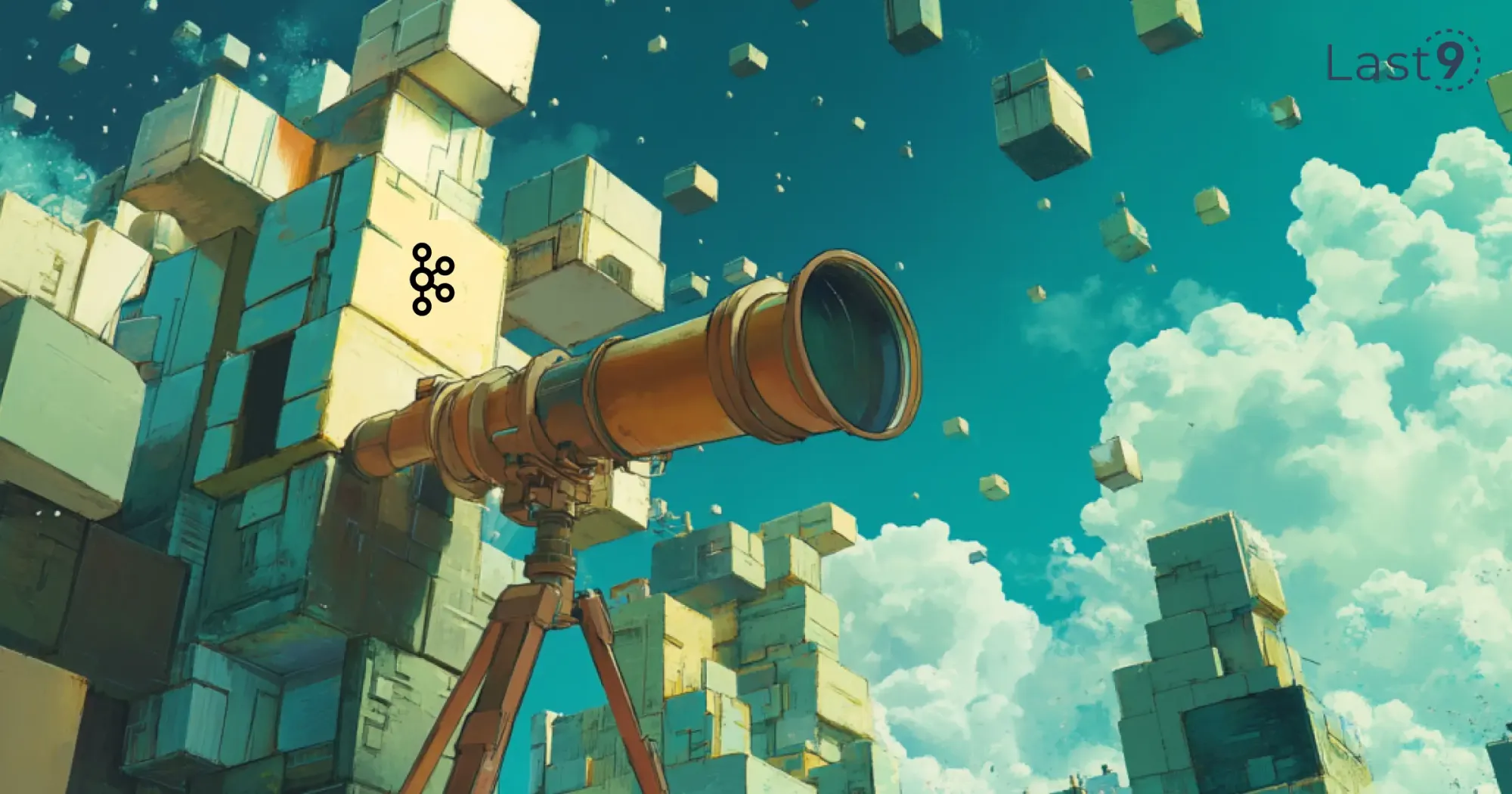
Kafka with OpenTelemetry: Distributed Tracing Guide
4. Export Data
Ensure your trace data is exported to a backend for analysis. Here’s an example configuration for Jaeger:
docker run -d --name jaeger \
-e COLLECTOR_ZIPKIN_HTTP_PORT=9411 \
-p 5775:5775/udp -p 6831:6831/udp -p 6832:6832/udp \
-p 5778:5778 -p 16686:16686 -p 14268:14268 -p 14250:14250 \
-p 9411:9411 jaegertracing/all-in-one:1.39
Update your OTLPSpanExporter endpoint to point to Jaeger:
otlp_exporter = OTLPSpanExporter(endpoint="http://localhost:14250")
Best Practices for Distributed Tracing
1. Use Clear Span Names
Choose clear and descriptive names for your spans:
with tracer.start_as_current_span("process_order") as span:
span.set_attribute("order_id", "12345")
print("Processing order...")
2. Optimize Sampling
Use OpenTelemetry’s ParentBasedTraceIdRatioBased
sampler to control sampling rates and reduce overhead:
from opentelemetry.sdk.trace.sampling import ParentBased, TraceIdRatioBased
trace.set_tracer_provider(
TracerProvider(sampler=ParentBased(TraceIdRatioBased(0.1)))
)
3. Secure Sensitive Data
Always mask or avoid capturing sensitive data, like personally identifiable information (PII), in your spans:
span.set_attribute("user_email", "redacted@example.com")
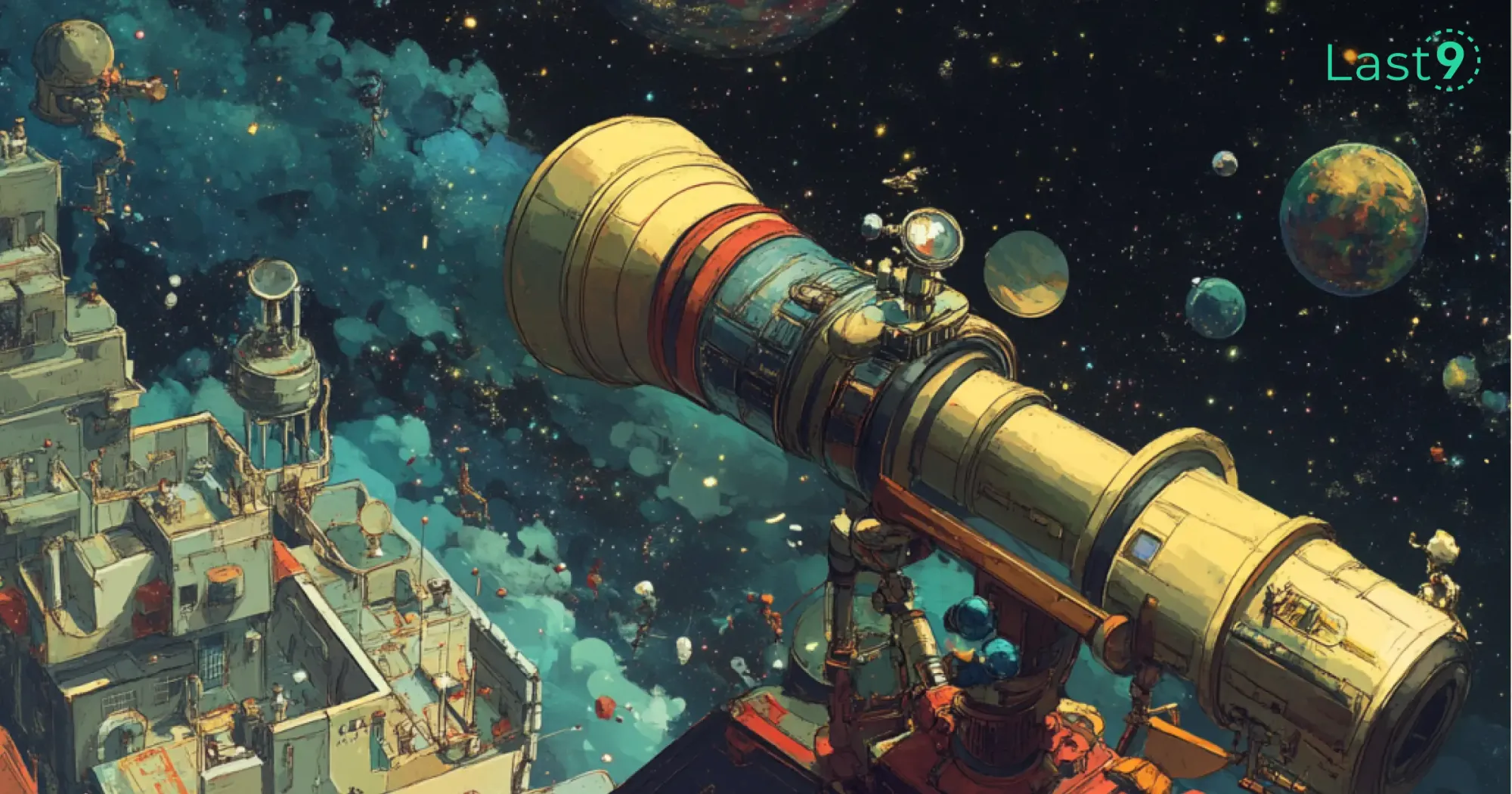
Instrumenting AWS Lambda Functions with OpenTelemetry
How Do You Prioritize Components for Distributed Tracing Instrumentation?
When setting up distributed tracing with OpenTelemetry, the goal is to focus on critical components that impact performance, user experience, or system reliability.
Here's how to identify and prioritize key components for instrumentation:
1. User-Centric Services
Start with services that directly affect the user experience. For example, in an e-commerce platform, services like checkout, payment processing, and inventory management should be prioritized.
2. High-Traffic Services
Look for services that handle a large volume of requests. These services are often bottlenecks and should be instrumented to spot slowdowns early.
3. Inter-Service Communication
Instrument communication layers like APIs or message queues to track how data moves across services. Issues in these layers can propagate across the system.
4. Critical Business Logic
Any service involved in key business processes (e.g., recommendation engines or fraud detection) should be considered for instrumentation. Monitoring these will help optimize business-critical services.
5. External Dependencies
Instrument third-party services, databases, and external APIs that could affect performance, such as payment gateways or shipping providers. This helps identify points of failure or latency.
6. Error-Prone Components
Prioritize services with historical error-prone components, such as complex workflows, legacy systems, or poor test coverage. Instrumenting these services helps identify issues early.
7. Resource-Intensive Services
Services that are resource-heavy (e.g., memory, CPU, or database-intensive) should be instrumented to spot potential resource constraints and allow for optimization.
8. User Journeys
For full transaction flows like placing an order or signing up, instrument these end-to-end user journeys to track system performance from the user's perspective.
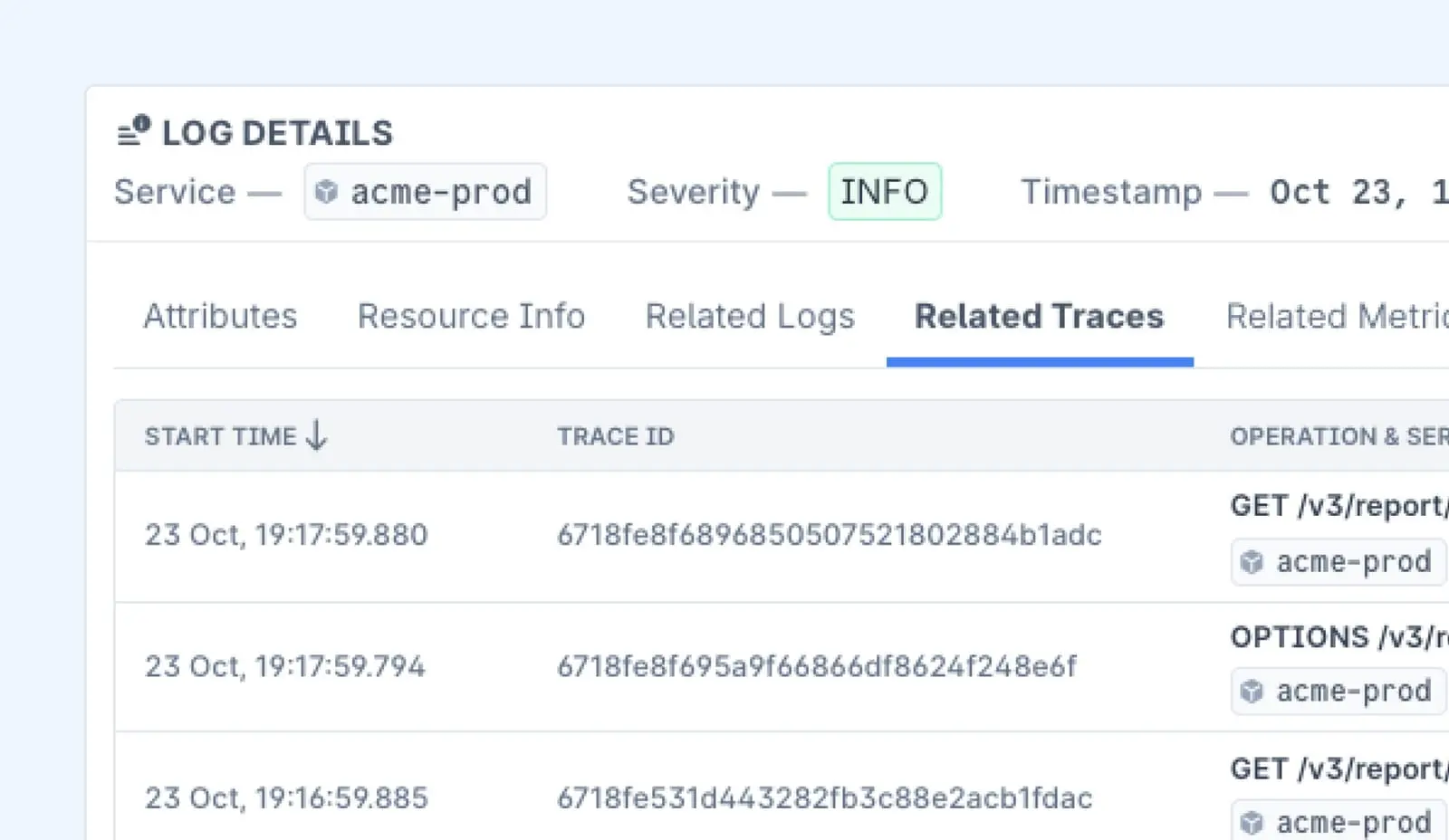
Conclusion
Distributed tracing with OpenTelemetry is a powerful approach to gaining deep insights into the performance and behavior of modern, distributed systems.
OpenTelemetry's flexibility in supporting different languages and propagation formats makes it a versatile solution, while its ability to integrate with a variety of backends ensures you can tailor it to your infrastructure needs.
If you're looking for a managed observability solution that works seamlessly with OpenTelemetry, Last9 is the perfect choice. It brings together metrics, logs, and traces in one unified view, making it easier to identify issues, improve alert management, and simplify troubleshooting.
Schedule a demo today or try it for free!
FAQs
What is OpenTelemetry distributed tracing?
OpenTelemetry distributed tracing is a method of tracking and visualizing the journey of a request as it moves through various services in a distributed system. It provides insights into how different components interact and helps identify performance bottlenecks or errors.
Why is distributed tracing important?
Distributed tracing is crucial for understanding the flow of requests in microservices architectures. It helps identify where delays, failures, or errors occur, ensuring quicker root cause analysis, smoother user experiences, and better overall system performance.
How does OpenTelemetry differ from other tracing solutions?
OpenTelemetry is an open-source, vendor-neutral framework that supports a variety of tracing backends. Unlike proprietary solutions, it allows users to collect, process, and export traces to different observability platforms, providing flexibility and freedom of choice.
What are traces and spans in OpenTelemetry?
- Trace: A trace represents the entire journey of a request across multiple services in your system.
- Span: A span is a single unit of work that captures an operation’s start and end. Multiple spans are grouped together to form a trace.
What are attributes and events in distributed tracing?
- Attributes: Attributes are key-value pairs attached to spans to add context, such as request parameters, user IDs, or the type of operation.
- Events: Events are time-stamped records within a span, providing additional details about specific actions or changes in the system during that span's lifetime.
How do I implement OpenTelemetry distributed tracing in my microservices?
To implement distributed tracing with OpenTelemetry:
- Install OpenTelemetry SDK in your services.
- Configure the tracer to capture spans for key operations.
- Export the traces to a backend (e.g., Jaeger, Prometheus, or Zipkin).
- Use attributes and events to enhance trace visibility.
Can OpenTelemetry work with my existing observability tools?
Yes, OpenTelemetry is designed to integrate with a wide range of observability tools, including Last9, Jaeger, Prometheus, Zipkin, and others. It acts as a bridge, allowing you to use your preferred backend for trace visualization and analysis.
How does distributed tracing help with performance optimization?
Distributed tracing helps identify performance bottlenecks by visualizing how long different operations take. This enables you to spot slow services, optimize database queries, and improve the overall performance of your system.
What is the difference between a trace and a log?
- Trace: Shows the path of a request across multiple services and operations.
- Log: A record of discrete events that may not show the full flow of a request. Logs provide detailed insights at the point of occurrence but don’t typically offer a holistic view of a request's journey like traces do.
Can distributed tracing help with debugging errors?
Yes, by providing detailed visibility into how a request progresses through various services, distributed tracing helps pinpoint where errors occur. You can see which service or operation caused a failure, speeding up the debugging process.
Is distributed tracing resource-intensive?
While distributed tracing adds some overhead, the performance impact is generally minimal when set up correctly. You can adjust the sampling rate to control how much data is captured, balancing performance with visibility.
Can I trace requests across different languages with OpenTelemetry?
Yes, OpenTelemetry supports multiple programming languages, including Java, Python, Go, JavaScript, and more. This makes it possible to trace requests across a heterogeneous environment with services written in different languages.
How can I visualize the traces?
To visualize traces, you’ll need a backend system like Jaeger, Zipkin, or Prometheus. These platforms offer user interfaces to search, filter, and analyze your traces, helping you identify issues and optimize performance.
How do I handle trace data retention?
Trace data can be retained based on the configuration of your tracing backend. Most systems allow you to set retention policies that determine how long trace data is stored. OpenTelemetry lets you control how data is exported, but the retention policy depends on your backend solution.